Building Desktop GUI Applications with Node.js and NW.js
Introduction to NW.js
NW.js, formerly known as node-webkit, is an open-source framework that enables developers to create cross-platform desktop applications using web technologies such as HTML, CSS, and JavaScript. NW.js combines the flexibility of web development with the power of Node.js, allowing developers to access native APIs and build feature-rich desktop applications.
Getting Started with NW.js
To kickstart your journey with NW.js, you’ll need to have Node.js installed on your machine. Once Node.js is set up, you can install NW.js using npm, the Node.js package manager, with a simple command:
npm install nw
After installing NW.js, you can create a new project and structure it similar to a web application, with HTML, CSS, and JavaScript files. NW.js provides a familiar development environment for web developers, enabling them to leverage their existing skills and tools.
Building Desktop GUI Applications
Now, let’s dive into the process of building a desktop GUI application with Node.js and NW.js. We’ll outline the essential steps and provide examples to illustrate each concept.
Creating the User Interface (UI)
Start by designing the user interface using HTML and CSS. You can use popular front-end frameworks like Bootstrap or Materialize to streamline the UI development process.
Example:
<!DOCTYPE html> <html> <head> <title>My NW.js App</title> <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/materialize/1.0.0/css/materialize.min.css"> </head> <body> <div class="container"> <h1>Hello NW.js!</h1> </div> </body> </html>
Adding Functionality with JavaScript
Next, enhance your application’s functionality using JavaScript. You can use Node.js modules to perform file I/O operations, interact with databases, or implement other backend functionality.
Example:
const fs = require('fs'); fs.writeFile('example.txt', 'Hello, NW.js!', (err) => { if (err) throw err; console.log('File created successfully!'); });
Accessing Native APIs
NW.js allows you to access native APIs using Node.js modules, enabling seamless integration with the underlying operating system. You can leverage these APIs to access system resources, interact with hardware peripherals, or perform other system-level tasks.
Example:
const { app, BrowserWindow } = require('nw'); let mainWindow; app.on('ready', () => { mainWindow = new BrowserWindow({ width: 800, height: 600 }); mainWindow.loadURL('https://example.com'); });
Packaging and Distribution
Once your desktop application is ready, you can package it for distribution across different platforms. NW.js provides built-in tools for packaging your application as standalone executables for Windows, macOS, and Linux.
Example:
nwjs-builder . -p [platform]
Real-World Examples
Slack
Slack, the popular messaging platform, utilizes NW.js to deliver its desktop application across multiple operating systems. By leveraging NW.js, Slack offers a seamless user experience with access to native features and functionalities.
Visual Studio Code
Microsoft’s Visual Studio Code, a widely-used code editor, is built using NW.js. Visual Studio Code combines the power of a native desktop application with the flexibility of web technologies, providing developers with a feature-rich coding environment.
Atom
Atom, a customizable text editor developed by GitHub, is another example of a desktop application built with NW.js. Atom’s extensible architecture, powered by web technologies, enables developers to personalize their coding experience with various plugins and themes.
Conclusion
Node.js and NW.js offer a compelling solution for building desktop GUI applications with web technologies. By leveraging the power of Node.js and combining it with the flexibility of web development, developers can create cross-platform desktop applications with ease. Whether you’re building productivity tools, communication platforms, or creative applications, Node.js and NW.js provide the tools and resources you need to bring your ideas to life. Happy coding!
External Resources:
Table of Contents
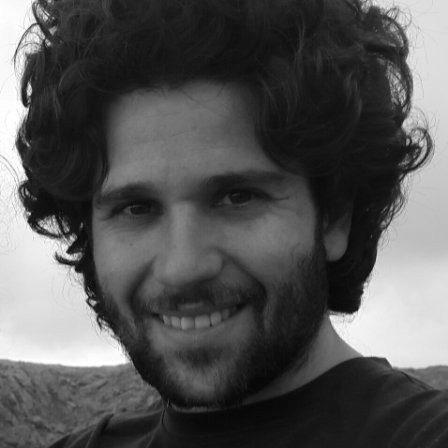
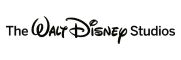