Building GraphQL APIs with Node.js and Apollo Server
In the world of modern web development, APIs play a crucial role in connecting frontend and backend systems. Traditional REST APIs have long been the go-to solution, but a more efficient and flexible alternative has emerged: GraphQL. Developed by Facebook in 2012 and open-sourced in 2015, GraphQL allows developers to fetch exactly the data they need, reducing over-fetching and under-fetching of data. In this tutorial, we will explore how to build GraphQL APIs using Node.js and Apollo Server, a popular GraphQL server implementation.
Table of Contents
1. Understanding GraphQL
GraphQL is a query language and runtime for APIs that allows clients to request only the data they need. Unlike traditional REST APIs, where endpoints often return fixed data structures, GraphQL enables clients to define the shape of the response they require. This eliminates over-fetching (retrieving more data than needed) and under-fetching (retrieving insufficient data). GraphQL also provides a strong typing system through schemas, enabling clear communication between frontend and backend teams.
2. Key Advantages of GraphQL
- Flexibility: Clients can request specific fields and nested data, reducing unnecessary data transfers and enhancing performance.
- Versioning: GraphQL avoids versioning issues by allowing clients to specify exactly what data they need, reducing the risk of breaking changes.
- Efficiency: With a single request, clients can retrieve multiple types of data, minimizing round-trips between the client and server.
- Introspection: GraphQL schemas are self-documenting, making it easier to understand the available types, fields, and operations.
- Real-time Data: GraphQL supports subscriptions, enabling real-time data updates by establishing a persistent connection between clients and the server.
3. GraphQL Schema and Types
A GraphQL schema defines the types of data that can be queried and the relationships between them. Each type has fields that represent the data to be retrieved. Let’s consider an example schema for a blog system:
graphql type Post { id: ID! title: String! content: String! author: User! } type User { id: ID! username: String! email: String! posts: [Post!]! } type Query { post(id: ID!): Post user(id: ID!): User posts: [Post!]! }
In this schema, we have three types: Post, User, and Query. The Query type defines the entry points for retrieving data. The User type has a relationship with the Post type through the posts field, demonstrating how GraphQL handles complex data structures.
4. Setting Up the Project
Before we dive into creating the GraphQL API, we need to set up our development environment.
4.1. Node.js Installation
If you don’t have Node.js installed, head over to the official Node.js website and download the latest LTS version.
4.2. Project Initialization
Create a new project directory and navigate to it in your terminal. Run the following command to initialize a new Node.js project:
bash npm init -y
4.3. Installing Dependencies
We will need several packages to build our GraphQL API. Install them using the following command:
bash npm install express apollo-server graphql
5. Creating the GraphQL Schema
The heart of any GraphQL API is its schema. The schema defines the types of data that can be queried and the relationships between them.
5.1. Defining Types
In your project directory, create a file named schema.js to define your GraphQL types. Here’s how the types from our previous example would look:
javascript const { gql } = require('apollo-server'); const typeDefs = gql` type Post { id: ID! title: String! content: String! author: User! } type User { id: ID! username: String! email: String! posts: [Post!]! } type Query { post(id: ID!): Post user(id: ID!): User posts: [Post!]! } `; module.exports = typeDefs;
5.2. Adding Resolvers
Resolvers are functions that define how to fetch data for each field in a type. Create a file named resolvers.js in your project directory and define resolvers for the types in your schema:
javascript const resolvers = { Query: { post: (parent, { id }, context, info) => { // Fetch and return post data based on the provided id }, user: (parent, { id }, context, info) => { // Fetch and return user data based on the provided id }, posts: (parent, args, context, info) => { // Fetch and return all posts }, }, User: { posts: (parent, args, context, info) => { // Fetch and return posts authored by the user }, }, }; module.exports = resolvers;
5.3. Combining into Schema
In your main server file (let’s name it index.js), combine the schema and resolvers to create the Apollo Server instance:
javascript const { ApolloServer } = require('apollo-server'); const typeDefs = require('./schema'); const resolvers = require('./resolvers'); const server = new ApolloServer({ typeDefs, resolvers }); server.listen().then(({ url }) => { console.log(`Server ready at ${url}`); });
6. Setting Up Apollo Server
With the schema and resolvers in place, it’s time to set up Apollo Server to handle GraphQL requests.
6.1. Installing Apollo Server
We’ve already installed the necessary packages, including apollo-server, so we can move on to creating the server instance.
6.2. Creating the Server Instance
In the index.js file, import ApolloServer and create an instance, passing in the typeDefs and resolvers:
javascript const { ApolloServer } = require('apollo-server'); const server = new ApolloServer({ typeDefs, resolvers });
6.3. Connecting Schema and Server
Next, start the server by calling the listen method and passing in a callback function to indicate that the server is running:
javascript server.listen().then(({ url }) => { console.log(`Server ready at ${url}`); });
7. Querying Data with GraphQL
Now that our Apollo Server is up and running, let’s start querying data using GraphQL.
7.1. Writing Basic Queries
In your preferred GraphQL client (such as Apollo Client, Postman, or GraphQL Playground), you can now send queries to your server. Here’s an example query to retrieve a post by its ID:
graphql query { post(id: "1") { title content author { username } } }
7.2. Query Variables
GraphQL supports query variables, allowing you to pass dynamic values to your queries. Modify the query to use a variable for the post ID:
graphql query GetPost($postId: ID!) { post(id: $postId) { title content author { username } } }
Then, in your client, you can provide the value for the postId variable.
7.3. Query Fragments
Query fragments enable you to reuse parts of a query in multiple places. Define a fragment and then use it within your queries:
graphql fragment PostDetails on Post { title content author { username } } query { post(id: "1") { ...PostDetails } }
8. Mutating Data with GraphQL
GraphQL not only allows querying data but also modifying it through mutations.
8.1. Creating Mutations
In your schema, define mutation types and their input types. For instance, let’s create a mutation to add a new post:
graphql type Mutation { createPost(input: CreatePostInput!): Post! } input CreatePostInput { title: String! content: String! authorId: ID! }
8.2. Input Types
Mutation arguments can be complex objects, so GraphQL provides input types to ensure consistency and validation. In this example, CreatePostInput is an input type for the createPost mutation.
8.3. Handling Mutations on the Server
In your resolvers, implement the mutation function and handle the creation of a new post:
javascript const resolvers = { Mutation: { createPost: (parent, { input }, context, info) => { // Create and return a new post }, }, // ... };
9. Pagination and Filtering
For APIs that deal with large amounts of data, implementing pagination and filtering mechanisms is essential.
9.1. Implementing Pagination
Add pagination arguments to your queries, such as first and after, to control the number of items returned and where to start from:
graphql query { posts(first: 10, after: "cursor") { id title } }
9.2. Adding Filtering and Sorting
Implement arguments for filtering and sorting to enhance data retrieval:
graphql query { posts( first: 10 after: "cursor" filter: { authorId: "user123" } sort: { field: "createdAt", order: ASC } ) { id title } }
10. Authentication and Authorization
Securing your GraphQL API is crucial. Implementing authentication and authorization ensures that only authorized users can access certain data or perform specific actions.
10.1. Securing the GraphQL API
Use HTTPS to encrypt data transmitted between clients and the server. Additionally, implement authentication mechanisms such as API keys, JWT, or OAuth.
10.2. Implementing Authentication
In your server setup, integrate authentication middleware to verify user identity before processing requests.
10.3. Role-Based Authorization
Implement role-based access control to ensure that only users with the appropriate roles can perform certain actions or access specific data.
11. Error Handling
Handling errors effectively improves the user experience and helps developers diagnose and fix issues.
11.1. Handling Errors in GraphQL
GraphQL provides a way to return both data and errors in a single response. Each field can have its own set of errors if necessary.
11.2. Custom Error Messages
You can provide custom error messages to provide more context to clients when errors occur.
11.3. Error Bubbling
Errors in nested fields can be propagated to their parent fields, giving you a clear overview of where the issue originated.
12. Caching and Performance
Caching plays a significant role in optimizing the performance of your GraphQL API.
12.1. Caching with Apollo Server
Apollo Server supports various caching strategies, including in-memory caching and integration with popular caching solutions like Redis.
12.2. Optimizing Performance
Consider using data loaders to batch and cache database queries, reducing the number of round-trips to the database.
12.3. Using DataLoader for Efficient Data Loading
DataLoader is a utility that batches and caches database queries, preventing the “N+1 query” problem often encountered with GraphQL APIs.
13. Testing GraphQL APIs
Unit and integration testing are essential to ensure your GraphQL API functions as expected.
13.1. Unit Testing Resolvers
Write unit tests for your resolvers to verify that they return the correct data.
13.2. Integration Testing the API
Test the entire API by sending queries and mutations to the server and asserting the expected outcomes.
13.3. Using Testing Libraries
Use testing libraries like Jest, Mocha, or Chai to write and run tests for your GraphQL API.
14. Deployment and Best Practices
Deploying your GraphQL API and following best practices ensure that your application runs smoothly in production.
14.1. Deployment Options
Choose a deployment strategy that suits your project, whether it’s deploying to traditional servers, cloud platforms, or serverless architectures.
14.2. Monitoring and Logging
Implement monitoring and logging to track API performance, detect anomalies, and troubleshoot issues.
14.3. Best Practices for GraphQL Development
- Keep the Schema Simple: Design a clear and intuitive schema that meets the needs of your application without becoming overly complex.
- Use DataLoader for Efficient Data Fetching: Utilize DataLoader to batch and cache database queries for optimized performance.
- Implement Caching: Take advantage of caching mechanisms to reduce unnecessary database queries and improve response times.
- Secure Your API: Implement authentication and authorization to ensure data security and protect against unauthorized access.
- Test Thoroughly: Write unit and integration tests to ensure the reliability and correctness of your API.
- Document Your Schema: Provide clear and comprehensive documentation for your schema and resolvers to aid other developers.
- Optimize Queries: Pay attention to query efficiency, avoiding over-fetching and under-fetching of data.
- Keep an Eye on Performance: Monitor your API’s performance and identify bottlenecks or areas for optimization.
Conclusion
Building GraphQL APIs with Node.js and Apollo Server empowers developers to create efficient, flexible, and well-documented APIs. By leveraging GraphQL’s querying capabilities, schema definition, and resolvers, you can design APIs that provide exactly the data clients need. With proper authentication, authorization, and error handling, along with best practices in deployment and performance optimization, you can ensure your GraphQL API is robust, secure, and responsive. As you embark on your GraphQL journey, keep experimenting, learning, and refining your skills to craft exceptional APIs that power modern applications.
Table of Contents
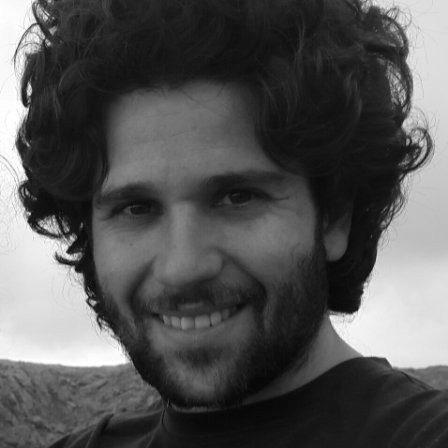
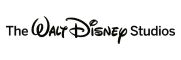