Taking Web Development to New Heights with Practical Node.js Applications
Node.js has established itself as a powerful, efficient, and versatile technology for developing web applications. Built on Chrome’s V8 JavaScript engine, Node.js is open-source and cross-platform, leading to its widespread popularity among developers. This has also sparked a growing trend where businesses opt to hire Node.js developers to harness this robust platform effectively. This blog post aims to showcase the power of Node.js and how hiring skilled Node.js developers can help leverage its full potential through practical examples.
What is Node.js?
Node.js is a JavaScript runtime built on Chrome’s V8 JavaScript engine. It allows developers to use JavaScript to write command-line tools and for server-side scripting, extending the reach of JavaScript from the browser to the server. Node.js enables the development of scalable network applications, making it an ideal choice for real-time applications such as chats, gaming servers, collaboration tools, and more.
Why Node.js?
Node.js offers numerous advantages:
- Event-driven and non-blocking I/O model: This makes Node.js lightweight and efficient, perfect for data-intensive real-time applications that run across distributed devices.
- Single-threaded: Although JavaScript is single-threaded, Node.js uses multiple threads in the background to execute asynchronous tasks.
- NPM (Node Package Manager): With the largest ecosystem of open-source libraries in the world, it provides developers with numerous packages for faster and effective development.
Now let’s delve into some practical applications of Node.js and its capabilities.
Example 1: Building a Simple Web Server
One of the most basic uses of Node.js is to create a web server. It just takes a few lines of code:
```javascript const http = require('http'); const server = http.createServer((req, res) => { res.statusCode = 200; res.setHeader('Content-Type', 'text/plain'); res.end('Hello World\n'); }); server.listen(3000, '127.0.0.1', () => { console.log('Server running at http://127.0.0.1:3000/'); }); ```
When you run this script, it starts a server at http://127.0.0.1:3000 and responds with “Hello World” for every request.
Example 2: Building a Real-time Chat Application
Node.js, in conjunction with Socket.IO, a library for real-time web applications, enables you to create complex applications like chat rooms effortlessly. It works on every platform, browser, or device, focusing equally on reliability and speed. Here is a simple implementation of a chat application:
Server side (server.js):
```javascript const express = require('express'); const socketIO = require('socket.io'); const http = require('http'); const app = express(); const server = http.createServer(app); const io = socketIO(server); io.on('connection', (socket) => { console.log('User connected'); socket.on('disconnect', () => { console.log('User disconnected'); }); socket.on('chat message', (msg) => { io.emit('chat message', msg); }); }); server.listen(3000, () => { console.log('Server running at http://localhost:3000'); }); ```
Client side (index.html):
```html <!DOCTYPE html> <html> <body> <ul id="messages"></ul> <form action=""> <input id="m" autocomplete="off" /><button>Send</button> </form> <script src="/socket.io/socket.io.js"></script> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> <script> $(function () { const socket = io(); $('form').submit(function(e){ e.preventDefault(); // prevents page reloading socket.emit('chat message', $('#m').val()); $('#m').val(''); return false; }); socket.on('chat message', function(msg){ $('#messages').append($('<li>').text(msg)); }); }); </script> </body> </html> ```
This example demonstrates the capability of Node.js to handle real-time applications efficiently.
Example 3: Data Streaming
Data streaming is another area where Node.js excels. You can process files while they’re still being uploaded, as the data comes in through a Stream. This could be useful for real-time audio or video encoding, or even file uploads.
```javascript const fs = require('fs'); const http = require('http'); http.createServer((req, res) => { // This opens up the writeable stream to `output` const writeStream = fs.createWriteStream('./output'); // This pipes the POST data to the file req.pipe(writeStream); // After all the data is saved, respond with a simple html form so they can post more data req.on('end', () => { res.writeHead(200, {"content-type":"text/html"}); res.end('<form method="POST" action="/upload"><input name="upload" type="file"/></form>'); }); // This is here incase any errors occur writeStream.on('error', (err) => { console.log(err); }); }).listen(3000); ```
In this example, the server creates a writable stream to an output file, and as soon as you start posting data to the server, it starts writing it to the output file.
Conclusion
These examples are just the tip of the iceberg, illustrating how Node.js can be leveraged for creating robust and scalable applications. Its non-blocking, event-driven architecture makes it an excellent choice for developing high-performance applications, while its easy-to-understand syntax ensures a more seamless and productive development experience.
With the power of Node.js, you can build complex web applications such as real-time chats, gaming servers, interactive websites, and so much more. The possibilities are endless. This is why many organizations opt to hire Node.js developers, to fully utilize this versatile platform. No wonder why big giants like LinkedIn, Walmart, Uber, Netflix, and NASA have trusted Node.js in their tech stacks.
Whether you are a beginner or an expert in JavaScript, Node.js is a technology worth exploring and mastering. Hiring skilled Node.js developers can give your business the technological edge it needs, allowing you to embrace the full potential of Node.js and broaden your horizons in the realm of web development.
Table of Contents
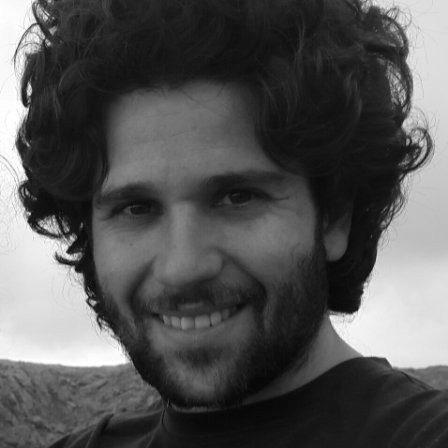
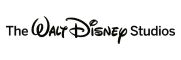