Unleashing Node.js Potential: A Comprehensive Guide to Navigating npm
In the rapidly evolving world of web development, Node.js has emerged as a premier tool in JavaScript’s vanguard, leading many businesses to hire Node.js developers. With the power to run JavaScript server-side, Node.js has paved the way for robust and dynamic applications. To enhance this capacity, Node.js uses the npm (Node Package Manager), an online repository of packages, making it easier for developers, including those Node.js developers for hire, to share and distribute code. npm is a fundamental cog in the Node.js machine, simplifying the development process and enhancing the JavaScript ecosystem.
In this blog post, we will delve into the power of npm, demystifying its capabilities and how we can harness it in our Node.js development. This understanding is key, not just for individual developers, but also for businesses looking to hire Node.js developers. We will explore the installation of packages, scripts, versioning, and a lot more with practical examples. By doing so, we aim to provide a comprehensive guide for those interested in Node.js and those who are looking to hire Node.js developers who can effectively use these tools.
Installing Packages with npm
The primary function of npm is to install packages that developers need for their projects. It’s as simple as specifying the name of the package you need. For instance, to install Express.js, a popular web application framework for Node.js, you use the following command in your terminal:
``` npm install express ```
This command installs the Express.js package into a local `node_modules` folder in your project directory and also adds it to the dependencies in the `package.json` file.
Example:
Create a simple HTTP server using Express.js. Here’s how:
- Initialize a new npm project:
``` npm init -y ```
- Install Express.js:
``` npm install express ```
- Create a new file named `app.js` and add the following code:
```javascript const express = require('express'); const app = express(); const port = 3000; app.get('/', (req, res) => { res.send('Hello World!'); }); app.listen(port, () => { console.log(`Server running at http://localhost:${port}`); }); ```
- Run your server:
``` node app.js ```
Navigate to `http://localhost:3000` in your browser, and you’ll see “Hello World!”.
Managing Scripts with npm
npm is not only a package installer, it’s also a powerful task runner. It can run scripts defined in the `package.json` file. For instance, you can set a script to start your application.
In your `package.json` file, under `scripts`, add:
```json "scripts": { "start": "node app.js" } ```
You can now start your application by running `npm start` in your terminal.
Example:
Let’s enhance our previous Express.js application by adding nodemon to it. Nodemon is a utility that will monitor for any changes in your source and automatically restart your server.
- Install nodemon as a dev dependency:
``` npm install --save-dev nodemon ```
- Add a start script in `package.json`:
```json "scripts": { "start": "nodemon app.js" } ```
- Run your server:
``` npm start ```
With this setup, your server restarts automatically whenever you make changes to `app.js`.
Versioning and Updating with npm
npm helps manage versions of the installed packages. The versioning system used by npm follows Semantic Versioning or SemVer (`MAJOR.MINOR.PATCH`). When installing packages, npm saves the semantic range of the version in `package.json` by default. It allows npm to install updates that do not break your existing code.
To update all packages to the latest version, you can use:
``` npm update ```
To update a specific package:
``` npm update <package-name> ```
Example:
Suppose you have an application using lodash, a JavaScript utility library. The version installed is `4.17.20`.
- Check the latest version of lodash:
``` npm show lodash version ```
- If the latest version is newer than `4.17.20`, update lodash:
``` npm update lodash ```
- Confirm the installed version:
``` npm list lodash ```
Publishing your Package to npm
npm allows you to publish your package and share it with the world. You can publish your packages privately or publicly.
Example:
Let’s say you have created a simple utility function to add two numbers and you want to publish it to npm.
- Initialize a new npm project:
``` npm init -y ```
- Create a new file `index.js` and add your function:
```javascript function add(a, b) { return a + b; } module.exports = add; ```
- Login to npm:
``` npm login ```
Enter your npm account credentials. If you don’t have an account, you can create one using `npm adduser` or through the npm website.
- Publish your package:
``` npm publish ```
Your package is now available in the npm registry and can be installed by anyone using `npm install <your-package-name>`.
Conclusion
From package installation to script management, version control, and publishing, npm simplifies and accelerates the development process. It is an indispensable tool in Node.js development, encapsulating a world of possibilities. This is one reason why businesses are increasingly looking to hire Node.js developers who are skilled in navigating the npm ecosystem.
The beauty of npm lies in its vast, open-source ecosystem, where sharing is the norm, and everyone benefits. By harnessing the power of npm, developers – including Node.js developers for hire – can stand on the shoulders of giants, leveraging the efforts of others to focus on creating something unique and innovative.
npm truly personifies the spirit of collaboration and creativity in the Node.js community. As you venture into your next Node.js project, or when you hire Node.js developers, remember the power you wield with npm at your fingertips. With every package installed, every script run, and perhaps one day, with every package published, you are contributing to the thriving Node.js ecosystem. Whether you’re an individual developer or an organization looking to hire Node.js developers, let’s continue to harness the power of npm in our Node.js development journey!
Table of Contents
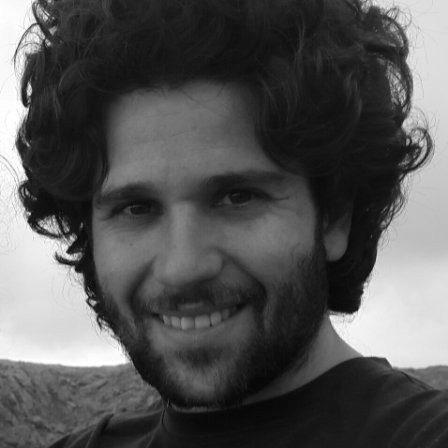
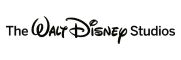