Deploying Node.js Applications: Best Practices and Options
In today’s fast-paced digital landscape, deploying web applications quickly and efficiently is crucial for staying competitive. Node.js, a popular runtime environment for building scalable and high-performance applications, has gained widespread adoption in the development community. However, deploying Node.js applications requires careful consideration of various factors to ensure a seamless and reliable deployment process.
Table of Contents
1. Understanding the Deployment Process
Deploying a Node.js application involves a series of steps to make your application accessible to users over the internet. This process includes setting up servers, configuring environments, managing dependencies, and automating deployment pipelines. Let’s delve into the best practices and deployment options that can help you streamline this process.
2. Best Practices for Deploying Node.js Applications
2.1. Version Control and Continuous Integration (CI):
Before you start deploying your application, ensure that you are using a version control system like Git to track your code changes. Integrating a CI/CD (Continuous Integration/Continuous Deployment) pipeline into your workflow helps automate the deployment process. Services like Jenkins, Travis CI, and CircleCI can be used to automatically build, test, and deploy your application whenever you push changes to your repository.
yaml # Example .travis.yml configuration for Node.js application language: node_js node_js: - 14 script: - npm install - npm test deploy: provider: heroku app: your-app-name api_key: $HEROKU_API_KEY
2.2. Environment Configuration:
Use environment variables to manage configuration settings that vary between development, staging, and production environments. Tools like dotenv can help you load environment-specific variables from .env files during development. For production, use the environment variables provided by your hosting platform.
javascript // Using dotenv to load environment variables require('dotenv').config(); const port = process.env.PORT || 3000; const dbConnectionString = process.env.DB_CONNECTION_STRING;
2.3. Dependency Management:
Utilize package.json to manage your application’s dependencies. Use a lockfile (e.g., package-lock.json or yarn.lock) to ensure consistent and reproducible installations across environments.
json // Example package.json dependency management { "dependencies": { "express": "^4.17.1", "mongodb": "^4.0.0" }, "devDependencies": { "jest": "^27.0.6", "nodemon": "^2.0.12" } }
3. Deployment Options for Node.js Applications
3.1. Traditional Server Hosting:
You can deploy your Node.js application on traditional server hosting providers like AWS EC2, DigitalOcean, or Linode. This approach gives you more control over the server environment, allowing you to configure security settings, databases, and other services. However, it also requires more maintenance and scaling efforts.
bash # Example of deploying via SSH to a remote server scp -r ./dist myuser@your-server-ip:/var/www/myapp
3.2. Platform as a Service (PaaS):
PaaS providers like Heroku and Google App Engine abstract much of the server management complexity. They offer streamlined deployment processes, automatic scaling, and integrated databases. However, PaaS solutions might limit customization and can be more expensive as your application scales.
bash # Deploying to Heroku git push heroku main
3.3. Containerization and Orchestration:
Docker and Kubernetes provide a powerful solution for packaging applications along with their dependencies and configurations. This enables consistent deployments across different environments and simplifies scaling.
Dockerfile # Example Dockerfile for a Node.js application FROM node:14 WORKDIR /app COPY package*.json ./ RUN npm install COPY . . EXPOSE 3000 CMD ["node", "index.js"] bash Copy code # Deploying a Docker container docker build -t my-node-app . docker run -p 3000:3000 my-node-app
3.4. Serverless Computing:
Serverless platforms like AWS Lambda and Azure Functions allow you to deploy code in response to events without managing servers. This approach is suitable for applications with variable workloads and can significantly reduce operational overhead.
javascript // Example AWS Lambda function handler exports.handler = async (event) => { // Handle the event return { statusCode: 200, body: JSON.stringify('Hello from Lambda!') }; };
4. Automating Deployment with Continuous Deployment Pipelines
Setting up a continuous deployment pipeline is essential to achieve a smooth and efficient deployment process. Here’s a general outline of the steps involved:
- Source Control: Host your code in a version control system like Git.
- Continuous Integration (CI): Use a CI service to build and test your application whenever changes are pushed to the repository.
- Automated Tests: Implement automated tests to ensure that your application functions correctly after each change.
- Deployment Configuration: Define deployment configuration settings, including environment variables and platform-specific settings.
- Deployment Script: Create a script that automates the deployment process, including building, packaging, and deploying your application.
- Deployment Platform: Choose a deployment platform that aligns with your requirements (PaaS, containerization, serverless, etc.).
- Deployment Trigger: Set up a trigger to deploy your application automatically when changes are merged into the main branch.
- Monitoring and Rollback: Implement monitoring and error tracking to detect issues in real-time. Prepare a rollback strategy in case deployment issues arise.
Conclusion
Deploying Node.js applications involves a blend of best practices and strategic choices based on your application’s requirements. Whether you opt for traditional hosting, PaaS solutions, containerization, or serverless architecture, the key lies in maintaining a reliable and efficient deployment pipeline. By following the best practices outlined in this guide and leveraging the deployment options available, you can ensure that your Node.js application reaches your audience seamlessly while maintaining high performance and availability. Remember, a well-executed deployment process is a significant step toward the success of your application in today’s competitive digital landscape.
In summary, Node.js empowers developers to create powerful applications, and deploying them effectively is equally important to deliver exceptional user experiences. Keep refining your deployment strategy, stay updated with the latest tools and techniques, and watch your Node.js application thrive in the online world.
Table of Contents
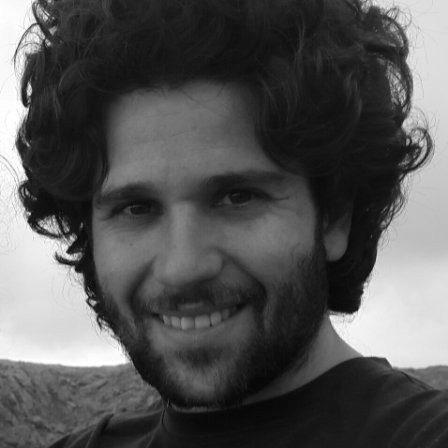
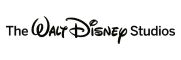