Exploring Functional Programming in Node.js: Concepts and Examples
Understanding Functional Programming
At its core, functional programming emphasizes the use of pure functions, immutability, and higher-order functions. Let’s break down these concepts:
- Pure Functions: A pure function is a function that, given the same input, will always return the same output without causing any side effects. This predictability makes pure functions easier to reason about and test.
- Immutability: Immutability refers to the practice of not changing data after it’s been created. Instead of modifying existing data structures, immutable programming encourages creating new ones. This helps prevent unintended changes and facilitates concurrency.
- Higher-Order Functions: Higher-order functions are functions that can accept other functions as arguments or return functions as results. This allows for a more modular and composable codebase.
Applying Functional Programming in Node.js
Now, let’s see how these principles translate into practical examples within a Node.js environment:
Map, Filter, and Reduce
These higher-order functions are fundamental building blocks in functional programming. They allow for concise and expressive manipulation of arrays.
const numbers = [1, 2, 3, 4, 5]; // Map: Transform each element in the array const doubled = numbers.map(num => num * 2); // Filter: Filter elements based on a condition const evens = numbers.filter(num => num % 2 === 0); // Reduce: Reduce the array to a single value const sum = numbers.reduce((acc, curr) => acc + curr, 0);
Immutable Data Structures
Libraries like Immutable.js or simply adhering to immutable programming principles can help maintain data integrity and facilitate state management in Node.js applications.
const { Map } = require('immutable'); let state = Map({ count: 0 }); const increment = currentState => currentState.update('count', count => count + 1); state = increment(state);
Currying and Partial Application
These techniques enable the creation of specialized functions by partially applying arguments to existing functions, promoting code reuse and composability.
// Currying const multiply = x => y => x * y; const double = multiply(2); const triple = multiply(3); // Partial Application const add = (a, b, c) => a + b + c; const add5 = add.bind(null, 5);
Real-World Applications
Functional programming concepts aren’t just theoretical; they offer tangible benefits in real-world scenarios. Here are a few examples:
Concurrency and Parallelism
Immutable data structures and pure functions make it easier to reason about concurrent operations, leading to more scalable and efficient Node.js applications.
Testing and Debugging
The predictability of pure functions simplifies unit testing and debugging, as there are no hidden dependencies or side effects to worry about.
Code Maintainability
Functional programming promotes modular, reusable code, which leads to easier maintenance and refactoring as applications grow.
Conclusion
In conclusion, functional programming offers a paradigm shift in how we approach software development, and its principles are highly applicable in the context of Node.js. By embracing pure functions, immutability, and higher-order functions, developers can write more predictable, scalable, and maintainable code. Whether you’re building web servers, APIs, or microservices, incorporating functional programming concepts can elevate your Node.js development to new heights.
For Further Reading on Functional Programming in JavaScript, Check Out These Resources:
- Functional-Light JavaScript by Kyle Simpson
- Ramda – A practical functional library for JavaScript programmers
- Immutable.js Documentation – Official documentation for Immutable.js
Happy coding!
Table of Contents
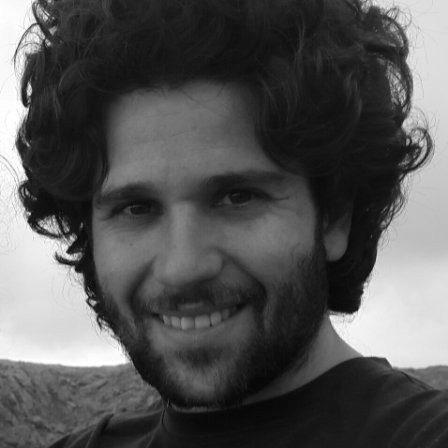
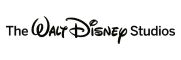