Building Microservices with Node.js and NATS
Microservices architecture is a powerful approach to building scalable, maintainable, and resilient applications. With Node.js as a lightweight, event-driven runtime, and NATS as a high-performance messaging system, you can efficiently connect and manage microservices in a distributed environment. This article explores how to build microservices with Node.js and NATS, covering key concepts, architecture patterns, and practical code examples.
Understanding Microservices Architecture
Microservices is an architectural style that structures an application as a collection of loosely coupled services, each responsible for a specific functionality. This approach allows for independent deployment, scalability, and maintainability. Node.js, with its non-blocking I/O and efficient event-driven model, is well-suited for developing microservices, while NATS provides fast, lightweight messaging capabilities to connect these services.
Using Node.js and NATS for Microservices
Node.js provides a robust foundation for building microservices, offering scalability and a vast ecosystem of libraries. NATS, on the other hand, enables seamless communication between services, making it an excellent choice for a microservices messaging backbone. Below, we will explore key aspects of building microservices with Node.js and NATS.
1. Setting Up a Basic Microservice with Node.js
The first step in building a microservices architecture is to create individual services that handle specific tasks. Here, we will set up a simple microservice using Express, a popular web framework for Node.js.
Example: Basic Node.js Microservice
```javascript const express = require('express'); const app = express(); const PORT = 3000; app.get('/service1', (req, res) => { res.send('Service 1: Responding to request'); }); app.listen(PORT, () => { console.log(`Service 1 running on port ${PORT}`); }); ```
This example sets up a basic microservice that listens for HTTP requests on a specific route and port.
2. Integrating NATS for Microservices Communication
NATS is a lightweight messaging system that facilitates communication between microservices. It supports pub/sub, request/reply, and other messaging patterns, making it ideal for microservices architecture.
Example: Connecting a Microservice to NATS
```javascript const NATS = require('nats'); const nats = NATS.connect({ url: 'nats://localhost:4222' }); nats.subscribe('service1.request', (msg) => { console.log(`Received request: ${msg}`); nats.publish('service1.response', 'Service 1: Responding to NATS request'); }); console.log('Service 1 connected to NATS'); ```
This example shows how a microservice can subscribe to a NATS subject (`service1.request`) and respond to messages by publishing a response on another subject (`service1.response`).
3. Implementing Asynchronous Communication
One of the key benefits of using NATS is its support for asynchronous messaging, which enables services to communicate efficiently without blocking operations.
Example: Asynchronous Request-Reply Pattern with NATS
```javascript const NATS = require('nats'); const nats = NATS.connect({ url: 'nats://localhost:4222' }); // Service 1: Requester nats.request('service2.request', 'Hello from Service 1', (response) => { console.log(`Service 1 received response: ${response}`); }); // Service 2: Responder nats.subscribe('service2.request', (msg, reply) => { console.log(`Service 2 received message: ${msg}`); nats.publish(reply, 'Hello from Service 2'); }); console.log('Services connected for asynchronous communication'); ```
In this example, Service 1 sends a request to Service 2 and processes the response asynchronously, demonstrating the non-blocking nature of NATS messaging.
4. Scaling Microservices with NATS
NATS excels in scenarios where services need to scale horizontally. By simply adding more instances of a service, NATS can distribute messages among them, ensuring load balancing and fault tolerance.
Example: Scaling with Multiple Service Instances
```javascript const NATS = require('nats'); const nats = NATS.connect({ url: 'nats://localhost:4222' }); nats.subscribe('service.request', (msg) => { console.log(`Service instance handling request: ${msg}`); // Process request... }); // Run multiple instances of this script to simulate scaling ```
Running multiple instances of this script allows NATS to distribute requests among them, demonstrating how easy it is to scale microservices with NATS.
5. Monitoring and Managing Microservices
Monitoring is crucial in a microservices architecture to ensure services are running smoothly. Node.js provides various tools like PM2 for process management, and NATS offers built-in monitoring capabilities.
Example: Monitoring Microservices with PM2
```bash Install PM2 globally npm install -g pm2 Start a microservice with PM2 pm2 start service1.js --name service1 Monitor the service pm2 monit ```
Using PM2, you can monitor, restart, and manage multiple instances of your Node.js microservices easily.
Conclusion
Building microservices with Node.js and NATS offers a scalable, efficient, and robust approach to modern application development. Node.js provides the foundation for creating lightweight services, while NATS enables seamless communication between them. By leveraging these tools, you can create a highly responsive, resilient microservices architecture that meets the demands of today’s distributed systems.
Further Reading:
Table of Contents
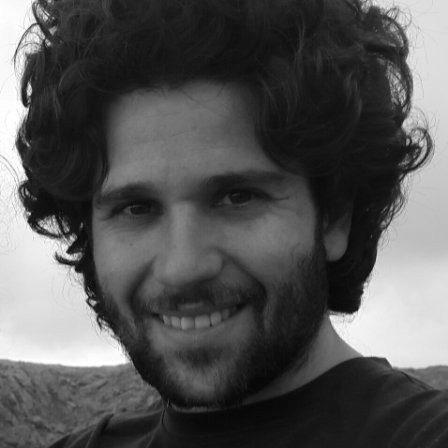
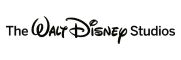