Error Handling in Node.js: Best Practices and Patterns
When it comes to building robust and reliable Node.js applications, proper error handling is a crucial aspect of the development process. Errors are an inevitable part of software development, and how you handle them can greatly impact the user experience and the overall stability of your application. In this blog post, we will delve into the best practices and patterns for error handling in Node.js, equipping you with the knowledge to write code that gracefully manages errors and provides meaningful feedback to users.
Table of Contents
1. Understanding the Importance of Error Handling
1.1. The Impact of Poor Error Handling
Neglecting proper error handling in your Node.js application can lead to a myriad of issues. Unhandled errors can crash your application, leaving users frustrated and potentially causing data loss. Moreover, poor error handling can compromise the security of your application by exposing sensitive information to malicious users.
1.2. Benefits of Effective Error Handling
Implementing effective error handling practices brings several advantages to your Node.js application:
- Enhanced User Experience: When errors occur, users are more likely to continue using an application that gracefully handles errors and provides clear instructions on how to proceed.
- Easier Debugging: Properly logging errors helps developers identify and fix issues more quickly. Error messages with relevant information guide developers towards the root cause of the problem.
- Improved Security: By handling errors properly, you can avoid leaking sensitive information that could potentially be exploited by attackers.
2. Common Sources of Errors in Node.js Applications
Node.js applications can encounter errors from various sources. Being aware of these sources helps you anticipate potential issues and design your error handling strategy accordingly.
2.1. Asynchronous Operations
Node.js heavily relies on asynchronous programming using callbacks, promises, and async/await. Errors occurring during asynchronous operations, such as database queries or network requests, need to be handled effectively.
javascript // Using async/await app.get('/user/:id', async (req, res, next) => { try { const user = await getUserFromDatabase(req.params.id); res.json(user); } catch (error) { next(error); // Pass the error to the global error handler } });
2.2. External Services and APIs
Interacting with external services and APIs introduces the possibility of errors due to network issues, rate limiting, or invalid responses.
javascript axios.get('https://api.example.com/data') .then(response => { // Process the response }) .catch(error => { // Handle the error });
2.3. Data Validation and Sanitization
Inadequate data validation can lead to unexpected errors. Validate and sanitize user input to prevent malformed data from causing issues.
javascript app.post('/create', (req, res, next) => { const { name, email } = req.body; if (!name || !email) { const error = new Error('Name and email are required.'); error.status = 400; return next(error); } // Create the user });
2.4. File System Operations
Working with the file system can result in errors due to missing files, insufficient permissions, or incorrect paths.
javascript fs.readFile('myfile.txt', 'utf8', (error, data) => { if (error) { // Handle the error } else { // Process the file data } });
3. Best Practices for Error Handling
3.1. Centralized Error Handling
Centralized error handling involves creating a global error handler to capture unhandled errors and ensure consistent error responses throughout the application.
3.1.1. Implementing a Global Error Handler
javascript app.use((error, req, res, next) => { console.error(error); // Log the error for debugging const statusCode = error.status || 500; const errorMessage = error.message || 'Internal Server Error'; res.status(statusCode).json({ error: errorMessage }); });
3.1.2. Logging Errors for Debugging
Logging errors with relevant information, such as the error message, stack trace, and request details, aids in diagnosing and resolving issues efficiently.
javascript app.use((error, req, res, next) => { console.error(`Error: ${error.message}`); console.error(`Stack trace: ${error.stack}`); console.error(`Request URL: ${req.url}`); console.error(`Request Method: ${req.method}`); // ...log other relevant details const statusCode = error.status || 500; const errorMessage = error.message || 'Internal Server Error'; res.status(statusCode).json({ error: errorMessage }); });
3.1.3. Sending Error Reports
For critical errors, consider integrating error reporting tools that send notifications to developers, enabling them to take immediate action.
3.2. Proper Use of Try-Catch
Try-catch blocks are essential for handling synchronous errors. They prevent application crashes and allow you to gracefully handle exceptions.
3.2.1. Handling Synchronous Errors
javascript app.post('/calculate', (req, res, next) => { try { const result = performCalculation(req.body.data); res.json({ result }); } catch (error) { next(error); } });
3.2.2. Avoiding Nested Try-Catch Blocks
Avoid nesting try-catch blocks excessively, as it can lead to confusing and hard-to-maintain code. Instead, use a single try-catch block at the appropriate level.
3.3. Handling Asynchronous Errors
Asynchronous errors require different handling approaches due to the nature of asynchronous programming.
3.3.1. Using Promises with .catch()
javascript async function fetchData(url) { try { const response = await axios.get(url); return response.data; } catch (error) { throw new Error(`Failed to fetch data: ${error.message}`); } }
3.3.2. Error-First Callbacks
For error-first callbacks, the convention is to pass the error as the first argument to the callback function.
javascript fs.readFile('myfile.txt', 'utf8', (error, data) => { if (error) { // Handle the error } else { // Process the file data } });
3.4. Custom Error Classes
Creating custom error classes allows you to provide more context and information about specific error scenarios.
3.4.1. Extending the Error Class
javascript class CustomError extends Error { constructor(message, statusCode) { super(message); this.name = this.constructor.name; this.statusCode = statusCode || 500; } }
3.4.2. Adding Custom Properties
Enhance error objects with custom properties that provide additional information about the error context.
javascript class ValidationError extends CustomError { constructor(message, field) { super(message, 400); this.field = field; } }
4. Design Patterns for Effective Error Handling
4.1. Error-Handling Middleware
In Express.js applications, error-handling middleware can be used to centralize error handling and avoid cluttering route handlers.
4.1.1. Integrating Error Middleware in Express.js
javascript app.use((req, res, next) => { const error = new Error('Not Found'); error.status = 404; next(error); }); app.use((error, req, res, next) => { res.status(error.status || 500); res.json({ error: error.message }); });
4.1.2. Centralized Error Handling with Middleware
javascript function errorHandler(error, req, res, next) { console.error(error); const statusCode = error.status || 500; const errorMessage = error.message || 'Internal Server Error'; res.status(statusCode).json({ error: errorMessage }); } app.use(errorHandler);
4.2. Circuit Breaker Pattern
The Circuit Breaker pattern helps prevent cascading failures by temporarily halting requests to a service that is experiencing issues.
4.2.1. Implementing the Circuit Breaker Pattern
javascript const circuitBreaker = require('circuit-breaker-js'); const options = { timeoutDuration: 5000, resetTimeout: 15000, errorThreshold: 50, }; const serviceCircuit = new circuitBreaker(someServiceFunction, options); app.get('/data', async (req, res, next) => { try { const result = await serviceCircuit.fire(); res.json(result); } catch (error) { next(error); } });
4.3. Retry Strategy
Implementing a retry strategy can help overcome temporary failures, such as network timeouts or rate limiting.
4.3.1. Implementing Automatic Retries
javascript const maxRetries = 3; async function fetchDataWithRetries(url, retries = maxRetries) { try { const response = await axios.get(url); return response.data; } catch (error) { if (retries > 0) { return fetchDataWithRetries(url, retries - 1); } throw new Error(`Failed to fetch data after ${maxRetries} retries.`); } }
4.3.2. Exponential Backoff for Retry Attempts
Implementing exponential backoff between retry attempts helps prevent overwhelming the server after a temporary failure.
javascript async function fetchDataWithExponentialBackoff(url, retries = maxRetries, delay = 1000) { try { const response = await axios.get(url); return response.data; } catch (error) { if (retries > 0) { await new Promise(resolve => setTimeout(resolve, delay)); return fetchDataWithExponentialBackoff(url, retries - 1, delay * 2); } throw new Error(`Failed to fetch data after ${maxRetries} retries.`); } }
Conclusion
Effective error handling is not just a feature of well-architected applications; it’s a necessity. By following the best practices and patterns outlined in this guide, you’ll be equipped to build Node.js applications that can gracefully manage errors, maintain a smooth user experience, and ensure the stability of your software. Remember, error handling is a continuous process that requires constant refinement as your application evolves, so keep honing your skills to create robust and reliable applications.
Table of Contents
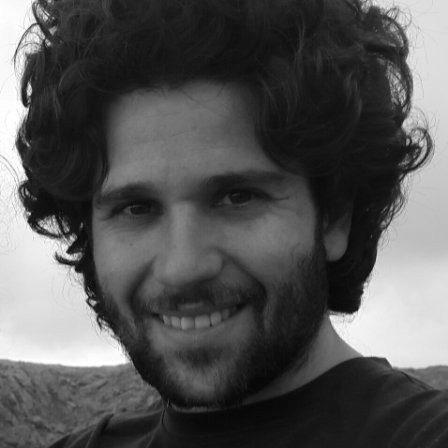
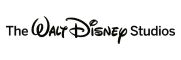