How do you implement pagination in Node.js?
Pagination is a common technique used in web applications to split large datasets into smaller chunks or pages, allowing users to navigate through the data incrementally. In Node.js, pagination can be implemented using various approaches, including:
- Limit and Offset:
-
-
- Use the limit and offset parameters in database queries to fetch a specific number of records (limit) starting from a given offset. Calculate the appropriate offset based on the current page number and page size to fetch data for pagination.
-
- Skip and Limit:
-
-
- Use the skip and limit methods provided by MongoDB or Mongoose to skip a certain number of records and limit the number of records returned in a query. Combine these methods to implement pagination for MongoDB databases.
-
- Cursor-based Pagination:
-
-
- Implement cursor-based pagination using a cursor or token representing the position of the last fetched record. Use this cursor to fetch the next set of records from the database, ensuring efficient pagination for large datasets without relying on offsets.
-
- Page Number and Page Size:
-
-
- Allow users to specify the page number and page size as query parameters in API requests. Use these parameters to calculate the appropriate offset and limit for fetching data from the database.
-
- Pagination Middleware:
-
-
- Implement pagination middleware in Express.js to handle pagination logic centrally for multiple routes or endpoints. Parse request parameters, calculate pagination parameters, and inject pagination metadata into the response for client consumption.
-
- Response Formatting:
-
-
- Format the paginated data in the response body, including the current page number, total number of pages, total number of records, and links to navigate to the previous and next pages.
-
- Client-Side Pagination:
-
-
- Implement client-side pagination using JavaScript frameworks/libraries like React, Vue.js, or Angular. Fetch paginated data from the server via API requests and render it dynamically on the client-side.
-
- Caching and Optimization:
-
- Implement caching mechanisms to cache paginated data or optimize database queries using indexes, query optimization techniques, or data denormalization to improve pagination performance for large datasets.
Here’s a simplified example demonstrating how to implement pagination in an Express.js application using MongoDB and Mongoose:
javascript
Copy code
const express = require('express'); const router = express.Router(); const mongoose = require('mongoose'); const { Product } = require('../models'); // GET route for fetching paginated products router.get('/products', async (req, res) => { const page = parseInt(req.query.page) || 1; // Current page number const pageSize = parseInt(req.query.pageSize) || 10; // Number of products per page try { // Calculate offset based on page number and page size const offset = (page - 1) * pageSize; // Fetch paginated products from the database const products = await Product.find() .skip(offset) .limit(pageSize); // Count total number of products const totalProducts = await Product.countDocuments(); // Calculate total number of pages const totalPages = Math.ceil(totalProducts / pageSize); // Prepare pagination metadata const pagination = { currentPage: page, pageSize: pageSize, totalProducts: totalProducts, totalPages: totalPages }; // Send paginated products and pagination metadata in the response res.json({ products, pagination }); } catch (err) { // Handle errors res.status(500).json({ message: 'Internal Server Error' }); } }); module.exports = router;
In this example, the /products route fetches paginated products from the database based on the requested page number and page size. It calculates the offset and limit for the MongoDB query to fetch the appropriate subset of records for the current page. Finally, it sends the paginated products and pagination metadata (current page, total pages, total products) in the response JSON.
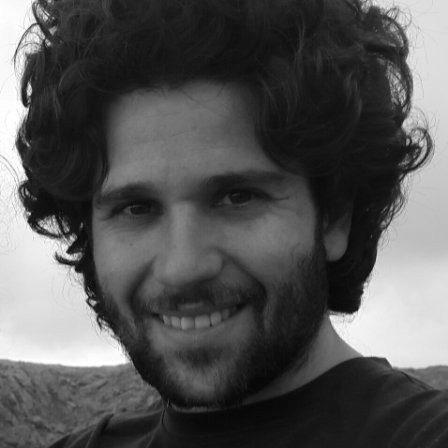
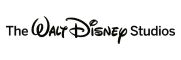