Optimizing Database Performance in Node.js Applications
In the world of Node.js application development, optimizing database performance is a crucial aspect that can significantly impact the overall efficiency and scalability of your application. Whether you’re building a small-scale project or a large-scale enterprise application, ensuring that your database operations are fast and efficient is essential for delivering a seamless user experience. In this article, we’ll explore some best practices and techniques for optimizing database performance in Node.js applications, along with real-world examples to illustrate each concept.
1. Choose the Right Database
The first step in optimizing database performance is choosing the right database for your application’s specific requirements. Different databases excel in different use cases, so it’s essential to evaluate your needs carefully before making a decision. Some popular databases for Node.js applications include:
- MongoDB: A NoSQL database known for its flexibility and scalability, making it suitable for applications with rapidly changing data schemas. MongoDB
- PostgreSQL: A powerful relational database known for its robust feature set and support for complex queries, making it suitable for data-intensive applications. PostgreSQL
- Redis: A high-performance in-memory data store that excels at caching and real-time analytics, making it suitable for applications that require low-latency data access. Redis
2. Use Indexing Wisely
Indexing plays a crucial role in improving query performance by allowing the database to quickly locate the relevant data. When designing your database schema, consider which fields will be frequently queried and create indexes accordingly. However, be cautious not to over-index, as this can lead to increased storage overhead and slower write performance. Here’s an example of creating an index in MongoDB:
db.collection.createIndex({ fieldName: 1 });
3. Implement Pagination
When dealing with large datasets, fetching all records at once can lead to performance issues and increased memory consumption. Implementing pagination allows you to retrieve data in smaller, manageable chunks, reducing the load on the database server and improving overall performance. Here’s an example of implementing pagination in a Node.js application using Mongoose (MongoDB):
const page = parseInt(req.query.page) || 1; const limit = parseInt(req.query.limit) || 10; const results = await Model.find() .skip((page - 1) * limit) .limit(limit);
4. Use Connection Pooling
Connection pooling helps reduce the overhead of establishing and tearing down database connections by reusing existing connections from a pool. This can significantly improve performance, especially in applications with a high volume of database requests. Most database drivers for Node.js support connection pooling out of the box, making it easy to implement. Here’s an example of using connection pooling with the pg library for PostgreSQL:
const { Pool } = require('pg'); const pool = new Pool({ connectionString: 'postgresql://user:password@localhost/database', max: 20, // maximum number of clients in the pool idleTimeoutMillis: 30000, // how long a client is allowed to remain idle before being closed });
Conclusion
Optimizing database performance is essential for ensuring the scalability and responsiveness of your Node.js applications. By choosing the right database, using indexing wisely, implementing pagination, and leveraging connection pooling, you can significantly improve the efficiency of your database operations and deliver a better user experience.
Remember that the examples provided in this article are just a starting point, and there are many other techniques and tools available for optimizing database performance in Node.js applications. Experiment with different approaches and monitor the performance of your application to identify areas for further optimization.
Happy coding!
External Links:
Table of Contents
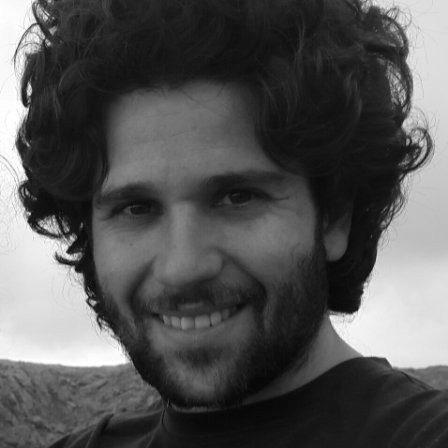
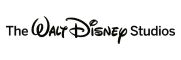