Node.js Q & A
How do you handle query parameters in Node.js?
Query parameters are commonly used in HTTP requests to pass data to the server as part of the URL. In Node.js, handling query parameters involves extracting and parsing query strings from request URLs to access parameter values and process them accordingly. Here’s how you can handle query parameters in Node.js:
- Using the URL Module:
-
-
- Parse query parameters from request URLs using the built-in URL module in Node.js. The URL module provides the URLSearchParams class for working with query strings and extracting parameter values
- Example:
-
- javascript
- Copy code
const { URLSearchParams } = require('url');
const url = require('url');
// Extract query parameters from request URL
const requestUrl = 'http://example.com/api/data?param1=value1¶m2=value2';
const parsedUrl = new URL(requestUrl);
const queryParams = parsedUrl.searchParams;
// Access individual query parameters
const param1Value = queryParams.get('param1');
const param2
Value = queryParams.get('param2');
const { URLSearchParams } = require('url');
const url = require('url');
// Extract query parameters from request URL
const requestUrl = 'http://example.com/api/data?param1=value1¶m2=value2';
const parsedUrl = new URL(requestUrl);
const queryParams = parsedUrl.searchParams;
// Access individual query parameters
const param1Value = queryParams.get('param1');
const param2
Value = queryParams.get('param2');
const { URLSearchParams } = require('url'); const url = require('url'); // Extract query parameters from request URL const requestUrl = 'http://example.com/api/data?param1=value1¶m2=value2'; const parsedUrl = new URL(requestUrl); const queryParams = parsedUrl.searchParams; // Access individual query parameters const param1Value = queryParams.get('param1'); const param2 Value = queryParams.get('param2');
- Using Express.js:
-
-
- If you’re using Express.js, query parameters are automatically parsed and made available through the req.query object. Express parses the URL-encoded query string from the req.url property and populates req.query with an object containing the parsed query parameters.
- Example:
-
- javascript
- Copy code
const express = require('express');
const app = express();
// Route handler to handle requests with query parameters
app.get('/api/data', (req, res) => {
const param1Value = req.query.param1;
const param2Value = req.query.param2;
res.json({ param1: param1Value, param2: param2Value });
});
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
const express = require('express');
const app = express();
// Route handler to handle requests with query parameters
app.get('/api/data', (req, res) => {
const param1Value = req.query.param1;
const param2Value = req.query.param2;
res.json({ param1: param1Value, param2: param2Value });
});
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
const express = require('express'); const app = express(); // Route handler to handle requests with query parameters app.get('/api/data', (req, res) => { const param1Value = req.query.param1; const param2Value = req.query.param2; res.json({ param1: param1Value, param2: param2Value }); }); app.listen(3000, () => { console.log('Server is running on port 3000'); });
- Parsing Query Strings Manually:
-
-
- In vanilla Node.js HTTP servers, you can manually parse query parameters from the request URL using the querystring module. This module provides utility functions for parsing and formatting URL query strings.
- Example:
-
- javascript
- Copy code
const http = require('http');
const querystring = require('querystring');
const server = http.createServer((req, res) => {
const queryString = req.url.split('?')[1];
const queryParams = querystring.parse(queryString);
const param1Value = queryParams.param1;
const param2Value = queryParams.param2;
res.end(JSON.stringify({ param1: param1Value, param2: param2Value }));
});
server.listen(3000, () => {
console.log('Server is running on port 3000');
});
const http = require('http');
const querystring = require('querystring');
const server = http.createServer((req, res) => {
const queryString = req.url.split('?')[1];
const queryParams = querystring.parse(queryString);
const param1Value = queryParams.param1;
const param2Value = queryParams.param2;
res.end(JSON.stringify({ param1: param1Value, param2: param2Value }));
});
server.listen(3000, () => {
console.log('Server is running on port 3000');
});
const http = require('http'); const querystring = require('querystring'); const server = http.createServer((req, res) => { const queryString = req.url.split('?')[1]; const queryParams = querystring.parse(queryString); const param1Value = queryParams.param1; const param2Value = queryParams.param2; res.end(JSON.stringify({ param1: param1Value, param2: param2Value })); }); server.listen(3000, () => { console.log('Server is running on port 3000'); });
- Handling Optional Parameters:
-
-
- Handle optional query parameters gracefully by checking if they exist before accessing their values. Use default values or fallbacks for optional parameters that are not provided in the request URL.
- Example:
-
- javascript
- Copy code
const param1Value = req.query.param1 || 'default';
const param2Value = req.query.param2 || null;
const param1Value = req.query.param1 || 'default';
const param2Value = req.query.param2 || null;
const param1Value = req.query.param1 || 'default'; const param2Value = req.query.param2 || null;
- Sanitizing and Validating Parameters:
-
-
- Validate and sanitize query parameters to ensure data integrity, prevent injection attacks, and handle unexpected input gracefully. Use validation libraries or custom validation logic to sanitize and validate parameter values before processing them.
- Example:
-
- javascript
- Copy code
const { sanitizeQuery } = require('express-validator');
app.get('/api/data', sanitizeQuery(['param1', 'param2']), (req, res) => {
// Process sanitized query parameters
});
const { sanitizeQuery } = require('express-validator');
app.get('/api/data', sanitizeQuery(['param1', 'param2']), (req, res) => {
// Process sanitized query parameters
});
const { sanitizeQuery } = require('express-validator'); app.get('/api/data', sanitizeQuery(['param1', 'param2']), (req, res) => { // Process sanitized query parameters });
By handling query parameters effectively in Node.js applications, you can extract parameter values from request URLs, process them securely, and use them to customize request handling, data retrieval, and response generation based on client requirements.
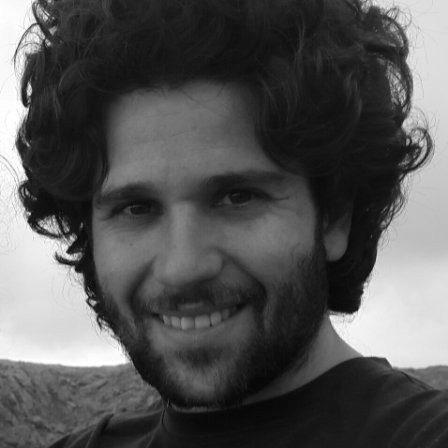
Previously at
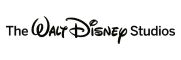
Experienced Principal Engineer and Fullstack Developer with a strong focus on Node.js. Over 5 years of Node.js development experience.