Building RESTful APIs with Node.js and Express
In the modern web development landscape, building robust and efficient APIs is a crucial skill. Representational State Transfer (REST) has emerged as a standard architectural style for designing networked applications. In this tutorial, we’ll dive into the process of creating RESTful APIs using the popular Node.js framework, Express. Whether you’re a beginner or an experienced developer, this guide will provide you with a comprehensive understanding of building APIs that communicate seamlessly with various clients.
Table of Contents
1. Introduction to RESTful APIs
1.1. What is a RESTful API?
A RESTful API (Representational State Transfer) is an architectural style that defines a set of constraints for creating web services. It relies on the use of standard HTTP methods (GET, POST, PUT, DELETE) to perform operations on resources. RESTful APIs are designed to be stateless, scalable, and easily consumed by various clients, including web browsers, mobile devices, and other applications.
1.2. Why choose Node.js and Express?
Node.js is a server-side JavaScript runtime that excels in building scalable and high-performance applications. When combined with the Express.js framework, it provides a solid foundation for creating RESTful APIs. Express simplifies the process of handling HTTP requests, routing, middleware, and more. Its flexibility allows developers to structure their projects in a way that suits their needs while ensuring optimal performance.
2. Setting Up Your Development Environment
2.1. Installing Node.js and npm
Before you start building your RESTful API, you need to have Node.js and npm (Node Package Manager) installed on your machine. Visit the official Node.js website and download the installer for your operating system. Once installed, you can verify the installation by running the following commands in your terminal:
bash node -v npm -v
2.2. Creating a New Node.js Project
After confirming that Node.js and npm are installed, you can create a new Node.js project by following these steps:
Open your terminal and navigate to the directory where you want to create your project.
Run the following command to initialize a new Node.js project:
bash npm init
You’ll be prompted to provide information about your project, such as its name, version, description, and entry point. You can either follow the prompts or add the –yes flag to accept the default values.
Once the initialization is complete, a package.json file will be generated in your project directory. This file contains metadata about your project and its dependencies.
3. Creating Your First Express Application
3.1. Installing Express
To create an Express application, you need to install the Express package. In your project directory, run the following command:
bash npm install express
3.2. Building the Basic Server Structure
With Express installed, you can now start building your first API. Create a new file named app.js (or any other name you prefer) in your project directory. Open the file and add the following code:
javascript const express = require('express'); const app = express(); const port = 3000; app.get('/', (req, res) => { res.send('Hello, World!'); }); app.listen(port, () => { console.log(`Server is running on port ${port}`); });
In this code, you’ve imported the Express module, created an instance of the Express application, and defined a route that responds with “Hello, World!” when the root URL is accessed. Finally, the server is started and listening on port 3000.
To run your Express application, execute the following command in your terminal:
bash node app.js
Open your web browser and navigate to http://localhost:3000 to see your API in action. You should see the “Hello, World!” message displayed in your browser.
4. Designing RESTful Routes
4.1. HTTP Methods and API Endpoints
RESTful APIs utilize different HTTP methods to perform various operations on resources. The most common HTTP methods used in RESTful APIs are:
- GET: Retrieve data from the server.
- POST: Create a new resource on the server.
- PUT: Update an existing resource on the server.
- DELETE: Remove a resource from the server.
These methods are associated with specific API endpoints, which are URLs that correspond to specific resources. For example:
- GET /users: Retrieve a list of users.
- POST /users: Create a new user.
- PUT /users/:id: Update a user with a specific ID.
- DELETE /users/:id: Delete a user with a specific ID.
4.2. Creating Routes for GET, POST, PUT, and DELETE Requests
In your Express application, you can define routes for different HTTP methods to handle various requests. Let’s create routes for managing a collection of tasks. In your app.js file, replace the existing code with the following:
javascript const express = require('express'); const app = express(); const port = 3000; const tasks = []; app.use(express.json()); app.get('/tasks', (req, res) => { res.json(tasks); }); app.post('/tasks', (req, res) => { const newTask = req.body; tasks.push(newTask); res.status(201).json(newTask); }); app.put('/tasks/:id', (req, res) => { const taskId = req.params.id; const updatedTask = req.body; tasks[taskId] = updatedTask; res.json(updatedTask); }); app.delete('/tasks/:id', (req, res) => { const taskId = req.params.id; tasks.splice(taskId, 1); res.sendStatus(204); }); app.listen(port, () => { console.log(`Server is running on port ${port}`); });
In this code, you’ve added an array called tasks to store task objects. The express.json() middleware is used to parse incoming JSON data. Routes for GET, POST, PUT, and DELETE requests are defined. When you access these endpoints, the corresponding operations are performed on the tasks array.
5. Handling Data Persistence
5.1. Integrating with a Database (MongoDB)
While storing data in memory can be useful for development purposes, production applications often require a more robust solution. Integrating with a database allows for data persistence and scalability. In this section, we’ll use MongoDB, a popular NoSQL database, to store and manage our tasks.
To get started, you’ll need to have MongoDB installed and running on your machine or a remote server. You’ll also need to install the mongoose package, which simplifies working with MongoDB in Node.js.
Install mongoose using the following command:
bash npm install mongoose
5.2. Using Mongoose for Data Modeling
Mongoose is an Object Data Modeling (ODM) library for MongoDB and Node.js. It provides a straightforward way to define schemas, create models, and interact with the database. Let’s modify our Express application to use Mongoose for data modeling and storage.
Create a new directory named models in your project directory. Inside the models directory, create a file named task.js. Add the following code to define the Task schema and model:
javascript const mongoose = require('mongoose'); const taskSchema = new mongoose.Schema({ title: String, description: String, completed: Boolean, }); const Task = mongoose.model('Task', taskSchema); module.exports = Task;
Now, in your app.js file, update the code to integrate Mongoose and the MongoDB database:
javascript const express = require('express'); const mongoose = require('mongoose'); const Task = require('./models/task'); const app = express(); const port = 3000; mongoose.connect('mongodb://localhost:27017/taskdb', { useNewUrlParser: true, useUnifiedTopology: true, }); app.use(express.json()); // Routes for handling tasks app.listen(port, () => { console.log(`Server is running on port ${port}`); });
With this setup, your Express application is connected to the MongoDB database. You can now use the Task model to interact with the database and perform CRUD operations on tasks.
6. Request and Response Handling
6.1. Parsing Incoming Data
In RESTful APIs, you often need to handle incoming data from clients. The express.json() middleware we’ve been using parses incoming JSON data. For other types of data, such as form submissions, you can use additional middleware like express.urlencoded().
6.2. Sending Appropriate HTTP Responses
When responding to client requests, it’s important to send appropriate HTTP status codes and response bodies. Here are some common HTTP status codes and their meanings:
- 200 OK: The request was successful.
- 201 Created: The resource was successfully created.
- 400 Bad Request: The request was malformed or invalid.
- 404 Not Found: The requested resource was not found.
- 500 Internal Server Error: An error occurred on the server.
You can set status codes and send JSON responses using the res.status() and res.json() methods, respectively.
7. Error Handling and Validation
7.1. Implementing Error Middleware
Error handling is a critical aspect of building robust APIs. Express allows you to define custom error-handling middleware functions. These functions are invoked when an error occurs during the request-response cycle. You can use them to format error responses and log error details.
javascript app.use((err, req, res, next) => { console.error(err.stack); res.status(500).json({ error: 'Something went wrong!' }); });
7.2. Validating User Input with express-validator
User input validation is essential to ensure the integrity of your data and prevent security vulnerabilities. The express-validator library simplifies the process of validating and sanitizing user input.
Install express-validator using the following command:
bash npm install express-validator
In your route handlers, you can use the validation functions provided by express-validator to check the validity of user input and sanitize it if necessary.
javascript const { body, validationResult } = require('express-validator'); app.post( '/tasks', [ body('title').notEmpty().withMessage('Title is required'), body('description').notEmpty().withMessage('Description is required'), ], (req, res) => { const errors = validationResult(req); if (!errors.isEmpty()) { return res.status(400).json({ errors: errors.array() }); } // Create a new task // ... } );
8. Structuring Your Project
8.1. Separation of Concerns
As your API grows, maintaining a clean and organized project structure becomes crucial. Separating concerns by using distinct folders for routes, controllers, models, and middleware can greatly enhance the maintainability of your codebase.
9. Authentication and Security
9.1. Implementing User Authentication
Securing your API is essential to protect sensitive data and ensure authorized access. Implementing user authentication is a common approach to achieve this. Popular authentication mechanisms include JSON Web Tokens (JWT) and OAuth.
10. Testing Your APIs
10.1 Writing Unit Tests with Jest
Testing your APIs is a fundamental part of the development process. Unit tests help identify bugs and ensure that your code behaves as expected. Jest is a popular testing framework that provides tools for writing and running unit tests.
11. Deployment and Best Practices
11.1. Configuring Environment Variables
When deploying your API to different environments (development, staging, production), it’s crucial to use environment variables to manage configuration settings.
11.2. Deploying on Platforms like Heroku
Platforms like Heroku simplify the deployment process and provide tools for scaling and managing your application in the cloud.
11.3. API Documentation and Versioning
Documenting your API is essential for developers who want to integrate with it. Tools like Swagger or API Blueprint can help you generate comprehensive API documentation automatically.
Conclusion
Building RESTful APIs with Node.js and Express empowers you to create scalable, efficient, and feature-rich web services. By following the steps outlined in this guide, you’ve learned how to set up your development environment, create routes, handle data persistence, validate user input, and implement error handling and security. Remember that building great APIs requires continuous learning and practice, so keep exploring and experimenting with new techniques to enhance your skills further. With your newfound knowledge, you’re well-equipped to embark on the journey of crafting powerful APIs that cater to a diverse range of client needs.
Table of Contents
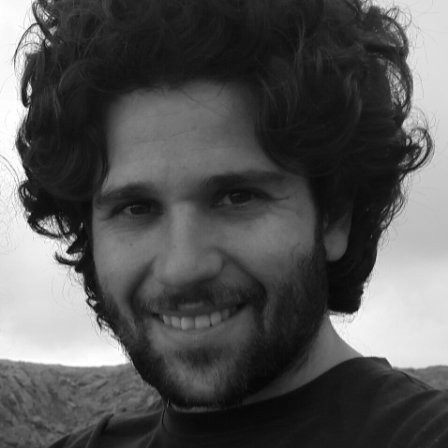
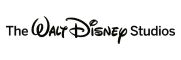