How do you handle CORS in Node.js?
Cross-Origin Resource Sharing (CORS) is a security mechanism implemented by web browsers to restrict web pages from making requests to a different origin domain. In Node.js, CORS issues often arise when developing APIs or web applications that need to interact with resources from different domains. Here’s how you can handle CORS in Node.js:
Use CORS Middleware:
Utilize middleware packages like cors in your Node.js application to handle CORS headers automatically. Install the cors package via npm:
Copy code
npm install cors
Integrate the cors middleware into your Express.js application to enable CORS handling for all routes:
javascript
Copy code
const express = require('express'); const cors = require('cors'); const app = express(); app.use(cors());
Configure CORS Options:
Customize CORS behavior by specifying configuration options such as allowed origins, allowed methods, allowed headers, and preflight request handling. Configure CORS options based on your application’s security requirements and CORS policies.
javascript
Copy code
app.use(cors({ origin: 'https://example.com', methods: 'GET,POST', allowedHeaders: 'Content-Type,Authorization', }));
Enable CORS Headers:
Manually set CORS headers in your Express.js routes or middleware functions to control CORS behavior for specific endpoints or responses. Set Access-Control-Allow-Origin, Access-Control-Allow-Methods, Access-Control-Allow-Headers, and other CORS headers as needed.
javascript
Copy code
app.get('/api/data', (req, res) => { res.setHeader('Access-Control-Allow-Origin', 'https://example.com'); res.json({ message: 'Data from API' }); });
Handle Preflight Requests:
Respond to CORS preflight requests (OPTIONS requests) sent by browsers to check CORS permissions before making actual requests. Implement middleware or route handlers to handle preflight requests and provide appropriate CORS headers and responses.
javascript
Copy code
app.options('/api/data', (req, res) => { res.setHeader('Access-Control-Allow-Origin', 'https://example.com'); res.setHeader('Access-Control-Allow-Methods', 'GET,POST'); res.setHeader('Access-Control-Allow-Headers', 'Content-Type,Authorization'); res.status(204).end(); });
Implement CORS Whitelisting:
Whitelist specific origins, methods, or headers to restrict CORS access to trusted domains and prevent unauthorized cross-origin requests. Configure CORS options to only allow requests from specified origins and reject requests from untrusted domains.
javascript
Copy code
const corsOptions = { origin: ['https://example.com', 'https://trusted.com'], methods: ['GET', 'POST'], }; app.use(cors(corsOptions));
Handle CORS Errors:
Implement error handling middleware to catch and respond to CORS-related errors or invalid CORS requests. Customize error responses, log error messages, and enforce CORS policies to protect against CORS vulnerabilities and security risks.
By implementing CORS handling in your Node.js application, you can ensure secure cross-origin communication, prevent unauthorized access to sensitive resources, and comply with CORS policies enforced by web browsers.
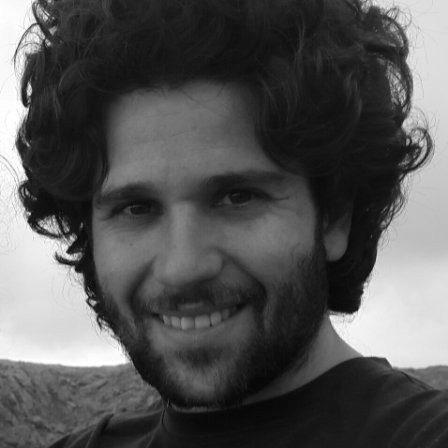
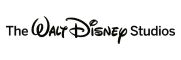