Developing APIs with Node.js and LoopBack
In the ever-evolving landscape of web development, creating robust and efficient APIs (Application Programming Interfaces) is paramount for building scalable and maintainable applications. Node.js, with its asynchronous, event-driven architecture, has become a popular choice for backend development. When combined with LoopBack, a highly extensible Node.js framework, developers can streamline the process of building APIs with minimal effort. In this comprehensive guide, we’ll explore the ins and outs of developing APIs with Node.js and LoopBack, accompanied by examples to illustrate key concepts.
What is LoopBack?
LoopBack is an open-source Node.js framework that enables developers to quickly create RESTful APIs and connect them to various data sources. It offers a powerful set of tools for defining models, handling authentication, and implementing business logic, making it an ideal choice for building both simple prototypes and complex enterprise applications.
Getting Started with LoopBack
To kickstart your journey with LoopBack, you’ll first need to install it via npm, Node.js’s package manager. Once installed, you can scaffold a new LoopBack application using the provided CLI (Command Line Interface). From there, you can define models to represent your data structures, create endpoints to expose CRUD (Create, Read, Update, Delete) operations, and configure data sources to interact with databases or external services.
npm install -g loopback-cli lb app my-api
Creating Models and Data Sources
Models in LoopBack define the structure of your data and provide methods for interacting with it. You can define models using the built-in schema definition language or extend existing models to inherit their properties and behaviors. Additionally, LoopBack supports various data sources out of the box, including relational databases like MySQL and PostgreSQL, NoSQL databases like MongoDB, and RESTful services.
// Example: Defining a Product model const { Model } = require('loopback'); class Product extends Model { static definition() { return { name: 'string', price: 'number', }; } }
Implementing Endpoints and Remote Methods
Once you’ve defined your models and data sources, you can create endpoints to expose your API’s functionality to clients. LoopBack provides decorators and mixins to define RESTful routes for your models automatically. Additionally, you can define remote methods to encapsulate business logic and expose custom endpoints tailored to your application’s needs.
// Example: Exposing CRUD endpoints for the Product model const { CRUDControllerMixin } = require('loopback'); class ProductController extends CRUDControllerMixin { constructor() { super(Product); } }
Securing Your API
Security is a critical aspect of API development, and LoopBack offers built-in support for authentication and authorization mechanisms. You can configure authentication providers like OAuth 2.0, JWT (JSON Web Tokens), or integrate with third-party identity providers such as Auth0. Additionally, LoopBack allows you to define access controls to restrict endpoints based on user roles and permissions.
Scaling and Extending Your API
As your application grows, you may need to scale your API to handle increased traffic and add new features. LoopBack’s modular architecture and extensible design make it easy to scale your application horizontally by deploying multiple instances behind a load balancer. Furthermore, you can extend LoopBack’s functionality by creating custom middleware, connectors, and mixins to integrate with third-party services or implement specialized business logic.
Conclusion
Developing APIs with Node.js and LoopBack empowers developers to create scalable, maintainable, and secure backend systems with ease. By leveraging LoopBack’s powerful features for defining models, creating endpoints, and handling authentication, you can accelerate the development process and focus on delivering value to your users. Whether you’re building a simple prototype or a complex enterprise application, Node.js and LoopBack provide the tools you need to succeed.
External Resources:
Start your journey with Node.js and LoopBack today and unlock the full potential of API development in the modern web ecosystem. Happy coding!
Table of Contents
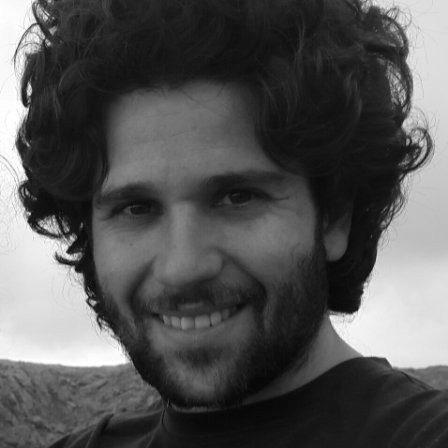
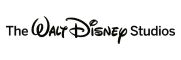