Implementing Real-Time Notifications with Node.js and Socket.IO
In today’s fast-paced digital landscape, users expect real-time updates and notifications from the applications they use. Whether it’s receiving instant messages, updates on social media interactions, or live data feeds, real-time functionality has become a cornerstone of modern app development. Fortunately, with technologies like Node.js and Socket.IO, implementing real-time notifications has never been more accessible.
Understanding the Basics: Node.js and Socket.IO
Node.js, an open-source, cross-platform JavaScript runtime environment, has gained immense popularity among developers for building scalable and high-performance applications. Its event-driven architecture and non-blocking I/O operations make it particularly well-suited for real-time applications.
Socket.IO, a WebSocket library for Node.js, enables real-time, bidirectional communication between clients and servers. It simplifies the implementation of WebSocket-based applications by providing a powerful yet straightforward API.
Getting Started with Node.js and Socket.IO
To begin implementing real-time notifications in your Node.js application, you’ll first need to install Node.js and initialize your project. Once your project is set up, you can install Socket.IO using npm:
npm install socket.io
Next, create a server instance and integrate Socket.IO into your application:
const express = require('express'); const http = require('http'); const socketIo = require('socket.io'); const app = express(); const server = http.createServer(app); const io = socketIo(server); io.on('connection', (socket) => { console.log('A client has connected'); // Handle events socket.on('disconnect', () => { console.log('A client has disconnected'); }); }); server.listen(3000, () => { console.log('Server is running on port 3000'); });
Broadcasting Real-Time Notifications
With Socket.IO, broadcasting real-time notifications to connected clients is straightforward. You can emit custom events from the server and listen for them on the client side. For example, to notify clients of a new message:
// Server side io.on('connection', (socket) => { socket.on('newMessage', (message) => { io.emit('newMessage', message); // Broadcast to all connected clients }); }); // Client side socket.on('newMessage', (message) => { console.log('New message received:', message); // Update UI with the new message });
Enhancing User Experience with External Resources
To further enrich your real-time notifications, consider integrating external APIs or services. For instance, you can leverage Pusher or Firebase Cloud Messaging (FCM) to send push notifications to mobile devices. Additionally, incorporating data visualization libraries like Chart.js can provide real-time analytics to users.
Here are a few resources to help you explore these options further:
- Pusher – A hosted service that provides real-time messaging via WebSocket.
- Firebase Cloud Messaging – Google’s cross-platform messaging solution for reliably delivering messages at no cost.
- Chart.js – Simple yet flexible JavaScript charting for designers and developers.
Conclusion
By leveraging the power of Node.js and Socket.IO, you can seamlessly integrate real-time notifications into your applications, enhancing user engagement and providing a more immersive experience. Whether you’re building a messaging platform, a live streaming app, or a collaborative tool, the combination of these technologies empowers you to deliver timely updates and keep users connected like never before.
Start revolutionizing your app today with real-time notifications powered by Node.js and Socket.IO!
Remember, in the world of digital innovation, staying ahead means keeping your users in the loop in real-time.
Feel free to reach out with any questions or share your experiences implementing real-time notifications in the comments below!
Happy coding!
Table of Contents
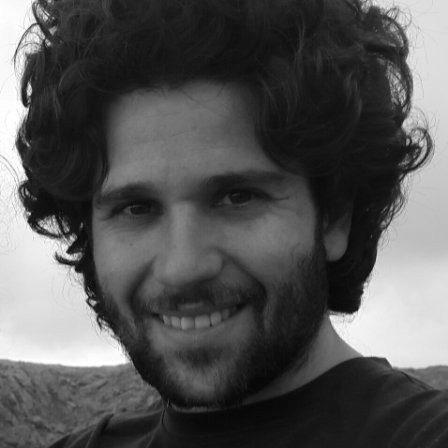
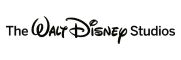