Node.js Q & A
What is the purpose of the HTTP/HTTPS module in Node.js?
The HTTP (Hypertext Transfer Protocol) and HTTPS (HTTP Secure) modules in Node.js provide APIs for creating HTTP/HTTPS servers and making HTTP requests, allowing developers to build web servers, web applications, and HTTP clients in Node.js.
Key purposes of the HTTP/HTTPS modules include:
- Server Creation:
-
- The HTTP/HTTPS modules allow developers to create HTTP/HTTPS servers in Node.js using the http.createServer() and https.createServer() methods. Developers can define request handlers to process incoming HTTP requests, handle routing, and generate HTTP responses.
- Example:
- javascript
- Copy code
const http = require('http'); const server = http.createServer((req, res) => { res.writeHead(200, { 'Content-Type': 'text/plain' }); res.end('Hello, World!'); }); server.listen(3000, () => { console.log('Server running on port 3000'); });
- Request Handling:
-
-
- HTTP/HTTPS servers handle incoming HTTP requests by invoking request handler functions provided by developers. Request handlers can parse request data, validate input, execute business logic, and generate appropriate HTTP responses.
-
- Response Generation:
-
-
- Developers can generate HTTP responses dynamically using the response object provided by HTTP/HTTPS servers. Response objects allow setting response headers, status codes, and response bodies to send data back to clients.
-
- Client Requests:
-
- The HTTP/HTTPS modules enable making HTTP requests to external servers or APIs from Node.js applications. Developers can create HTTP client instances using the http.request() and https.request() methods to send requests, receive responses, and handle data asynchronously.
- Example:
- javascript
- Copy code
const https = require('https'); const options = { hostname: 'www.example.com', port: 443, path: '/', method: 'GET' }; const req = https.request(options, (res) => { console.log('statusCode:', res.statusCode); console.log('headers:', res.headers); res.on('data', (data) => { process.stdout.write(data); }); }); req.end();
- Secure Communication:
-
-
- The HTTPS module extends the functionality of the HTTP module by adding support for SSL/TLS encryption, ensuring secure communication between clients and servers. HTTPS servers use SSL/TLS certificates to encrypt data and verify server identities.
-
- Middleware Integration:
-
-
- HTTP/HTTPS servers in Node.js support middleware integration, allowing developers to use middleware frameworks like Express.js to enhance server functionality, implement routing, handle sessions, and manage request/response pipelines effectively.
-
- WebSocket Support:
-
- HTTP/HTTPS servers can be extended to support WebSocket communication using WebSocket libraries or frameworks. WebSocket connections can be upgraded from HTTP/HTTPS connections to enable real-time bidirectional communication between clients and servers.
The HTTP/HTTPS modules in Node.js serve as fundamental building blocks for creating web servers, handling HTTP requests, making HTTP client requests, and facilitating secure communication over the web.
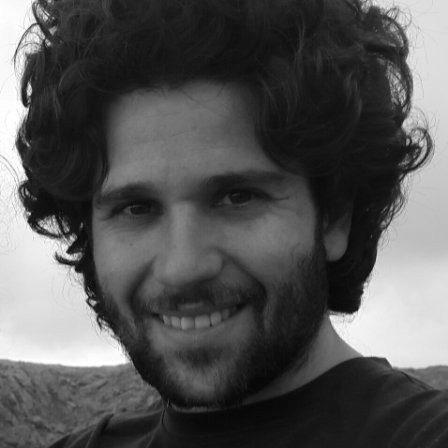
Previously at
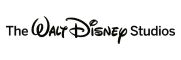
Experienced Principal Engineer and Fullstack Developer with a strong focus on Node.js. Over 5 years of Node.js development experience.