What is RESTful API in Node.js?
RESTful API (Representational State Transfer) is an architectural style for designing networked applications, and it is commonly used in Node.js for building web APIs. RESTful APIs are designed around resources, which are identified by unique URLs (Uniform Resource Locators). These APIs use standard HTTP methods (GET, POST, PUT, DELETE, etc.) to perform CRUD (Create, Read, Update, Delete) operations on resources.
Key principles of RESTful APIs in Node.js include:
- Stateless Communication: Each request from a client to the server contains all the information needed to process the request. The server does not store any client state between requests, which simplifies scaling and improves reliability.
- Uniform Interface: RESTful APIs use a uniform interface that consists of standard HTTP methods (GET, POST, PUT, DELETE) for interacting with resources. This uniformity simplifies client-server communication and promotes consistency.
- Resource-Based: RESTful APIs are built around resources, which are identified by unique URLs. Resources represent the data entities in the application domain, such as users, products, or articles.
- Representation: Resources in RESTful APIs are represented in a format such as JSON (JavaScript Object Notation) or XML (eXtensible Markup Language). Clients can request different representations of the same resource using content negotiation.
- State Transfer: The state of a resource is transferred between the client and server through representations. Clients can create, read, update, or delete resources by sending HTTP requests with appropriate methods.
In Node.js, developers use frameworks like Express.js to implement RESTful APIs by defining routes for different HTTP methods and handling requests accordingly. Express.js provides built-in support for routing, middleware, and request handling, making it well-suited for building RESTful APIs in Node.js.
Here’s an example of implementing a simple RESTful API in Node.js using Express.js:
javascript Copy code const express = require('express'); const app = express(); // Sample data (array of objects) let books = [ { id: 1, title: 'The Great Gatsby', author: 'F. Scott Fitzgerald' }, { id: 2, title: 'To Kill a Mockingbird', author: 'Harper Lee' }, { id: 3, title: '1984', author: 'George Orwell' } ]; // GET /books - Get all books app.get('/books', (req, res) => { res.json(books); }); // GET /books/:id - Get a single book by ID app.get('/books/:id', (req, res) => { const bookId = parseInt(req.params.id); const book = books.find(book => book.id === bookId); if (!book) { return res.status(404).json({ error: 'Book not found' }); } res.json(book); }); // POST /books - Create a new book app.post('/books', (req, res) => { const { title, author } = req.body; if (!title || !author) { return res.status(400).json({ error: 'Title and author are required' }); } const newBook = { id: books.length + 1, title, author }; books.push(newBook); res.status(201).json(newBook); }); // PUT /books/:id - Update an existing book by ID app.put('/books/:id', (req, res) => { const bookId = parseInt(req.params.id); const { title, author } = req.body; const bookIndex = books.findIndex(book => book.id === bookId); if (bookIndex === -1) { return res.status(404).json({ error: 'Book not found' }); } books[bookIndex] = { ...books[bookIndex], title, author }; res.json(books[bookIndex]); }); // DELETE /books/:id - Delete a book by ID app.delete('/books/:id', (req, res) => { const bookId = parseInt(req.params.id); const bookIndex = books.findIndex(book => book.id === bookId); if (bookIndex === -1) { return res.status(404).json({ error: 'Book not found' }); } books.splice(bookIndex, 1); res.status(204).send(); }); // Start the server const port = process.env.PORT || 3000; app.listen(port, () => { console.log(`Server is running on port ${port}`); });
In this example, we define routes for CRUD operations on a collection of books. The routes correspond to different HTTP methods: GET (retrieve), POST (create), PUT (update), and DELETE (delete). Each route handles requests and interacts with the books array to perform the desired operation. This is a simple illustration of implementing a RESTful API in Node.js using Express.js, and real-world APIs may involve more complex logic and data persistence mechanisms.
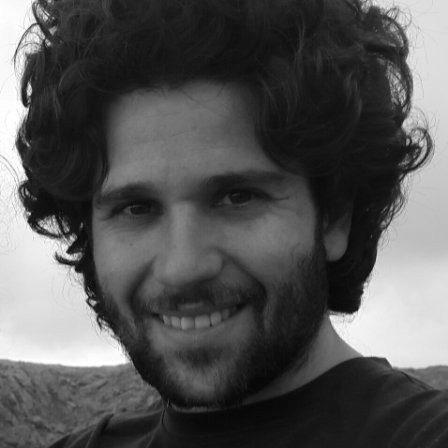
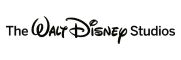