Building Interactive Web Experiences: A Guide to Real-Time Applications with Node.js and Socket.IO
In the current digital landscape, real-time applications are transforming how we interact with the web. These applications allow users to receive information as it happens, resulting in highly responsive and interactive experiences. Two of the key technologies making these real-time applications possible are Node.js and Socket.IO. To fully leverage these technologies, many businesses opt to hire Node.js developers, who possess the skill set to navigate the intricacies of such powerful tools.
Node.js is an open-source, back-end JavaScript runtime environment that runs on the V8 engine and executes JavaScript code outside a web browser. Socket.IO is a JavaScript library that allows for real-time, bi-directional communication between web clients and servers. When combined, they form a powerful toolkit for creating real-time applications. Node.js developers, with their in-depth understanding of these technologies, can create robust, scalable, and real-time solutions that enhance user experience.
This article is a practical guide that will show you how to create a real-time application using these two powerful tools. It will also underscore the value of Node.js developers in shaping and implementing these sophisticated solutions. Let’s begin by understanding why real-time applications matter.
1. The Importance of Real-Time Applications
The hallmark of real-time applications is their ability to push data to clients instantly as soon as the server receives it. Whether it’s a live chat application, online gaming, live tracking apps, or collaborative platforms like Google Docs, real-time applications enhance user experiences by offering seamless, immediate feedback and interaction.
2. Getting Started with Node.js and Socket.IO
Before we dive into the coding part, ensure that you have Node.js and npm (Node Package Manager) installed on your machine.
To confirm the installation, open your terminal or command prompt and type:
```bash node -v npm -v ```
Each command should return a version number, indicating that Node.js and npm are installed. If they’re not, you can download Node.js [here](https://nodejs.org/), which will also install npm alongside.
2.1 Setting up the Project
To start, create a new directory for the project, navigate into it, and initialize a new Node.js project:
```bash mkdir socket-app cd socket-app npm init -y ```
This will create a `package.json` file in your project directory, which stores information about your project and its dependencies.
Install Express and Socket.IO using npm:
```bash npm install express socket.io ```
Let’s create an `index.js` file which will be the entry point to our application:
```bash touch index.js ```
2.2 Creating a Basic Server with Express
Express is a minimal and flexible Node.js web application framework that provides a robust set of features for web and mobile applications. We’ll use Express to set up our server.
In your `index.js`, add the following:
```javascript const express = require('express'); const http = require('http'); const socketIo = require('socket.io'); const app = express(); const server = http.createServer(app); const io = socketIo(server); app.get('/', (req, res) => { res.send('Welcome to our real-time application!'); }); server.listen(3000, () => { console.log('Server is running on port 3000'); }); ```
In this code, we’re creating a simple server with Express that listens on port 3000. We’re also setting up Socket.IO to work in conjunction with our server. If you run your server now with `node index.js`, and visit `http://localhost:3000`, you’ll see a greeting message.
2.3 Integrating Socket.IO
Socket.IO works by adding event listeners to an instance of `http.Server`. Events are triggered in response to server-side and client-side occurrences. When an event is triggered, a function (known as an event handler) is executed.
Add the following code within your `index.js`:
```javascript io.on('connection', (socket) => { console.log('A user has connected.'); socket.on('disconnect', () => { console.log('A user has disconnected.'); }); }); ```
Now, whenever a client connects or disconnects from our server, a message will be logged in our server console. However, there’s no way for a client to connect yet. To make this possible, we need to create a client-side application.
2.4 Creating a Client-side Application
Let’s create an `index.html` file in a new `public` directory:
```bash mkdir public cd public touch index.html ```
In the `index.html` file, add the following:
```html <!DOCTYPE html> <html> <head> <title>Real-Time App</title> </head> <body> <h1>Welcome to our real-time application!</h1> <script src="/socket.io/socket.io.js"></script> <script> const socket = io(); </script> </body> </html> ```
Here, we’re linking the client-side Socket.IO library using the ‘/socket.io/socket.io.js’ path, which is automatically provided by the server-side Socket.IO we installed earlier. Then, we’re connecting to the server using `io()`.
Back in our `index.js`, we need to have Express serve this HTML file. Above the server listener, add:
```javascript app.use(express.static('public')); ```
Now, when you run your server and visit `http://localhost:3000`, you’ll see the greeting message from the HTML file, and in your server console, you’ll see a log indicating that a user has connected.
3. Building a Real-Time Chat Application
Now, let’s evolve our application to become a basic chat app. We’ll allow users to type in a message and display that message to all connected users in real-time.
3.1 Updating the Server
Update your ‘connection’ event in `index.js`:
```javascript io.on('connection', (socket) => { console.log('A user has connected.'); socket.on('chat message', (msg) => { io.emit('chat message', msg); }); socket.on('disconnect', () => { console.log('A user has disconnected.'); }); }); ```
Here, we’re listening for a ‘chat message’ event, which will be emitted by the client whenever a user sends a chat message. When this event is received, we’re broadcasting it to all connected clients.
3.2 Updating the Client-side Application
Update your `index.html`:
```html <!DOCTYPE html> <html> <head> <title>Real-Time Chat App</title> <style> /* Add some basic styling */ #messages { list-style-type: none; margin: 0; padding: 0; } #messages li { padding: 5px 10px; } #messages li:nth-child(odd) { background: #eee; } </style> </head> <body> <ul id="messages"></ul> <form id="form" action=""> <input id="input" autocomplete="off" /><button>Send</button> </form> <script src="/socket.io/socket.io.js"></script> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> <script> const socket = io(); $('form').submit(function(e) { e.preventDefault(); socket.emit('chat message', $('#input').val()); $('#input').val(''); return false; }); socket.on('chat message', function(msg) { $('#messages').append($('<li>').text(msg)); }); </script> </body> </html> ```
We’re creating a form for users to submit their messages. When the form is submitted, we’re emitting a ‘chat message’ event to the server with the message from the input field.
We’re also listening for ‘chat message’ events from the server. When such an event is received, we’re appending the message to the list of messages.
Now, run your server and open multiple tabs or windows to `http://localhost:3000`. Type a message in one window and see it appear in real-time in all the other windows.
Conclusion
In this guide, we explored how to build real-time applications using Node.js and Socket.IO, with a practical example of a chat application. This not only underlines the power and flexibility of these technologies but also highlights the skills that proficient Node.js developers can bring to your project.
While this tutorial only scratches the surface of what’s possible, seasoned Node.js developers could extend this basic chat application further, incorporating features like user authentication, private messaging, and much more. With real-time applications and the right development expertise, the sky’s the limit!
Note: While Socket.IO is incredibly powerful, it’s important to be mindful of scaling. As your application grows, you may need to explore additional tools and techniques, like using Redis for multi-server communication or integrating with a load balancer. Real-time apps can be complex, and hiring expert Node.js developers can help navigate these challenges, enabling you to create amazing, responsive experiences for your users.
Table of Contents
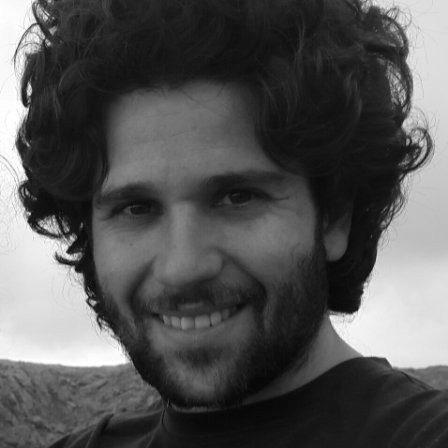
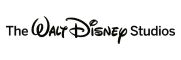