Implementing OAuth 2.0 in Node.js Applications
OAuth 2.0 is a robust framework for authorization that enables applications to access user data without exposing user credentials. By implementing OAuth 2.0 in Node.js applications, developers can create secure and scalable authentication systems. This guide will explore how to integrate OAuth 2.0 into your Node.js applications and provide practical examples to help you get started.
Understanding OAuth 2.0
OAuth 2.0 is an authorization framework that allows third-party applications to obtain limited access to user resources on a server. It is widely used for authorizing access to APIs and managing user permissions securely.
Setting Up OAuth 2.0 in a Node.js Application
To implement OAuth 2.0 in a Node.js application, you’ll typically need to handle user authentication, access token management, and integration with OAuth providers. Below are the key steps and code examples to guide you through the process.
1. Installing Required Packages
To get started, you need to install several packages to handle OAuth 2.0 in your Node.js application. These include `express` for building the web server and `passport` along with `passport-oauth2` for managing OAuth 2.0 authentication.
```bash npm install express passport passport-oauth2 ```
2. Configuring Passport for OAuth 2.0
Passport.js is a popular authentication middleware for Node.js. You’ll need to configure it to use the OAuth 2.0 strategy.
Example: Configuring Passport with OAuth 2.0
```javascript const express = require('express'); const passport = require('passport'); const OAuth2Strategy = require('passport-oauth2'); const session = require('express-session'); const app = express(); passport.use(new OAuth2Strategy({ authorizationURL: 'https://provider.com/oauth2/authorize', tokenURL: 'https://provider.com/oauth2/token', clientID: 'your-client-id', clientSecret: 'your-client-secret', callbackURL: 'http://localhost:3000/auth/callback' }, (accessToken, refreshToken, profile, cb) => { // Use the profile and tokens to authenticate the user return cb(null, profile); } )); passport.serializeUser((user, done) => { done(null, user); }); passport.deserializeUser((obj, done) => { done(null, obj); }); app.use(session({ secret: 'secret', resave: false, saveUninitialized: true })); app.use(passport.initialize()); app.use(passport.session()); // Routes will be defined here app.listen(3000, () => { console.log('Server is running on http://localhost:3000'); }); ```
3. Implementing Authentication Routes
Create routes to handle the OAuth 2.0 authorization and callback process.
Example: Authentication Routes
```javascript app.get('/auth', passport.authenticate('oauth2')); app.get('/auth/callback', passport.authenticate('oauth2', { failureRedirect: '/' }), (req, res) => { // Successful authentication res.redirect('/'); }); app.get('/', (req, res) => { if (req.isAuthenticated()) { res.send(`Hello ${req.user.displayName}`); } else { res.send('<a href="/auth">Login with OAuth Provider</a>'); } }); ```
4. Handling Access Tokens
To access protected resources, you’ll need to manage the access tokens received from the OAuth provider. Ensure that tokens are securely stored and used.
Example: Fetching Protected Resources
```javascript const axios = require('axios'); app.get('/profile', async (req, res) => { if (req.isAuthenticated()) { try { const response = await axios.get('https://provider.com/api/profile', { headers: { 'Authorization': `Bearer ${req.user.accessToken}` } }); res.json(response.data); } catch (error) { res.status(500).send('Error fetching profile'); } } else { res.redirect('/auth'); } }); ```
Conclusion
Implementing OAuth 2.0 in Node.js applications enhances security by managing user authentication and authorization effectively. With the steps and examples provided, you can integrate OAuth 2.0 into your applications and ensure secure access to user data.
Further Reading:
Table of Contents
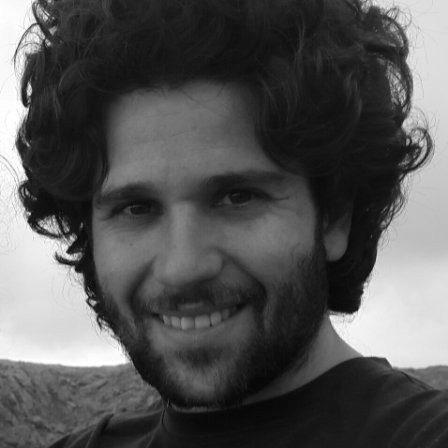
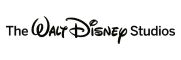