Developing GraphQL Servers with Node.js and Prisma
GraphQL has become a popular choice for building APIs due to its flexibility and efficiency in querying data. When combined with Node.js and Prisma, developers can create powerful and scalable GraphQL servers. This article explores how to leverage Node.js and Prisma for developing GraphQL servers, providing practical examples and insights.
Understanding GraphQL
GraphQL is a query language for APIs and a server-side runtime that provides a more flexible and efficient alternative to REST. It allows clients to request exactly the data they need, minimizing over-fetching and under-fetching.
Setting Up Your Development Environment
Before diving into code, ensure you have Node.js and npm (Node Package Manager) installed. You can download them from the [official Node.js website](https://nodejs.org/).
1. Setting Up a Node.js Project
First, create a new Node.js project and install the necessary dependencies.
```bash mkdir graphql-server cd graphql-server npm init -y npm install @prisma/client graphql express express-graphql prisma ```
2. Initializing Prisma
Prisma is an ORM that simplifies database interactions. Start by setting up Prisma in your project.
```bash npx prisma init ```
This command creates a `prisma` folder with a `schema.prisma` file and a `.env` file for environment variables.
3. Defining Your Prisma Schema
Edit the `schema.prisma` file to define your data models. Here’s an example schema for a simple blog:
```prisma datasource db { provider = "postgresql" url = env("DATABASE_URL") } generator client { provider = "prisma-client-js" } model Post { id Int @id @default(autoincrement()) title String body String } ```
4. Generating the Prisma Client
Generate the Prisma client to interact with your database.
```bash npx prisma generate ```
5. Creating a GraphQL Schema
Define your GraphQL schema and resolvers. Create a new file named `schema.graphql`.
```graphql type Query { posts: [Post!]! } type Post { id: ID! title: String! body: String! } ```
6. Setting Up Express and GraphQL
Create an `index.js` file to set up an Express server and integrate GraphQL.
```javascript const express = require('express'); const { ApolloServer, gql } = require('apollo-server-express'); const { PrismaClient } = require('@prisma/client'); const prisma = new PrismaClient(); const typeDefs = gql` type Query { posts: [Post!]! } type Post { id: ID! title: String! body: String! } `; const resolvers = { Query: { posts: async () => await prisma.post.findMany(), }, }; const server = new ApolloServer({ typeDefs, resolvers }); const app = express(); server.applyMiddleware({ app }); app.listen({ port: 4000 }, () => console.log(`Server running at http://localhost:4000${server.graphqlPath}`) ); ```
7. Running Your GraphQL Server
Start your server and test it using a GraphQL client or playground.
```bash node index.js ```
Visit `http://localhost:4000/graphql` to interact with your GraphQL API.
8. Integrating with Frontend Applications
You can use GraphQL clients like Apollo Client or Relay to interact with your GraphQL server from frontend applications. Here’s a basic example using Apollo Client:
```bash npm install @apollo/client graphql ``` ```javascript import { ApolloClient, InMemoryCache, gql } from '@apollo/client'; const client = new ApolloClient({ uri: 'http://localhost:4000/graphql', cache: new InMemoryCache(), }); client .query({ query: gql` query { posts { id title body } } `, }) .then((result) => console.log(result)); ```
Conclusion
By combining Node.js and Prisma with GraphQL, you can build efficient and scalable APIs that streamline data access and enhance performance. This setup provides a powerful foundation for developing modern applications with robust data handling capabilities.
Further Reading:
Table of Contents
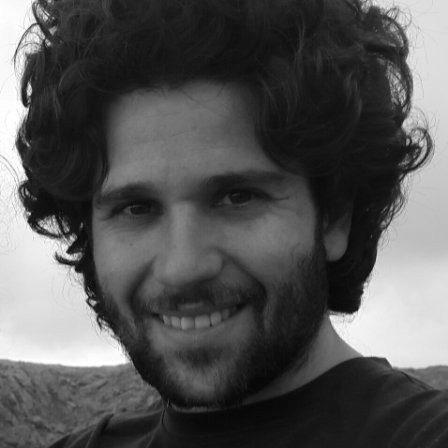
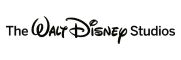