Deploying Node.js Applications with Docker and Kubernetes
Deploying applications effectively is crucial for maintaining smooth operations and scaling efficiently. Docker and Kubernetes are powerful tools that streamline the deployment process, especially for Node.js applications. This guide explores how to use Docker for containerization and Kubernetes for orchestration to manage your Node.js applications.
Understanding Docker and Kubernetes
– Docker is a platform that enables developers to package applications and their dependencies into containers. Containers ensure that your application runs consistently across different environments.
– Kubernetes is an orchestration tool that automates the deployment, scaling, and management of containerized applications. It provides advanced features for load balancing, rolling updates, and self-healing.
Using Docker for Node.js Applications
1. Creating a Dockerfile
The Dockerfile is a script containing instructions on how to build a Docker image for your Node.js application. Here’s an example Dockerfile:
```dockerfile # Use the official Node.js image FROM node:18 # Set the working directory WORKDIR /usr/src/app # Copy package.json and package-lock.json COPY package*.json ./ # Install dependencies RUN npm install # Copy the rest of the application code COPY . . # Expose the application port EXPOSE 3000 # Start the application CMD ["npm", "start"] ```
2. Building and Running the Docker Container
To build and run your Docker container, use the following commands:
```bash # Build the Docker image docker build -t my-node-app . # Run the Docker container docker run -p 3000:3000 my-node-app ```
Using Kubernetes for Orchestration
1. Creating a Kubernetes Deployment
A Kubernetes Deployment manages the deployment and scaling of your containerized application. Here’s a sample deployment configuration:
```yaml apiVersion: apps/v1 kind: Deployment metadata: name: node-app-deployment spec: replicas: 3 selector: matchLabels: app: node-app template: metadata: labels: app: node-app spec: containers: - name: node-app image: my-node-app:latest ports: - containerPort: 3000 ```
2. Creating a Kubernetes Service
A Kubernetes Service exposes your application to the network. Here’s a sample service configuration:
```yaml apiVersion: v1 kind: Service metadata: name: node-app-service spec: type: LoadBalancer selector: app: node-app ports: - protocol: TCP port: 80 targetPort: 3000 ```
3. Deploying to Kubernetes
To deploy your Node.js application to a Kubernetes cluster, use the following commands:
```bash # Apply the deployment configuration kubectl apply -f deployment.yaml # Apply the service configuration kubectl apply -f service.yaml # Check the status of your deployment kubectl get deployments # Check the status of your service kubectl get services ```
Scaling and Updating Your Deployment
1. Scaling the Deployment
You can scale your application to handle more traffic by adjusting the number of replicas:
```bash kubectl scale deployment node-app-deployment --replicas=5 ```
2. Rolling Updates
Kubernetes supports rolling updates, which allows you to update your application without downtime:
```bash # Update the deployment with a new image version kubectl set image deployment/node-app-deployment node-app=my-node-app:v2 ```
Conclusion
Deploying Node.js applications with Docker and Kubernetes simplifies the process of managing and scaling your applications. Docker provides a consistent environment across different stages of development, while Kubernetes offers robust orchestration for deployment, scaling, and management. By leveraging these tools, you can ensure a smoother deployment process and a more resilient application infrastructure.
Further Reading:
Table of Contents
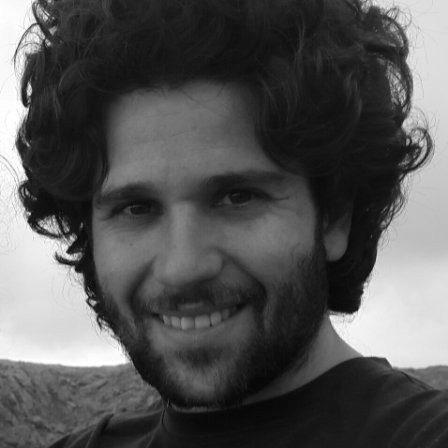
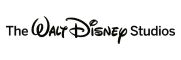