Securing Node.js Applications: Best Practices and Techniques
Node.js has become a popular choice for building scalable and efficient web applications. However, with its growing popularity, it has also become a target for various security threats. Securing Node.js applications is crucial to protect sensitive data and ensure the integrity of your systems. This blog post explores best practices and techniques to secure Node.js applications, providing practical examples and code snippets.
Understanding Security Threats in Node.js
Before diving into specific security practices, it’s important to understand the common threats that can affect Node.js applications. These include:
- Injection Attacks: SQL injection, command injection, and other forms of injection attacks.
- Cross-Site Scripting (XSS): Injecting malicious scripts into web pages.
- Cross-Site Request Forgery (CSRF): Forcing users to execute unwanted actions on a web application.
- Insecure Dependencies: Using outdated or vulnerable third-party packages.
Best Practices for Securing Node.js Applications
1. Use HTTPS for Secure Communication
One of the first steps in securing your Node.js application is to ensure that all communication between the client and server is encrypted. HTTPS is essential for protecting data in transit from eavesdropping and man-in-the-middle attacks.
Example: Setting up HTTPS in a Node.js application.
```javascript const https = require('https'); const fs = require('fs'); const express = require('express'); const app = express(); const options = { key: fs.readFileSync('server.key'), cert: fs.readFileSync('server.cert') }; https.createServer(options, app).listen(443, () => { console.log('Server running on https://localhost:443'); }); ```
2. Sanitize and Validate User Input
Sanitizing and validating user input is crucial to prevent injection attacks. Always assume that user input is malicious and ensure that it is properly sanitized before being processed.
Example:Validating and sanitizing input using the `validator` library.
```javascript const validator = require('validator'); app.post('/submit', (req, res) => { const userInput = req.body.input; if (validator.isAlphanumeric(userInput)) { // Proceed with processing } else { res.status(400).send('Invalid input'); } }); ```
3. Implement Strong Authentication and Authorization
Authentication and authorization are key to ensuring that only authorized users have access to your application. Implement strong password policies, use two-factor authentication (2FA), and restrict access based on roles.
Example:Using `passport.js` for authentication.
```javascript const passport = require('passport'); const LocalStrategy = require('passport-local').Strategy; passport.use(new LocalStrategy((username, password, done) => { // Implement user authentication logic here })); app.post('/login', passport.authenticate('local', { successRedirect: '/dashboard', failureRedirect: '/login' })); ```
4. Secure Your Dependencies
Node.js applications often rely on third-party packages. It’s important to keep these dependencies up to date and ensure that they do not contain vulnerabilities.
Example: Auditing dependencies with `npm audit`.
```bash npm audit ```
This command scans your project for vulnerabilities and suggests fixes.
5. Protect Against Cross-Site Scripting (XSS)
XSS attacks occur when an attacker injects malicious scripts into your web application. To prevent XSS, ensure that you escape and sanitize all user-generated content before rendering it on the page.
Example: Using `helmet` to set security-related HTTP headers.
```javascript const helmet = require('helmet'); app.use(helmet()); ```
Helmet helps secure your Node.js application by setting various HTTP headers to protect against XSS and other common vulnerabilities.
6. Use Environment Variables for Configuration
Never hard-code sensitive information such as API keys, database credentials, or encryption keys directly in your code. Instead, use environment variables to manage configuration securely.
Example:Accessing environment variables in Node.js.
```javascript const dbPassword = process.env.DB_PASSWORD; ```
Store sensitive information in a `.env` file and use the `dotenv` package to load them into your application.
Conclusion
Securing Node.js applications requires a proactive approach to prevent common vulnerabilities and threats. By following these best practices and implementing the provided techniques, you can significantly enhance the security of your Node.js applications. From using HTTPS to securing dependencies and validating user input, these steps will help protect your application and your users.
Further Reading:
Table of Contents
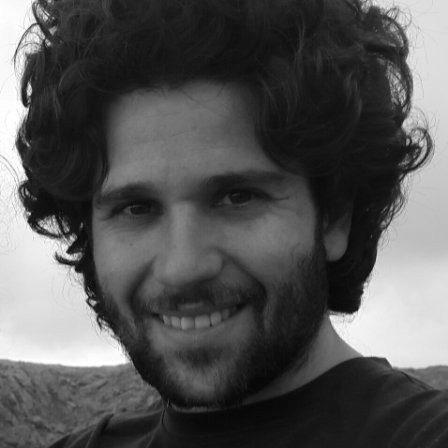
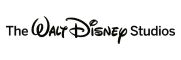