Implementing Web Authentication in Node.js: OAuth, JWT, and more
Understanding Web Authentication
Authentication is the process of verifying the identity of users accessing your application. It involves confirming that users are who they claim to be before granting them access to protected resources. There are several authentication protocols and mechanisms available, each with its own set of advantages and use cases.
OAuth: Delegated Authorization
OAuth is an open standard for delegated authorization, commonly used for enabling third-party applications to access user data without sharing sensitive credentials, such as passwords. It allows users to grant limited access to their resources on one site to another site without exposing their credentials.
Example: Implementing OAuth with Passport.js
Passport.js is a popular authentication middleware for Node.js applications. By integrating Passport.js with OAuth providers like Google, Facebook, or GitHub, you can enable OAuth authentication in your application effortlessly. Check out this guide to learn how to implement OAuth using Passport.js.
JWT (JSON Web Tokens): Stateless Authentication
JWT is a compact, URL-safe means of representing claims to be transferred between two parties. It’s commonly used for stateless authentication, where the server doesn’t need to store session information. JWTs can be securely transmitted as a URL parameter, a POST parameter, or within an HTTP header.
Example: Implementing JWT Authentication in Express.js
Express.js is a popular web application framework for Node.js. You can implement JWT-based authentication in Express.js using middleware libraries like jsonwebtoken and express-jwt. Check out this tutorial for a step-by-step guide on implementing JWT authentication in Express.js.
Session-Based Authentication
Session-based authentication involves storing session information on the server-side and associating a session identifier with each user session. This identifier is then used to authenticate subsequent requests from the same user.
Example: Using Express Session Middleware
Express-session is a popular middleware for session-based authentication in Express.js applications. It provides a simple and flexible API for managing sessions. Check out this documentation to learn how to integrate express-session into your Node.js application.
Conclusion
Implementing web authentication in Node.js requires careful consideration of your application’s requirements, security concerns, and user experience. Whether you choose OAuth, JWT, or session-based authentication, understanding the underlying principles and best practices is essential for building secure and user-friendly applications.
By leveraging the power of frameworks like Passport.js and middleware libraries like express-session, you can streamline the authentication process and focus on delivering value to your users.
Remember to stay updated on the latest security trends and vulnerabilities to ensure that your authentication mechanisms remain robust and resilient against potential threats.
Additional Resources:
Table of Contents
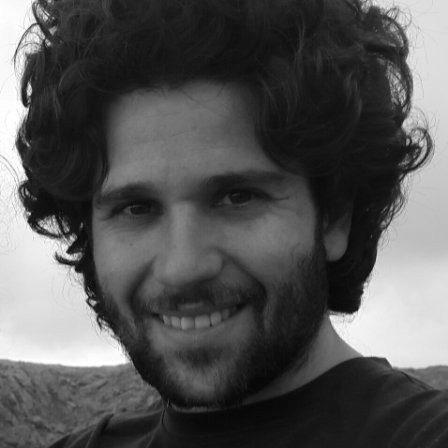
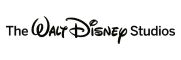