What is GraphQL and how is it used in Node.js?
GraphQL is a query language and runtime for executing queries against APIs, developed by Facebook in 2012 and open-sourced in 2015. Unlike traditional RESTful APIs, where clients retrieve data from predefined endpoints with fixed response structures, GraphQL allows clients to specify the exact data requirements in a single query, enabling more efficient and flexible data fetching.
The key features of GraphQL include:
- Declarative Data Fetching: Clients can specify the exact data they need, including nested and related data, in a single GraphQL query, eliminating over-fetching and under-fetching of data common in RESTful APIs.
- Strong Typing System: GraphQL APIs are strongly typed, allowing you to define a clear schema with types, fields, and relationships between data entities. This enables automatic validation, introspection, and documentation generation for the API.
- Single Endpoint: GraphQL APIs expose a single endpoint for all queries, mutations, and subscriptions, simplifying client-server interactions and reducing network overhead compared to multiple REST endpoints.
- Real-time Data: GraphQL supports real-time data with subscriptions, allowing clients to subscribe to data changes and receive updates in real-time as they occur on the server.
In Node.js, you can implement GraphQL APIs using various libraries and frameworks, such as Apollo Server, Express-GraphQL, or GraphQL Yoga. These libraries provide tools and middleware for defining GraphQL schemas, handling GraphQL queries and mutations, resolving data from backend services, and integrating with existing Node.js applications.
Here’s a basic example of setting up a GraphQL server using Apollo Server in Node.js:
const { ApolloServer, gql } = require('apollo-server'); // Define GraphQL schema const typeDefs = gql` type Query { hello: String } `; // Define resolver functions const resolvers = { Query: { hello: () => 'Hello, world!' } }; // Create Apollo Server instance const server = new ApolloServer({ typeDefs, resolvers }); // Start the server server.listen().then(({ url }) => { console.log(`GraphQL server running at ${url}`); });
In this example, we define a simple GraphQL schema with a single query field (hello) that returns a string. We then define resolver functions that handle the query execution logic and return the corresponding data.
Finally, we create an Apollo Server instance with the schema and resolvers, and start the server, making the GraphQL API available at the specified URL.
By using GraphQL in Node.js, developers can build efficient, flexible, and powerful APIs that meet the precise data requirements of client applications, resulting in improved developer productivity and enhanced user experiences.
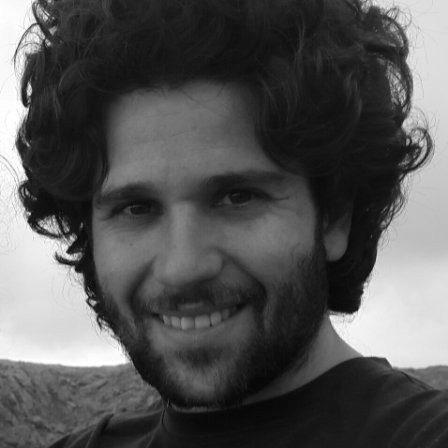
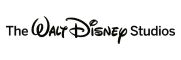