Building Real-Time Multiplayer Games with Node.js and Phaser
Building real-time multiplayer games presents unique challenges, from handling multiple connections to ensuring smooth gameplay. Node.js, with its non-blocking I/O and event-driven architecture, combined with Phaser, a powerful HTML5 game framework, provides a robust solution for creating engaging multiplayer games. This article explores how Node.js and Phaser can be used together to develop real-time multiplayer games, offering practical examples and insights into the development process.
Understanding Real-Time Multiplayer Game Development
Real-time multiplayer games require seamless communication between players and servers to maintain game state consistency. Efficient handling of player actions, real-time updates, and synchronization across different clients are key aspects of developing such games.
Using Node.js for Real-Time Communication
Node.js excels in scenarios that require real-time communication due to its event-driven nature and support for WebSocket protocols. WebSocket enables two-way communication between the client and server, making it ideal for real-time interactions in games.
Example: Setting Up a Basic WebSocket Server
Here’s a basic example of how to set up a WebSocket server using Node.js and the `ws` library:
```javascript const WebSocket = require('ws'); const wss = new WebSocket.Server({ port: 8080 }); wss.on('connection', (ws) => { console.log('Client connected'); ws.on('message', (message) => { console.log(`Received message => ${message}`); ws.send(`Server received: ${message}`); }); ws.on('close', () => { console.log('Client disconnected'); }); }); console.log('WebSocket server is running on ws://localhost:8080'); ```
Using Phaser for Game Development
Phaser is a versatile framework for building 2D games using JavaScript. It provides tools for creating game scenes, handling animations, and managing game state.
Example: Creating a Basic Phaser Game
Below is a simple Phaser game setup where a player can move around a game world:
```html <!DOCTYPE html> <html> <head> <title>Phaser Game</title> <script src="https://cdn.jsdelivr.net/npm/phaser@3/dist/phaser.js"></script> </head> <body> <script> var config = { type: Phaser.AUTO, width: 800, height: 600, physics: { default: 'arcade', arcade: { gravity: { y: 300 }, debug: false } }, scene: { preload: preload, create: create, update: update } }; var player; var cursors; var game = new Phaser.Game(config); function preload() { this.load.image('sky', 'https://labs.phaser.io/assets/skies/space3.png'); this.load.image('ground', 'https://labs.phaser.io/assets/sprites/platform.png'); this.load.image('star', 'https://labs.phaser.io/assets/sprites/star.png'); this.load.spritesheet('dude', 'https://labs.phaser.io/assets/sprites/dude.png', { frameWidth: 32, frameHeight: 48 }); } function create() { this.add.image(400, 300, 'sky'); var platforms = this.physics.add.staticGroup(); platforms.create(400, 568, 'ground').setScale(2).refreshBody(); player = this.physics.add.sprite(100, 450, 'dude'); player.setBounce(0.2); player.setCollideWorldBounds(true); this.physics.add.collider(player, platforms); cursors = this.input.keyboard.createCursorKeys(); } function update() { if (cursors.left.isDown) { player.setVelocityX(-160); } else if (cursors.right.isDown) { player.setVelocityX(160); } else { player.setVelocityX(0); } if (cursors.up.isDown && player.body.touching.down) { player.setVelocityY(-330); } } </script> </body> </html> ```
Integrating Node.js and Phaser for Multiplayer
To create a real-time multiplayer experience, you’ll need to synchronize the game state across multiple clients. This involves sending player actions and game state updates between the client and server.
Example: Syncing Player Movement
Below is a simplified example showing how to sync player movement between clients:
Server (Node.js with WebSocket):
```javascript const WebSocket = require('ws'); const wss = new WebSocket.Server({ port: 8080 }); let players = {}; wss.on('connection', (ws) => { let playerId = Math.random().toString(36).substring(7); players[playerId] = { x: 0, y: 0 }; ws.on('message', (message) => { let data = JSON.parse(message); if (data.type === 'MOVE') { players[playerId] = { x: data.x, y: data.y }; broadcast(JSON.stringify({ type: 'UPDATE', players })); } }); ws.on('close', () => { delete players[playerId]; }); }); function broadcast(message) { wss.clients.forEach((client) => { if (client.readyState === WebSocket.OPEN) { client.send(message); } }); } console.log('WebSocket server is running on ws://localhost:8080'); ```
Client (Phaser):
```javascript // Connect to WebSocket server const socket = new WebSocket('ws://localhost:8080'); // Send player movement data function sendMovement(x, y) { socket.send(JSON.stringify({ type: 'MOVE', x, y })); } // Handle server messages socket.onmessage = (event) => { let data = JSON.parse(event.data); if (data.type === 'UPDATE') { // Update game state with received player data } }; // Update player position and send to server function update() { if (cursors.left.isDown) { player.setVelocityX(-160); sendMovement(player.x - 160, player.y); } else if (cursors.right.isDown) { player.setVelocityX(160); sendMovement(player.x + 160, player.y); } else { player.setVelocityX(0); } if (cursors.up.isDown && player.body.touching.down) { player.setVelocityY(-330); sendMovement(player.x, player.y - 330); } } ```
Conclusion
Node.js and Phaser provide a powerful combination for building real-time multiplayer games. Node.js handles the server-side real-time communication, while Phaser manages the client-side game development. By integrating these technologies, you can create engaging and dynamic multiplayer experiences that offer smooth gameplay and real-time interactions.
Further Reading:
Table of Contents
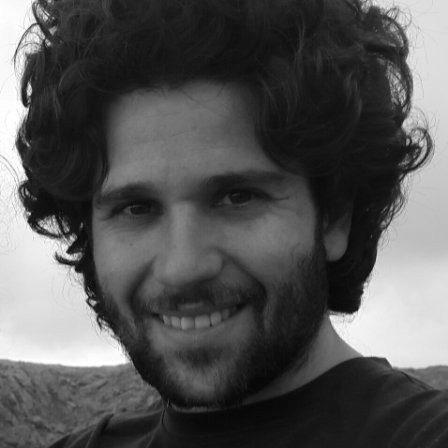
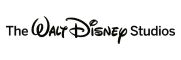