Server-Side Rendering with Node.js and React
In the ever-evolving landscape of web development, delivering fast-loading, SEO-friendly applications is crucial. Server-Side Rendering (SSR) has emerged as a powerful technique to achieve these goals. By combining the strengths of Node.js and React, developers can create web applications that load quickly, improve search engine visibility, and enhance the overall user experience. In this guide, we’ll delve into the fundamentals of Server-Side Rendering, explore its benefits, and provide step-by-step instructions, complete with code samples, on how to implement SSR with Node.js and React.
Table of Contents
1. Understanding Server-Side Rendering:
Server-Side Rendering (SSR) is a technique that involves rendering web pages on the server before delivering them to the client’s browser. Unlike Client-Side Rendering (CSR), where the browser fetches a minimal HTML document and populates it with JavaScript-generated content, SSR sends fully rendered HTML pages to the browser. This results in faster initial page loads, improved performance, and enhanced search engine optimization.
1.1. Benefits of Server-Side Rendering:
- Improved Performance: With SSR, the browser receives pre-rendered HTML content, reducing the time it takes to load the page and display meaningful content to users.
- Enhanced SEO: Search engines can easily crawl and index the content of SSR pages since the HTML is present in the initial response. This leads to better search engine rankings and visibility.
- Accessibility: SSR benefits users with slower internet connections or less powerful devices, as they can access content faster without waiting for JavaScript to load.
- Social Media Sharing: When a page is shared on social media platforms, the pre-rendered content is readily available, creating a more appealing and informative link preview.
2. Setting Up the Development Environment:
To start building SSR applications with Node.js and React, ensure you have Node.js and npm installed. You can download them from the official Node.js website. After installation, you can create a new React application using the following commands:
bash npx create-react-app ssr-app cd ssr-app
3. Implementing Server-Side Rendering with Node.js and React:
- Creating Express.js Server: Express.js is a popular Node.js framework for building web applications. Install it using npm install express. Create a new file, let’s call it server.js, and set up a basic Express server:
javascript const express = require('express'); const app = express(); const PORT = process.env.PORT || 3000; app.listen(PORT, () => { console.log(`Server is listening on port ${PORT}`); });
- Integrating React Components: Install the necessary packages using npm install react react-dom. Now, create a React component that you want to render on the server. For instance, let’s create a simple component named App in a file called App.js:
jsx import React from 'react'; const App = () => { return ( <div> <h1>Hello SSR with Node.js and React</h1> <p>Welcome to our SSR application!</p> </div> ); }; export default App;
- Rendering React Components on the Server: To render React components on the server, you need to install @babel/preset-react and @babel/preset-env using npm install @babel/preset-react @babel/preset-env. Create a Babel configuration file named .babelrc in your project’s root directory:
json { "presets": ["@babel/preset-react", "@babel/preset-env"] }
- Next, modify your server.js to import and render the App component:
javascript import express from 'express'; import React from 'react'; import ReactDOMServer from 'react-dom/server'; import App from './App'; // Import the App component const app = express(); const PORT = process.env.PORT || 3000; app.get('/', (req, res) => { const appContent = ReactDOMServer.renderToString(<App />); res.send(` <html> <head> <title>SSR with Node.js and React</title> </head> <body> <div id="root">${appContent}</div> <script src="/static/js/bundle.js"></script> </body> </html> `); }); app.listen(PORT, () => { console.log(`Server is listening on port ${PORT}`); });
- Sending Initial Data to the Client: To send initial data from the server to the client, modify the App component to accept props and use them during rendering:
jsx import React from 'react'; const App = ({ message }) => { return ( <div> <h1>Hello SSR with Node.js and React</h1> <p>{message}</p> </div> ); }; export default App;
- In your server.js, pass the initial data to the App component:
javascript // ... app.get('/', (req, res) => { const message = 'Welcome to our SSR application!'; const appContent = ReactDOMServer.renderToString(<App message={message} />); // ... }); // ...
4. Handling Data Fetching:
Fetching data on the server and sending it to the client is a crucial aspect of SSR. You might need to retrieve data from APIs or databases before rendering the page.
- Fetching Data on the Server: Install any necessary packages, such as axios, using npm install axios. Suppose you want to fetch some data from an API and display it on the page. Modify your server.js to fetch data before rendering the App component:
javascript import axios from 'axios'; // ... app.get('/', async (req, res) => { try { const response = await axios.get('https://api.example.com/data'); const apiData = response.data; const appContent = ReactDOMServer.renderToString( <App apiData={apiData} /> ); // ... } catch (error) { console.error('Error fetching API data:', error); } }); // ...
- Data Hydration on the Client: To make the data fetched on the server available to the client, you can embed it in a script tag:
javascript app.get('/', async (req, res) => { // Fetch and renderToString as before... res.send(` <html> <head> <title>SSR with Node.js and React</title> </head> <body> <div id="root">${appContent}</div> <script> window.__INITIAL_DATA__ = ${JSON.stringify(apiData)}; </script> <script src="/static/js/bundle.js"></script> </body> </html> `); });
5. SEO Benefits of Server-Side Rendering:
Search engine crawlers have difficulty parsing JavaScript-generated content, which makes traditional Client-Side Rendering less SEO-friendly. With SSR, search engines can easily crawl and index your content since it’s already present in the initial HTML response. This leads to better search engine rankings and increased visibility in search results.
6. Performance Optimization:
SSR significantly reduces the time-to-content for users, enhancing their overall experience. The initial HTML content is available immediately, even before JavaScript and other assets are fully loaded. This is especially beneficial for users on slower connections or less powerful devices.
7. Challenges and Considerations:
While SSR offers numerous benefits, it’s important to consider potential challenges:
- Server Load and Caching: SSR can increase server load, especially if your application experiences high traffic. Implementing caching mechanisms can help alleviate this issue and improve performance.
- Balancing Client-Side and Server-Side Logic: Finding the right balance between client-side and server-side logic is crucial. Certain interactivity might still be better suited for client-side rendering to avoid overloading the server.
- Code Splitting and SSR: Code splitting, a technique to optimize JavaScript bundle size, can be complex when combined with SSR. Careful consideration is needed to ensure the balance between performance gains and effective SSR.
8. Deploying SSR Applications:
Deploying SSR applications involves additional considerations compared to traditional client-side apps. Choose a hosting solution that supports Node.js, such as Heroku, AWS, or DigitalOcean. Build your application using a script defined in your package.json:
json { // ... "scripts": { "start": "node server.js", "build": "npm run build:client && npm run build:server", "build:client": "react-scripts build", "build:server": "babel server.js --out-file dist/server.js" }, // ... }
After building, you can run your server using npm start.
Conclusion
Server-Side Rendering with Node.js and React is a powerful technique that enhances the performance and SEO of web applications. By rendering content on the server and sending pre-rendered HTML to the client, developers can achieve faster loading times, improved search engine visibility, and enhanced user experiences. While SSR introduces challenges, such as server load and code splitting complexities, the benefits it offers make it a valuable approach in modern web development. As the web continues to evolve, Server-Side Rendering remains a crucial tool in creating high-performing, user-friendly applications.
Table of Contents
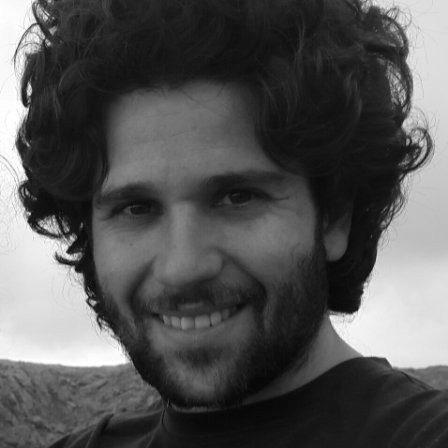
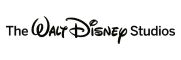