Developing RESTful Microservices with Node.js and Seneca
In today’s fast-paced tech landscape, the demand for scalable and efficient microservices architecture is higher than ever. Enter Node.js and Seneca, a dynamic duo empowering developers to craft robust RESTful microservices with ease. In this guide, we’ll delve into the fundamentals of developing RESTful microservices using Node.js and Seneca, accompanied by real-world examples to illustrate their prowess.
Understanding RESTful Microservices
RESTful microservices, a cornerstone of modern application development, offer a modular approach to building complex systems by breaking down functionalities into small, independent services. These services communicate via HTTP protocols, adhering to REST (Representational State Transfer) principles for seamless interaction and interoperability.
Getting Started with Node.js and Seneca
Node.js, renowned for its event-driven, non-blocking I/O model, serves as an ideal foundation for building microservices due to its lightweight and scalable nature. Coupled with Seneca, a microservices toolkit for Node.js, developers gain access to a plethora of features for service composition, communication, and deployment.
Setting Up Your Environment
Before diving into development, ensure you have Node.js and Seneca installed on your system. Follow the official documentation for installation instructions:
Once installed, initialize a new Node.js project and install Seneca as a dependency using npm:
npm init -y npm install seneca
Building Your First RESTful Microservice
Let’s create a simple microservice to handle user authentication. Start by defining a Seneca service:
// auth-service.js const seneca = require('seneca')(); seneca.add({ role: 'auth', cmd: 'login' }, (msg, respond) => { // Authentication logic const { username, password } = msg; // Authenticate user const isAuthenticated = true; // Dummy logic respond(null, { isAuthenticated }); }); seneca.listen(3000);
This code snippet defines an authentication service that listens on port 3000 and responds to login requests.
Integrating Microservices
Once you’ve created individual microservices, it’s time to integrate them into your application. Seneca offers various communication patterns such as Pub/Sub and Request/Response to facilitate seamless interaction between services.
// main-app.js const seneca = require('seneca')(); // Import microservices const authService = require('./auth-service'); // Integrate auth service seneca.client({ port: 3000, pin: { role: 'auth' } }); seneca.act({ role: 'auth', cmd: 'login', username: 'example', password: 'password' }, (err, response) => { if (err) { console.error(err); } else { console.log('Authentication status:', response.isAuthenticated); } });
In this example, the main application communicates with the authentication microservice to authenticate a user.
Real-World Applications
Node.js and Seneca have been instrumental in powering various real-world applications, including:
- Netflix: Utilizes microservices architecture for seamless content delivery and personalization.
- Walmart: Leverages microservices to enhance scalability and flexibility across its e-commerce platforms.
- Uber: Relies on microservices for managing ride requests, driver allocation, and trip tracking.
Conclusion
Node.js and Seneca empower developers to harness the full potential of microservices architecture, enabling scalable, resilient, and flexible solutions. By adopting these technologies and principles, businesses can stay ahead in today’s competitive landscape and deliver exceptional user experiences.
Embark on your journey of building RESTful microservices with Node.js and Seneca, and unleash the true power of modular architecture.
Happy coding!
Table of Contents
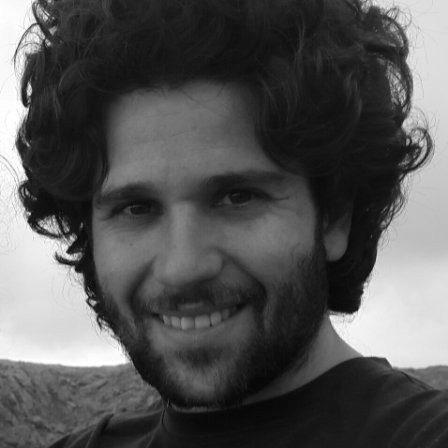
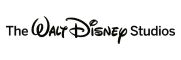