Improving Web Development: A Comprehensive Guide to Building Web Applications with Node.js and Express.js
When it comes to developing web applications, there’s a world of options and technologies available. Today, we are going to focus on a popular duo in web development: Node.js and Express. Both are tools that, when used together, can build robust and scalable applications with relative ease. In this blog post, we will explore how Node.js and Express can be used in web development, and we’ll walk through examples that show these tools in action. If you’re looking to build a web application using Node.js and Express, it might be worth considering hiring Node.js developers. These professionals are well-versed in leveraging the power of Node.js and Express, ensuring efficient development and delivering high-quality, feature-rich applications.
Introduction to Node.js
Node.js is a runtime environment that allows developers to execute JavaScript outside of a web browser. This makes it possible to use JavaScript for server-side scripting, thus creating dynamic web pages before sending the page to the user’s web browser. Essentially, it allows JavaScript to be used for more than just making websites interactive.
Node.js is built on Chrome’s V8 JavaScript engine, and it uses an event-driven, non-blocking I/O model that makes it lightweight and efficient, perfect for data-intensive real-time applications that run across distributed devices.
What is Express.js?
Express.js, or simply Express, is a minimalist web application framework for Node.js. Express simplifies the process of building web applications by providing a simple API for creating different types of HTTP requests, configuring middleware, setting up routes, and more. It’s part of the MEAN (MongoDB, Express.js, Angular.js, Node.js) and MERN (MongoDB, Express.js, React.js, Node.js) software stacks, which are full-stack JavaScript frameworks.
Building a Simple Web App with Node.js and Express
To illustrate how to use Node.js and Express, we’ll create a simple web application. This application will serve a static webpage and respond to HTTP GET and POST requests.
1. Setting Up Your Environment
Before we start, make sure you have Node.js and npm (node package manager) installed. If not, you can download and install Node.js from the official website, which will automatically install npm.
Now, let’s create a new directory for our project, initialize a new Node.js project, and install Express. Open a terminal or command prompt, then run the following commands:
```bash mkdir my-express-app && cd my-express-app npm init -y npm install express ```
2. Creating Your First Express Application
First, create a new file named `app.js` in your project root directory. This file will contain the code for our Express application. Add the following code to `app.js`:
```javascript const express = require('express'); const app = express(); const port = 3000; app.get('/', (req, res) => { res.send('Hello, World!'); }); app.listen(port, () => { console.log(`Server listening at http://localhost:${port}`); }); ```
In this script, we first require the Express module, then create an instance of an Express application. We define a basic route handler for the homepage (‘/’) that sends ‘Hello, World!’ to the client. Lastly, we set the application to listen on port 3000.
You can start the server with this command:
```bash node app.js ```
If you navigate to `http://localhost:3000` in your web browser, you should see ‘Hello, World!’ displayed.
3. Serving Static Files
Express makes serving static files like images, CSS files, and JavaScript files easy with the built-in middleware function, `express.static`. Let’s create a `public` directory in the root of our project and place an `index.html` file inside:
```html <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>My Express App</title> </head> <body> <h1>Welcome to My Express App!</h1> </body> </html> ```
Now, modify your `app.js` to use `express.static`:
```javascript const express = require('express'); const app = express(); const port = 3000; app.use(express.static('public')); app.listen(port, () => { console.log(`Server listening at http://localhost:${port}`); }); ```
Now, if you navigate to `http://localhost:3000` in your web browser, you should see your `index.html` file served.
4. Handling POST Requests
Let’s add functionality to handle POST requests. To parse the body of the POST request, we need the `express.json` middleware. Update your `app.js`:
Here, the POST request to ‘/data’ logs the body of the request to the console and sends a response with a 200 status code.
To test this, you can use tools like curl, Postman, or any HTTP client of your choice to send a POST request to `http://localhost:3000/data`.
Conclusion
This post explores the potential of Node.js and Express in web server construction. Their combination allows for user authentication, database integration, and much more, forming a powerful foundation for web applications. However, to leverage the full capability of these tools for complex applications, hiring Node.js developers can be an effective strategy. These experts can streamline the process while ensuring the output is robust and scalable. Regardless of your experience level, Node.js and Express are versatile tools that make web development more efficient, making them a favorite choice among modern Node.js developers.
Table of Contents
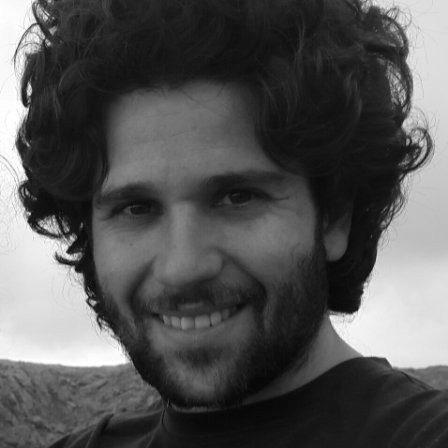
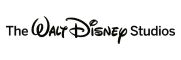