Implementing Server-Side Rendering with Node.js and Next.js
Server-side rendering (SSR) is a technique that allows web applications to be rendered on the server rather than the client, improving performance and search engine optimization (SEO). Node.js, combined with Next.js, provides a robust solution for implementing SSR in modern web applications. This blog explores how to utilize Node.js and Next.js for server-side rendering, complete with practical examples and best practices.
Understanding Server-Side Rendering
Server-side rendering involves generating HTML content on the server and sending it to the client. This approach has several benefits, including faster initial page loads and better SEO, as search engines can crawl pre-rendered content more effectively.
Using Node.js and Next.js for SSR
Next.js is a React framework that simplifies the process of server-side rendering. It integrates seamlessly with Node.js, making it an ideal choice for building high-performance web applications. Here’s how you can use Next.js and Node.js to implement server-side rendering.
1. Setting Up Your Next.js Project
To get started with server-side rendering using Next.js, you need to set up a Next.js project. You can initialize a new project with the following commands:
```bash npx create-next-app@latest my-next-app cd my-next-app ```
2. Creating a Server-Side Rendered Page
Next.js supports server-side rendering out of the box. You can create a page that is rendered on the server by using the `getServerSideProps` function. This function fetches data on the server side before rendering the page.
Example: Fetching Data with `getServerSideProps`
```javascript // pages/index.js import React from 'react'; const Home = ({ data }) => { return ( <div> <h1>Server-Side Rendered Page</h1> <ul> {data.map((item, index) => ( <li key={index}>{item}</li> ))} </ul> </div> ); }; export async function getServerSideProps() { // Fetch data from an API or database const response = await fetch('https://api.example.com/data'); const data = await response.json(); return { props: { data, }, }; } export default Home; ```
In this example, the `getServerSideProps` function fetches data from an API and passes it to the page component as props.
3. Handling Dynamic Routes with SSR
Next.js also supports server-side rendering for dynamic routes. You can use the `getServerSideProps` function to fetch data for dynamic pages based on route parameters.
Example: Dynamic Route with SSR
```javascript // pages/posts/[id].js import React from 'react'; const Post = ({ post }) => { return ( <div> <h1>{post.title}</h1> <p>{post.content}</p> </div> ); }; export async function getServerSideProps(context) { const { id } = context.params; const response = await fetch(`https://api.example.com/posts/${id}`); const post = await response.json(); return { props: { post, }, }; } export default Post; ```
Here, the `getServerSideProps` function fetches data for a specific post based on the `id` parameter from the URL.
4. Optimizing Performance with SSR
Server-side rendering can significantly improve performance, but there are additional optimizations you can make:
– Caching: Implement caching strategies for API responses to reduce server load and improve response times.
– Code Splitting: Use dynamic imports to split code and load components only when needed, reducing the initial load time.
Example: Code Splitting with Dynamic Imports
```javascript // pages/index.js import dynamic from 'next/dynamic'; const DynamicComponent = dynamic(() => import('../components/DynamicComponent')); const Home = () => { return ( <div> <h1>Home Page</h1> <DynamicComponent /> </div> ); }; export default Home; ```
5. Deploying Your Next.js Application
You can deploy your Next.js application to various platforms, including Vercel, AWS, or your custom server. For deployment to Vercel, you can simply push your code to a Git repository, and Vercel will handle the rest.
Example: Deploying to Vercel
- Create a Vercel account and link it to your Git repository.
- Vercel will automatically build and deploy your Next.js application.
Conclusion
Implementing server-side rendering with Node.js and Next.js can greatly enhance your web application’s performance and SEO. By leveraging the capabilities of Next.js for SSR, you can create fast, scalable, and SEO-friendly web applications. Utilizing these tools effectively will lead to a more optimized and user-friendly web experience.
Further Reading:
Table of Contents
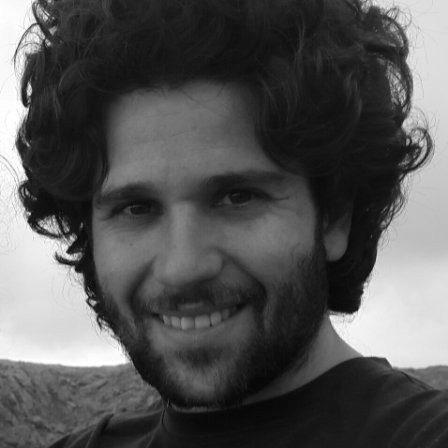
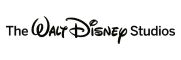