Working with Databases in Node.js: MongoDB, MySQL, and More
Databases are the backbone of modern web applications, allowing us to store, retrieve, and manipulate data efficiently. In the Node.js ecosystem, developers have a plethora of database options to choose from, each catering to different use cases and requirements. In this blog post, we’ll dive into two popular database systems for Node.js: MongoDB and MySQL. We’ll explore the fundamentals of working with databases, including connecting to databases, performing CRUD (Create, Read, Update, Delete) operations, and handling common challenges. So whether you’re building a small-scale application or a large-scale enterprise solution, let’s learn how to harness the power of databases in Node.js.
Table of Contents
1. Understanding Databases and Their Types
1.1. Relational Databases (MySQL)
Relational databases, such as MySQL, organize data into structured tables with predefined schemas. This makes them suitable for applications that require complex queries and maintain strict data consistency. Here’s a code snippet illustrating how to connect to a MySQL database using the popular mysql2 library:
javascript const mysql = require('mysql2'); const connection = mysql.createConnection({ host: 'localhost', user: 'your_username', password: 'your_password', database: 'your_database', }); connection.connect((err) => { if (err) { console.error('Error connecting to MySQL:', err); return; } console.log('Connected to MySQL database'); });
1.2. NoSQL Databases (MongoDB)
NoSQL databases, like MongoDB, offer a more flexible approach to data storage. They store data in collections of documents, often using a JSON-like format. This schema-less nature allows for quick and easy iteration and changes in data structures. Below is an example of connecting to a MongoDB database using the mongodb driver:
javascript const { MongoClient } = require('mongodb'); const uri = 'mongodb://localhost:27017'; const client = new MongoClient(uri); async function connectToMongoDB() { try { await client.connect(); console.log('Connected to MongoDB'); } catch (err) { console.error('Error connecting to MongoDB:', err); } } connectToMongoDB();
2. Setting Up Your Development Environment
Before diving into database operations, you need to set up your Node.js development environment.
2.1. Installing Required Dependencies
For MySQL:
bash npm install mysql2
For MongoDB:
bash npm install mongodb
2.2. Creating a Sample Project Structure
Create a project folder and organize it as follows:
go - my-database-app - node_modules - src - mysql.js - mongodb.js - package.json - package-lock.json
3. Connecting to Databases
Connecting to databases is the first step in working with them. Let’s see how to establish connections to both MySQL and MongoDB databases.
3.1. Connecting to MySQL
In the mysql.js file:
javascript const mysql = require('mysql2'); const connection = mysql.createConnection({ host: 'localhost', user: 'your_username', password: 'your_password', database: 'your_database', }); connection.connect((err) => { if (err) { console.error('Error connecting to MySQL:', err); return; } console.log('Connected to MySQL database'); });
3.2. Connecting to MongoDB
In the mongodb.js file:
javascript const { MongoClient } = require('mongodb'); const uri = 'mongodb://localhost:27017'; const client = new MongoClient(uri); async function connectToMongoDB() { try { await client.connect(); console.log('Connected to MongoDB'); } catch (err) { console.error('Error connecting to MongoDB:', err); } } connectToMongoDB();
4. Performing CRUD Operations
CRUD operations—Create, Read, Update, and Delete—are the core of any database interaction. Let’s walk through examples of each operation for both MySQL and MongoDB.
4.1. Creating Records
MySQL:
javascript // In mysql.js // ... const insertSql = 'INSERT INTO users (username, email) VALUES (?, ?)'; const values = ['john_doe', 'john@example.com']; connection.query(insertSql, values, (err, results) => { if (err) { console.error('Error inserting record:', err); return; } console.log('Record inserted:', results); }); // …
MongoDB:
javascript // In mongodb.js // ... const usersCollection = client.db('mydb').collection('users'); const newUser = { username: 'john_doe', email: 'john@example.com' }; const insertResult = await usersCollection.insertOne(newUser); console.log('Record inserted:', insertResult.insertedId); // …
4.2. Reading Records
MySQL:
javascript // In mysql.js // ... const selectSql = 'SELECT * FROM users'; connection.query(selectSql, (err, results) => { if (err) { console.error('Error fetching records:', err); return; } console.log('Fetched records:', results); }); // …
MongoDB:
javascript // In mongodb.js // ... const usersCollection = client.db('mydb').collection('users'); const fetchResult = await usersCollection.find({}).toArray(); console.log('Fetched records:', fetchResult); // …
4.3. Updating Records
MySQL:
javascript // In mysql.js // ... const updateSql = 'UPDATE users SET email = ? WHERE username = ?'; const updateValues = ['new_email@example.com', 'john_doe']; connection.query(updateSql, updateValues, (err, results) => { if (err) { console.error('Error updating record:', err); return; } console.log('Record updated:', results); }); // …
MongoDB:
javascript // In mongodb.js // ... const usersCollection = client.db('mydb').collection('users'); const filter = { username: 'john_doe' }; const update = { $set: { email: 'new_email@example.com' } }; const updateResult = await usersCollection.updateOne(filter, update); console.log('Record updated:', updateResult.modifiedCount); // …
4.4. Deleting Records
MySQL:
javascript // In mysql.js // ... const deleteSql = 'DELETE FROM users WHERE username = ?'; const deleteValue = ['john_doe']; connection.query(deleteSql, deleteValue, (err, results) => { if (err) { console.error('Error deleting record:', err); return; } console.log('Record deleted:', results); }); // …
MongoDB:
javascript // In mongodb.js // ... const usersCollection = client.db('mydb').collection('users'); const deleteFilter = { username: 'john_doe' }; const deleteResult = await usersCollection.deleteOne(deleteFilter); console.log('Record deleted:', deleteResult.deletedCount); // …
5. Advanced Database Operations
5.1. Indexing and Query Optimization
Both MySQL and MongoDB support indexing, which improves query performance. Ensure you create indexes on frequently queried fields for faster data retrieval.
5.2. Data Validation and Transactions
MySQL and MongoDB offer data validation and transactions to maintain data integrity. For example, MongoDB’s transactions allow you to perform multiple operations as a single unit, ensuring consistency across documents.
6. Handling Database Security
6.1. Authentication and Authorization
Always secure your databases with strong authentication credentials. MySQL and MongoDB provide authentication mechanisms to control access.
6.2. Implementing User Roles
Both database systems allow you to define roles with specific privileges. Assign roles to users based on their responsibilities and access needs.
7. Challenges and Best Practices
7.1. Managing Database Schema Changes
When using relational databases like MySQL, altering the schema can be complex. Plan ahead and use migration tools to handle schema changes smoothly.
7.2. Scaling Databases for High Traffic
As your application grows, scaling becomes crucial. Consider using sharding (MongoDB) or clustering (MySQL) techniques to distribute data across multiple nodes.
Conclusion
Working with databases in Node.js opens the door to endless possibilities in data-driven applications. By understanding the differences between MySQL and MongoDB, setting up your development environment, and mastering CRUD operations, you’re well on your way to building robust and scalable database-driven applications. Whether you choose a relational or NoSQL database, remember to follow best practices for security, performance, and data integrity. So go ahead and experiment with these databases, and watch your Node.js applications flourish with efficient and reliable data storage.
Table of Contents
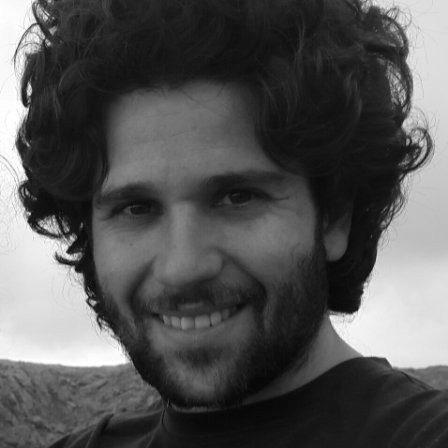
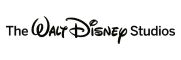