Exploring Design Patterns in Node.js: A Practical Approach
In the realm of Node.js development, mastering design patterns is akin to wielding a powerful arsenal of tools that can elevate your code to new heights of efficiency, scalability, and maintainability. Design patterns are proven solutions to recurring problems, offering structured approaches to common challenges faced by developers. In this article, we’ll delve into some key design patterns in Node.js, accompanied by real-world examples to illustrate their practical applications.
1. Singleton Pattern
The Singleton Pattern ensures that a class has only one instance and provides a global point of access to it. This pattern is useful when exactly one object is needed to coordinate actions across the system. Consider a scenario where you need to establish a database connection pool in your Node.js application. By implementing the Singleton Pattern, you can ensure that there is only one instance of the connection pool throughout the application’s lifecycle.
Example:
class Database { constructor() { if (!Database.instance) { this.connection = this.connectToDatabase(); Database.instance = this; } return Database.instance; } connectToDatabase() { // Database connection logic } } const dbInstance = new Database(); module.exports = dbInstance;
2. Factory Pattern
The Factory Pattern provides an interface for creating objects without specifying their concrete classes. It allows for the creation of objects based on certain conditions or parameters, promoting loose coupling and enhancing code maintainability. In a Node.js application, the Factory Pattern can be employed to create instances of different types of services or components dynamically.
Example:
class PaymentGatewayFactory { createPaymentGateway(type) { switch (type) { case 'credit': return new CreditCardPaymentGateway(); case 'paypal': return new PayPalPaymentGateway(); default: throw new Error('Invalid payment gateway type'); } } } const paymentGatewayFactory = new PaymentGatewayFactory(); const creditGateway = paymentGatewayFactory.createPaymentGateway('credit'); const paypalGateway = paymentGatewayFactory.createPaymentGateway('paypal');
3. Observer Pattern
The Observer Pattern defines a one-to-many dependency between objects, where multiple observers (or subscribers) are notified of any state changes in a subject (or publisher). This pattern is particularly useful in scenarios involving event handling and pub/sub systems. In a Node.js application, the Observer Pattern can facilitate communication between various modules or components.
Example:
class Subject { constructor() { this.observers = []; } addObserver(observer) { this.observers.push(observer); } notifyObservers(data) { this.observers.forEach(observer => observer.update(data)); } } class Observer { update(data) { // Handle updated data } } const subject = new Subject(); const observer1 = new Observer(); const observer2 = new Observer(); subject.addObserver(observer1); subject.addObserver(observer2); subject.notifyObservers('Some data has been updated');
Conclusion
Mastering design patterns in Node.js empowers developers to write more robust, scalable, and maintainable code. By leveraging patterns such as Singleton, Factory, and Observer, developers can tackle common challenges with confidence and efficiency, ultimately leading to better software design and development practices.
For further reading on design patterns in Node.js, check out these additional resources:
- Node.js Design Patterns – GitHub Repository
- Understanding Design Patterns in Node.js – Medium Article
- Node.js Design Patterns – Pluralsight Course
Happy coding!
Table of Contents
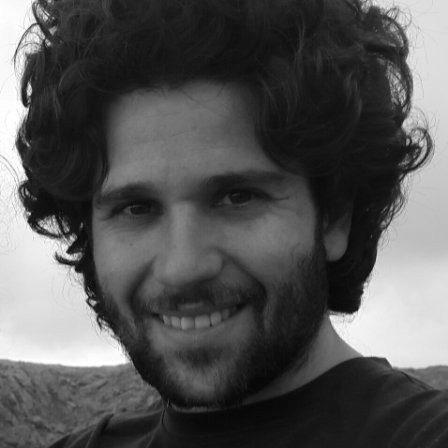
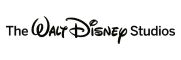