Implementing Pagination and Filtering in Node.js APIs
When building RESTful APIs, especially those that return large datasets, implementing pagination and filtering is essential. These features improve the performance of your API and enhance the user experience by allowing clients to retrieve only the data they need. This article will guide you through the process of adding pagination and filtering to your Node.js APIs with practical code examples.
Understanding Pagination and Filtering
Pagination is the process of dividing a large dataset into smaller, manageable chunks or pages. This prevents overwhelming the client with too much data at once and reduces server load.
Filtering allows users to refine their search by applying specific criteria, ensuring they receive only the data relevant to them.
1. Setting Up a Basic Node.js API
First, let’s set up a basic Node.js API using Express.
```javascript const express = require('express'); const app = express(); const PORT = process.env.PORT || 3000; const data = [ { id: 1, name: 'Item 1', category: 'A' }, { id: 2, name: 'Item 2', category: 'B' }, { id: 3, name: 'Item 3', category: 'A' }, // Add more items to simulate a larger dataset ]; app.get('/items', (req, res) => { res.json(data); }); app.listen(PORT, () => console.log(`Server running on port ${PORT}`)); ```
2. Implementing Pagination
Pagination involves splitting the data into pages and allowing the client to request specific pages. You can implement this by using query parameters such as `page` and `limit`.
```javascript app.get('/items', (req, res) => { const { page = 1, limit = 10 } = req.query; const startIndex = (page - 1) * limit; const endIndex = page * limit; const paginatedResults = data.slice(startIndex, endIndex); res.json(paginatedResults); }); ```
3. Adding Filtering
Filtering can be implemented by allowing the client to pass query parameters that specify the criteria they’re interested in. For instance, you might filter items by category.
```javascript app.get('/items', (req, res) => { const { page = 1, limit = 10, category } = req.query; let results = data; if (category) { results = results.filter(item => item.category === category); } const startIndex = (page - 1) * limit; const endIndex = page * limit; const paginatedResults = results.slice(startIndex, endIndex); res.json(paginatedResults); }); ```
4. Handling Edge Cases
It’s essential to handle scenarios where the client requests a page or limit that doesn’t exist or is out of range.
```javascript app.get('/items', (req, res) => { const { page = 1, limit = 10, category } = req.query; let results = data; if (category) { results = results.filter(item => item.category === category); } const startIndex = (page - 1) * limit; const endIndex = page * limit; const paginatedResults = results.slice(startIndex, endIndex); const totalItems = results.length; const totalPages = Math.ceil(totalItems / limit); if (page > totalPages) { return res.status(404).json({ message: "Page not found" }); } res.json({ page, limit, totalItems, totalPages, data: paginatedResults, }); }); ```
Conclusion
Implementing pagination and filtering in your Node.js APIs is crucial for managing large datasets efficiently. It enhances the user experience by allowing them to request only the data they need and helps reduce server load. By following the steps outlined in this article, you can ensure your API is both performant and user-friendly.
Further Reading:
Table of Contents
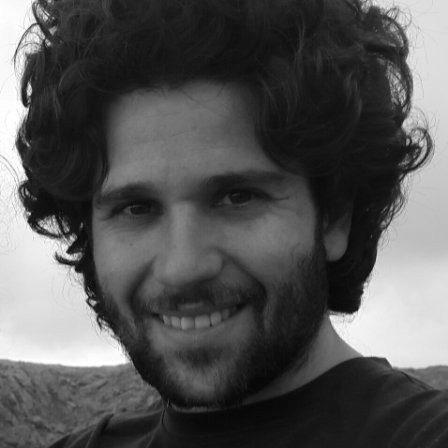
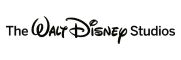