Node.js Q & A
How do you connect to a MongoDB database in Node.js?
To connect to a MongoDB database in Node.js, you can use the official MongoDB Node.js driver or popular libraries such as Mongoose. Here’s how to connect to a MongoDB database using the official MongoDB Node.js driver:
- Install the MongoDB Node.js driver using npm:
Copy code npm install mongodb
- In your Node.js application, import the mongodb module and create a MongoClient instance:
javascript Copy code const { MongoClient } = require('mongodb'); // MongoDB connection URI const uri = 'mongodb://localhost:27017/mydatabase'; // Create a new MongoClient const client = new MongoClient(uri);
- Connect to the MongoDB database using the connect() method:
javascript Copy code async function connectToDatabase() { try { // Connect to the MongoDB server await client.connect(); console.log('Connected to MongoDB'); } catch (error) { console.error('Error connecting to MongoDB:', error); } } connectToDatabase();
Once connected, you can perform database operations such as inserting documents, querying data, updating documents, and deleting documents using the MongoDB Node.js driver’s APIs.
Alternatively, you can use libraries like Mongoose, an ODM (Object-Document Mapper) for MongoDB, which provides a higher-level abstraction and additional features for working with MongoDB databases in Node.js.
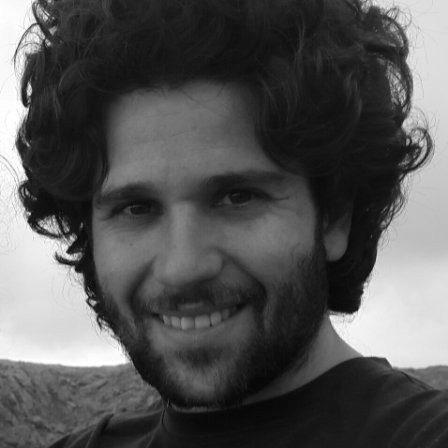
Previously at
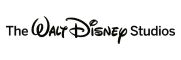
Experienced Principal Engineer and Fullstack Developer with a strong focus on Node.js. Over 5 years of Node.js development experience.