Building Real-Time Analytics Dashboards with Node.js and Elasticsearch
In today’s data-driven world, having the ability to analyze and visualize data in real-time is crucial for making informed decisions. Elasticsearch, combined with Node.js, offers a powerful solution for building dynamic and responsive analytics dashboards. This article explores how Node.js and Elasticsearch can be used to create real-time analytics dashboards, with practical examples and code snippets.
Understanding Real-Time Analytics Dashboards
Real-time analytics dashboards provide up-to-date insights into data as it is generated. These dashboards are used for monitoring system performance, user activity, and various other metrics in real-time. Building such dashboards involves collecting, processing, and visualizing data quickly and efficiently.
Using Node.js for Real-Time Data Processing
Node.js is well-suited for building real-time applications due to its non-blocking, event-driven architecture. It can handle numerous concurrent connections efficiently, making it ideal for real-time data processing and communication.
1. Setting Up a Node.js Server
First, set up a basic Node.js server that will handle data processing and communication with Elasticsearch.
```javascript const express = require('express'); const app = express(); const port = 3000; app.use(express.json()); app.get('/', (req, res) => { res.send('Real-Time Analytics Dashboard'); }); app.listen(port, () => { console.log(`Server running at http://localhost:${port}`); }); ```
2. Integrating Elasticsearch with Node.js
Elasticsearch is a powerful search and analytics engine that allows for fast searches and complex queries. To integrate Elasticsearch with Node.js, use the `@elastic/elasticsearch` client library.
First, install the Elasticsearch client:
```bash npm install @elastic/elasticsearch ```
Then, connect to Elasticsearch and perform basic operations:
```javascript const { Client } = require('@elastic/elasticsearch'); const client = new Client({ node: 'http://localhost:9200' }); app.post('/index-data', async (req, res) => { const { index, body } = req.body; try { const result = await client.index({ index, body }); res.status(200).json(result.body); } catch (err) { res.status(500).json({ error: err.message }); } }); ```
Analyzing and Visualizing Data
With data indexed in Elasticsearch, you can now query and visualize it to create meaningful insights.
3. Querying Elasticsearch for Real-Time Data
Perform queries to fetch and analyze data. For example, to fetch data for a specific metric:
```javascript app.get('/fetch-data', async (req, res) => { const { index, query } = req.query; try { const result = await client.search({ index, body: { query: { match: { metric: query } } } }); res.status(200).json(result.body.hits.hits); } catch (err) { res.status(500).json({ error: err.message }); } }); ```
4. Visualizing Data with a Front-End Framework
To display the data, use a front-end framework like React or Vue.js. For instance, you could use React with a library like `chart.js` to create interactive charts.
Install necessary packages:
```bash npm install react chart.js ```
Create a simple chart component:
```javascript import React from 'react'; import { Line } from 'react-chartjs-2'; import { Chart as ChartJS, LineElement, CategoryScale, LinearScale, Title } from 'chart.js'; ChartJS.register(LineElement, CategoryScale, LinearScale, Title); const LineChart = ({ data }) => { const chartData = { labels: data.map(item => item.timestamp), datasets: [ { label: 'Metric Value', data: data.map(item => item.value), fill: false, borderColor: 'rgb(75, 192, 192)', tension: 0.1 } ] }; return <Line data={chartData} />; }; export default LineChart; ```
Real-Time Data Updates
To handle real-time updates, you can use WebSockets. For instance, use the `ws` library for WebSocket communication:
Install `ws`:
```bash npm install ws ```
Set up a WebSocket server:
```javascript const WebSocket = require('ws'); const wss = new WebSocket.Server({ port: 8080 }); wss.on('connection', ws => { console.log('New client connected'); ws.on('message', message => { console.log('Received:', message); }); ws.send('Welcome to the real-time analytics dashboard'); }); ```
Update the client to handle WebSocket messages:
```javascript const socket = new WebSocket('ws://localhost:8080'); socket.onmessage = event => { console.log('Message from server:', event.data); }; ```
Conclusion
Building real-time analytics dashboards with Node.js and Elasticsearch provides a robust solution for dynamic data visualization and analysis. By leveraging Node.js’s non-blocking architecture and Elasticsearch’s powerful search capabilities, you can create responsive and insightful dashboards that help monitor and analyze data in real-time.
Further Reading
Table of Contents
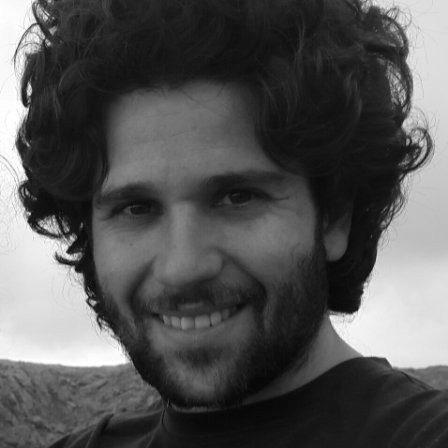
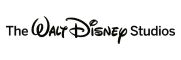