How do you handle database migrations in Node.js?
Database migrations in Node.js involve managing changes to database schemas, structures, and data over time to maintain consistency across different environments and versions of an application. Handling database migrations effectively requires implementing migration scripts, version control, rollback mechanisms, and automated migration workflows. Here’s how you can handle database migrations in Node.js:
- Choose a Migration Tool:
-
-
- Select a database migration tool or framework compatible with Node.js, such as Knex.js, Sequelize, TypeORM, or db-migrate. Evaluate tools based on features, compatibility with database systems, ease of use, and community support.
-
- Initialize Migration Environment:
-
-
- Initialize a migration environment by configuring migration settings, connecting to the database, and setting up migration directories and files. Use CLI commands or configuration files to initialize migration environments and define migration directories.
-
- Create Migration Scripts:
-
- Create migration scripts to define changes to the database schema, tables, indexes, constraints, and data. Each migration script should represent a discrete set of changes and include instructions for migrating and rolling back changes.
- Example:
- javascript
- Copy code
exports.up = async function(knex) { await knex.schema.createTable('users', function(table) { table.increments('id'); table.string('username').notNullable(); table.string('email').notNullable().unique(); table.timestamps(true, true); }); }; exports.down = async function(knex) { await knex.schema.dropTable('users'); };
- Apply Migrations:
-
-
- Apply migration scripts to the database using migration CLI commands or programmatic APIs provided by the migration tool. Run migration commands to execute pending migrations and update the database schema and data accordingly.
-
- Rollback Migrations:
-
- Implement rollback mechanisms to revert applied migrations and restore the database to a previous state. Rollback migrations using CLI commands or programmatic APIs provided by the migration tool. Rollback scripts should reverse the changes made by the corresponding migration scripts, ensuring data consistency and integrity.
- Version Control:
-
-
- Version control migration scripts using a version control system like Git to track changes, collaborate with team members, and manage migrations across different branches and environments. Maintain a clear history of migrations and document changes for future reference.
-
- Automated Migration Workflows:
-
-
- Automate migration workflows using CI/CD pipelines, deployment scripts, or task runners (e.g., npm scripts) to streamline the migration process. Integrate migration tasks with build pipelines to automate database schema updates and ensure consistency across deployments.
-
- Database Seeding and Fixture Data:
-
-
- Optionally, include database seeding and fixture data in migration scripts to populate initial data or test datasets in the database. Use seeding scripts to insert predefined records or generate sample data for testing and development purposes.
-
- Migration Testing:
-
-
- Test migration scripts thoroughly in development, staging, and production environments to validate changes, ensure compatibility with different database systems, and prevent data loss or corruption. Use automated tests, integration tests, and manual inspections to verify migration integrity.
-
- Error Handling and Rollback Strategies:
-
-
- Implement error handling mechanisms and rollback strategies to handle migration failures gracefully. Roll back incomplete or failed migrations automatically, log error messages, and notify administrators or developers about migration issues.
-
- Migration Status Tracking:
-
-
- Track migration status and history using migration tracking tables or metadata stored in the database. Maintain records of applied migrations, migration timestamps, and migration statuses to monitor migration progress and identify discrepancies.
-
- Database Backup and Recovery:
-
- Backup the database before applying migrations to mitigate the risk of data loss or corruption. Implement backup and recovery procedures to restore the database to a previous state in case of migration failures or unexpected issues.
By following these best practices, developers can effectively handle database migrations in Node.js applications, ensuring smooth and consistent database schema updates, data migrations, and version control across different environments and releases. Properly managed migrations contribute to application stability, maintainability, and scalability, facilitating the evolution of database schemas and data models over time.
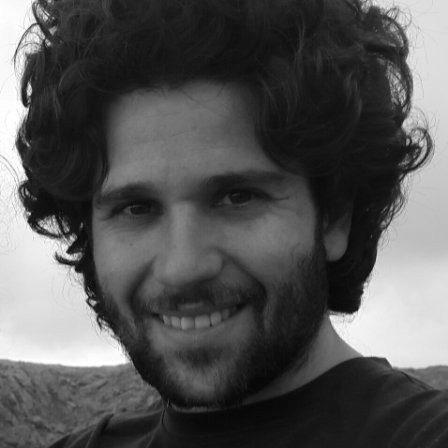
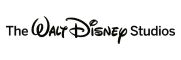