Node.js Q & A
How do you handle file compression in Node.js?
In Node.js, file compression is commonly handled using the zlib module, which provides utilities for compressing and decompressing data using various compression algorithms such as gzip and deflate. Here’s how you can handle file compression in Node.js:
- Compressing Files:
-
-
- Use the zlib.gzip() method to compress files using the gzip compression algorithm. Read the file contents using Node.js file system APIs (fs) or streams, and pipe the data to a writable stream created with zlib.createGzip().
- Example:
- javascript
- Copy code
- const fs = require(‘fs’);
- const zlib = require(‘zlib’);
- const input = fs.createReadStream(‘input.txt’);
- const output = fs.createWriteStream(‘input.txt.gz’);
- input.pipe(zlib.createGzip()).pipe(output);
-
- Decompressing Files:
-
- Use the zlib.gunzip() method to decompress gzip-compressed files. Read the compressed file contents using file system APIs or streams, and pipe the data to a writable stream created with zlib.createGunzip().
- Example:
- javascript
- Copy code
const fs = require('fs'); const zlib = require('zlib'); const input = fs.createReadStream('input.txt.gz'); const output = fs.createWriteStream('input.txt'); input.pipe(zlib.createGunzip()).pipe(output);
- Handling Errors:
-
-
- Implement error handling to catch and handle compression/decompression errors gracefully. Listen for error events on streams and handle errors using try-catch blocks or error callback functions.
-
- Asynchronous Compression:
-
-
- Use asynchronous file operations and stream APIs to perform file compression asynchronously without blocking the event loop. This ensures that the application remains responsive during compression/decompression operations.
-
- Configuration Options:
-
-
- Customize compression options such as compression level, window size, and strategy based on performance requirements and use cases. Pass options as parameters to compression/decompression functions to fine-tune compression parameters.
-
- Integration with File System:
-
-
- Integrate file compression with Node.js file system APIs (fs) to read/write compressed files directly from/to the file system. Use fs.createReadStream() and fs.createWriteStream() to create readable and writable streams for file compression operations.
-
- Buffer Compression:
-
-
- Perform buffer-based compression/decompression using zlib.deflate() and zlib.inflate() methods for in-memory compression operations. Read file contents into buffers, compress/decompress buffers using zlib functions, and write compressed/decompressed buffers to files or streams.
-
- Optimizing Performance:
-
- Optimize file compression performance by choosing appropriate compression algorithms, compression levels, and buffer sizes. Measure and benchmark compression/decompression operations to identify bottlenecks and optimize performance.
By following these best practices, developers can effectively handle file compression in Node.js applications, reducing file sizes, optimizing storage space, and improving data transfer efficiency.
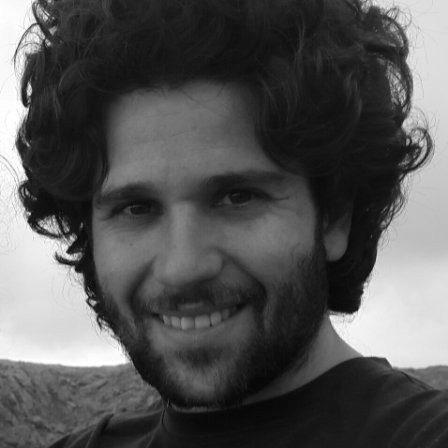
Previously at
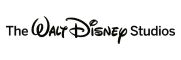
Experienced Principal Engineer and Fullstack Developer with a strong focus on Node.js. Over 5 years of Node.js development experience.