What is the purpose of the DNS module in Node.js?
The DNS (Domain Name System) module in Node.js provides utilities for performing DNS lookups, resolving domain names to IP addresses, and resolving IP addresses to domain names. It allows developers to interact with DNS servers, resolve hostnames, cache DNS responses, and handle DNS-related operations asynchronously.
Key purposes of the DNS module include:
- Hostname Resolution:
-
- Use the dns.resolve() and dns.resolve4() methods to resolve domain names (hostnames) to IP addresses (IPv4 addresses). These methods perform DNS lookups and return IP addresses associated with the specified domain name.
- Example:
- javascript
- Copy code
const dns = require('dns'); dns.resolve4('www.example.com', (err, addresses) => { if (err) throw err; console.log('IP addresses:', addresses); });
- Reverse DNS Lookup:
-
- Perform reverse DNS lookups using the dns.reverse() method to resolve IP addresses to domain names. This method returns an array of domain names associated with the specified IP address.
Example:
javascript
const dns = require('dns'); dns.reverse('8.8.8.8', (err, hostnames) => { if (err) throw err; console.log('Hostnames:', hostnames); });
- Hostname Resolution Cache:
-
-
- Node.js maintains a built-in DNS cache to cache resolved DNS queries for improved performance and reduced DNS lookup latency. The DNS cache caches resolved IP addresses and domain names, reducing the overhead of repeated DNS lookups for frequently accessed resources.
-
- Custom DNS Server Configuration:
-
- Configure custom DNS server settings, timeouts, and resolver options using the dns.setServers() method. This allows developers to specify alternative DNS servers, customize DNS resolution behavior, and override default DNS settings as needed.
- Example:
- javascript
- Copy code
const dns = require('dns'); const customServers = ['8.8.8.8', '8.8.4.4']; dns.setServers(customServers);
- DNS Resolution Events:
-
-
- Node.js emits DNS resolution events such as ‘lookup’, ‘error’, and ‘close’ to notify applications about DNS resolution status, errors, and changes in DNS server configuration. Developers can listen for these events and implement error handling and logging mechanisms accordingly.
-
- DNSSEC (DNS Security Extensions) Support:
-
-
- Node.js supports DNSSEC (DNS Security Extensions) for secure DNS resolution and validation of DNS responses. DNSSEC ensures the authenticity and integrity of DNS data by adding digital signatures to DNS records and validating DNS responses using cryptographic keys.
-
- Asynchronous DNS Operations:
-
-
- All DNS operations in Node.js are asynchronous and non-blocking, allowing applications to perform DNS lookups, reverse lookups, and DNS-related tasks without blocking the event loop or delaying other I/O operations.
-
- DNS Resolution Performance:
-
- Optimize DNS resolution performance by minimizing DNS lookup latency, leveraging DNS caching, and configuring DNS resolver settings based on application requirements. Monitor DNS resolution times and optimize DNS configurations for improved application performance.
The DNS module in Node.js facilitates domain name resolution, IP address resolution, reverse DNS lookup, and DNS caching, providing essential functionalities for network communication, web applications, and server-side development. By leveraging the DNS module, developers can perform DNS-related tasks efficiently, resolve hostnames and IP addresses reliably, and ensure robust network connectivity in Node.js applications.
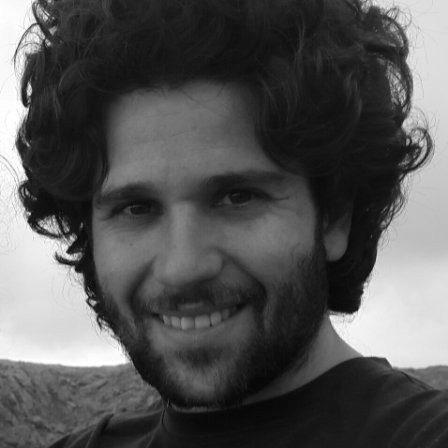
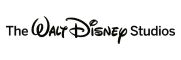