Exploring Full-Stack JavaScript Development with Node.js and React
Full-stack JavaScript development involves using JavaScript for both the front-end and back-end of web applications, creating a seamless and efficient development experience. Node.js and React are two powerful tools in this ecosystem, enabling developers to build scalable and interactive applications with a unified language.
Utilizing Node.js for Back-End Development
Node.js is a runtime environment that allows you to run JavaScript on the server side. Its non-blocking I/O and event-driven architecture make it well-suited for handling concurrent operations and building scalable network applications.
Example: Building a Simple API with Node.js
Here’s a basic example of setting up an Express server to create a RESTful API.
```javascript const express = require('express'); const app = express(); const port = 3000; app.use(express.json()); app.get('/api/greeting', (req, res) => { res.json({ message: 'Hello, World!' }); }); app.post('/api/data', (req, res) => { const data = req.body; res.json({ received: data }); }); app.listen(port, () => { console.log(`Server is running on http://localhost:${port}`); }); ```
React for Front-End Development
React is a JavaScript library for building user interfaces, particularly single-page applications with dynamic content. Its component-based architecture and virtual DOM provide a fast and efficient way to create interactive UIs.
Example: Creating a Basic React Component
Here’s how you can create a simple React component to display a greeting message.
```javascript import React from 'react'; import ReactDOM from 'react-dom'; function Greeting() { return <h1>Hello, World!</h1>; } ReactDOM.render(<Greeting />, document.getElementById('root')); ```
Integrating Node.js and React
Combining Node.js and React allows you to create a full-stack JavaScript application where Node.js handles the server-side logic, and React manages the client-side interface.
Example: Fetching Data from Node.js API in React
Here’s how you might fetch data from a Node.js API and display it in a React component.
```javascript import React, { useState, useEffect } from 'react'; function App() { const [message, setMessage] = useState(''); useEffect(() => { fetch('/api/greeting') .then(response => response.json()) .then(data => setMessage(data.message)); }, []); return <h1>{message}</h1>; } export default App; ```
Deploying a Full-Stack Application
Deploying a full-stack JavaScript application involves setting up both the front-end and back-end to work together in a production environment. You can use platforms like Heroku, Vercel, or AWS for deployment.
Example: Deploying to Heroku
- Create a Heroku Account: Sign up for a free account at [Heroku](https://www.heroku.com/).
- Install the Heroku CLI: Follow the installation instructions [here](https://devcenter.heroku.com/articles/heroku-cli).
- Prepare Your Application: Ensure that your application has a `Procfile` for Heroku to understand how to run it.
- Deploy: Use the following commands to deploy your application:
```bash git init heroku create git add . git commit -m "Initial commit" git push heroku master ```
Conclusion
Full-stack JavaScript development with Node.js and React provides a cohesive and efficient approach to building modern web applications. By leveraging these technologies, developers can create scalable, interactive, and dynamic applications with a single language throughout the stack.
Further Reading:
Table of Contents
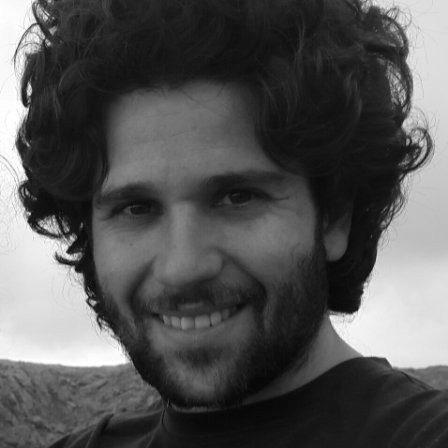
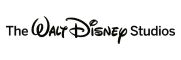