Node.js Q & A
How do you handle long-running processes in Node.js?
Handling long-running processes in Node.js requires careful consideration to ensure optimal performance, resource management, and responsiveness. Here are several approaches to handle long-running processes effectively:
- Use Worker Threads:
-
-
- Node.js provides Worker Threads, which allow developers to execute JavaScript code in parallel across multiple threads. By offloading CPU-intensive tasks to Worker Threads, you can prevent blocking the event loop and improve overall application performance.
-
- Optimize Algorithms and Data Structures:
-
-
- Review and optimize algorithms and data structures used in long-running processes to reduce their computational complexity and memory usage. Consider using efficient algorithms and data structures to improve performance and scalability.
-
- Implement Asynchronous Operations:
-
-
- Utilize asynchronous programming techniques such as Promises, async/await, or callback functions to handle I/O-bound operations efficiently. Asynchronous operations ensure that the event loop remains responsive while waiting for I/O operations to complete.
-
- Implement Timeout and Throttling Mechanisms:
-
-
- Set timeouts and implement throttling mechanisms to limit the execution time and concurrency of long-running processes. Throttling prevents excessive resource consumption and ensures fair allocation of resources.
-
- Scale Horizontally:
-
-
- Distribute long-running processes across multiple instances or servers to scale horizontally and handle increased workload effectively. Load balancing techniques such as round-robin, least connections, or IP hash can help distribute traffic evenly.
-
- Monitor and Optimize Resource Usage:
-
-
- Monitor CPU, memory, and other resource usage metrics to identify bottlenecks and optimize resource utilization. Use profiling tools like Node.js Profiler or CPU profilers to analyze CPU usage and identify performancehotspots.
-
- Implement Graceful Shutdowns:
-
-
- Implement graceful shutdown mechanisms to handle unexpected errors, interruptions, or termination signals gracefully. Close database connections, release resources, and perform cleanup tasks to ensure data integrity and application stability.
-
- Use Caching and Memoization:
-
- Cache frequently accessed data or results of expensive computations to reduce redundant work and improve response times. Memoization techniques can help cache function results and avoid recomputation.
By applying these strategies and best practices, Node.js applications can effectively handle long-running processes while maintaining responsiveness, scalability, and reliability.
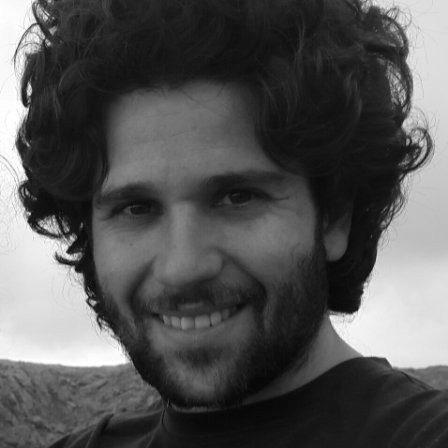
Previously at
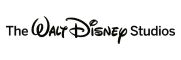
Experienced Principal Engineer and Fullstack Developer with a strong focus on Node.js. Over 5 years of Node.js development experience.