Implementing Real-Time Data Sync with Node.js and Firebase
Real-time data synchronization is essential in modern applications where data consistency across multiple clients is crucial. Firebase, a backend-as-a-service platform, offers robust real-time capabilities that can be seamlessly integrated with Node.js to build dynamic and responsive applications. This article explores how to implement real-time data synchronization using Node.js and Firebase, complete with practical examples.
Understanding Real-Time Data Synchronization
Real-time data synchronization ensures that all clients connected to an application have access to the most up-to-date data. This feature is particularly important in collaborative applications, live chats, and real-time dashboards. Firebase simplifies this process by providing real-time database capabilities that automatically sync data across all clients.
Setting Up Firebase with Node.js
To get started with Firebase and Node.js, you’ll need to set up your Firebase project and install the necessary Node.js packages.
```bash npm install firebase ``` ```javascript const firebase = require('firebase/app'); require('firebase/database'); // Your web app's Firebase configuration const firebaseConfig = { apiKey: "your-api-key", authDomain: "your-app-id.firebaseapp.com", databaseURL: "https://your-database-name.firebaseio.com", projectId: "your-project-id", storageBucket: "your-storage-bucket", messagingSenderId: "your-messaging-sender-id", appId: "your-app-id" }; // Initialize Firebase firebase.initializeApp(firebaseConfig); const db = firebase.database(); ```
1. Real-Time Data Synchronization
Firebase’s real-time database allows you to sync data in real-time. When data changes in the database, all connected clients receive updates instantly.
Example: Real-Time Data Sync between Multiple Clients
```javascript // Reference to the database location const messagesRef = db.ref('messages'); // Listening for new messages messagesRef.on('child_added', (snapshot) => { console.log('New message:', snapshot.val()); }); // Adding a new message messagesRef.push({ user: 'John Doe', text: 'Hello, World!', timestamp: Date.now() }); ```
In this example, whenever a new message is added to the “messages” node, all connected clients receive the update in real-time.
2. Handling Concurrent Data Updates
Concurrency issues can arise when multiple clients try to update the same data simultaneously. Firebase provides mechanisms to handle these conflicts, ensuring data consistency.
Example: Handling Concurrent Updates with Transactions
```javascript // Reference to the counter node const counterRef = db.ref('counter'); // Using a transaction to handle concurrent updates counterRef.transaction((currentValue) => { return (currentValue || 0) + 1; }, (error, committed, snapshot) => { if (error) { console.error('Transaction failed:', error); } else if (!committed) { console.log('Transaction not committed'); } else { console.log('Counter updated to:', snapshot.val()); } }); ```
In this example, the counter is updated in a way that handles potential conflicts from concurrent updates by multiple clients.
3. Real-Time Listeners and Event Handling
Firebase allows you to set up listeners for different events such as `child_added`, `child_changed`, and `child_removed`, enabling your application to respond to data changes dynamically.
Example: Listening to Data Changes
```javascript // Reference to the tasks node const tasksRef = db.ref('tasks'); // Listening for changes in tasks tasksRef.on('child_changed', (snapshot) => { console.log('Task updated:', snapshot.val()); }); // Listening for task removal tasksRef.on('child_removed', (snapshot) => { console.log('Task removed:', snapshot.val()); }); ```
These listeners allow your application to react to changes in the database in real-time, providing a dynamic user experience.
4. Integrating Firebase Authentication
Firebase Authentication can be seamlessly integrated with your Node.js application to manage user identities and secure data access.
Example: Implementing Firebase Authentication
```javascript const firebaseAuth = firebase.auth(); // Sign in a user firebaseAuth.signInWithEmailAndPassword('user@example.com', 'password123') .then((userCredential) => { console.log('Signed in:', userCredential.user); }) .catch((error) => { console.error('Authentication failed:', error); }); // Listen to authentication state changes firebaseAuth.onAuthStateChanged((user) => { if (user) { console.log('User is signed in:', user); } else { console.log('User is signed out'); } }); ```
With Firebase Authentication, you can secure your real-time data sync operations and ensure that only authenticated users can access and modify data.
Conclusion
Implementing real-time data synchronization with Node.js and Firebase allows you to build responsive and dynamic applications that offer seamless user experiences. From setting up real-time listeners to handling concurrent data updates and integrating authentication, Firebase provides all the tools you need to keep your data in sync across multiple clients.
Further Reading:
Table of Contents
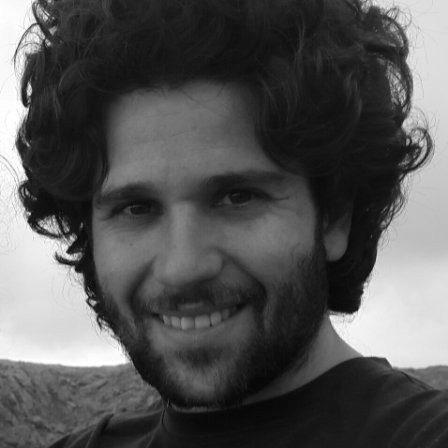
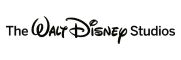