Node.js Q & A
How do you handle input validation in Node.js?
Input validation is essential for ensuring data integrity, preventing security vulnerabilities, and enhancing the reliability of Node.js applications. Here are some best practices for handling input validation in Node.js:
- Use Validation Libraries:
-
-
- Utilize validation libraries such as validator, joi, or express-validator to validate input data against predefined rules and constraints. These libraries provide built-in validation functions for common use cases (e.g., string validation, email validation, URL validation) and support custom validation rules.
-
- Validate Incoming Requests:
-
-
- Validate incoming requests and request parameters (e.g., query parameters, route parameters, request body) before processing them. Use middleware functions or route handlers to perform input validation and reject invalid requests.
-
- Sanitize Input Data:
-
-
- Sanitize input data by removing potentially dangerous characters, escaping special characters, or encoding data to prevent injection attacks (e.g., XSS, SQL injection). Use sanitization libraries like sanitize-html or xss to sanitize user-generated content before rendering it in HTML or executing it in SQL queries.
-
- Use Schema Validation:
-
-
- Define data schemas or validation schemas using JSON Schema or schema validation libraries like joi to specify the structure, data types, and validation rules for input data. Validate input data against the schema to ensure it meets the expected format and constraints.
-
- Handle Errors Gracefully:
-
-
- Handle validation errors gracefully by returning meaningful error messages, status codes, and error responses to clients. Use descriptive error messages to inform users about validation failures and provide guidance on how to correct them.
-
- Validate Server-Side and Client-Side:
-
-
- Implement input validation on both the server-side and client-side to enforce data validation rules consistently and prevent malicious or malformed data from reaching the server. Use client-side validation to provide immediate feedback to users and reduce unnecessary server requests.
-
- Test Input Validation Logic:
-
- Write unit tests and integration tests to validate the input validation logic thoroughly. Test various scenarios, including valid inputs, invalid inputs, edge cases, and boundary conditions, to ensure that the validation rules are enforced correctly and error handling is implemented appropriately.
- Implement Custom Validation Logic:
-
-
- Implement custom validation logic for specific business requirements or complex validation scenarios that cannot be handled by standard validation libraries. Use custom validation functions or middleware to enforce custom validation rules and constraints.
-
- Update Validation Rules Regularly:
-
-
- Regularly review and update validation rules based on changing requirements, security considerations, and feedback from users. Stay informed about common security vulnerabilities (e.g., OWASP Top 10) and emerging threats to improve the effectiveness of input validation.
-
- Log Validation Failures:
-
- Log validation failures and security incidents to monitor and track potential security threats, malicious activities, or data breaches. Use logging frameworks like winston or built-in logging capabilities in Node.js to log validation errors and security events.
By following these best practices, Node.js applications can implement robust input validation mechanisms to ensure data integrity, prevent security vulnerabilities, and enhance the overall reliability and security of the application.
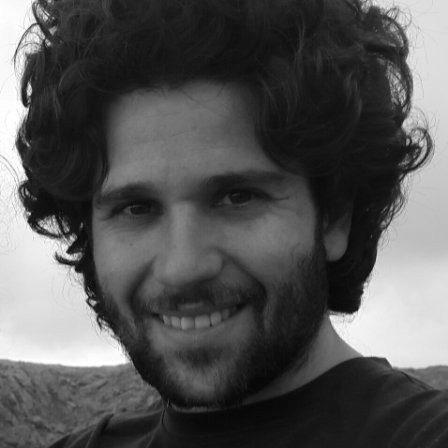
Previously at
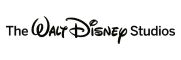
Experienced Principal Engineer and Fullstack Developer with a strong focus on Node.js. Over 5 years of Node.js development experience.