Building Serverless APIs with Node.js and AWS API Gateway
In today’s fast-paced world of software development, scalability and flexibility are no longer just desirable traits but absolute necessities. With the rise of cloud computing, serverless architecture has emerged as a powerful solution for building highly scalable applications without the hassle of managing infrastructure. One of the key components of serverless architecture is the ability to deploy APIs quickly and efficiently. In this guide, we’ll explore how to leverage Node.js and AWS API Gateway to build serverless APIs that can scale to meet the demands of your application.
Understanding Serverless Architecture
Before diving into the specifics of building serverless APIs, let’s take a moment to understand what serverless architecture entails. At its core, serverless architecture allows developers to focus on writing code without worrying about managing servers or infrastructure. Instead of provisioning and managing servers, applications are broken down into smaller, event-driven functions that are executed in response to triggers. This approach offers several benefits, including automatic scaling, reduced operational overhead, and cost efficiency.
Getting Started with Node.js and AWS Lambda
Node.js has become a popular choice for building serverless applications due to its lightweight runtime and asynchronous programming model. Combined with AWS Lambda, which allows you to run code without provisioning or managing servers, Node.js provides a powerful platform for building serverless APIs.
To get started, you’ll need an AWS account and the AWS Command Line Interface (CLI) installed on your machine. Once you have these prerequisites in place, you can create a new Lambda function using the AWS Management Console or the CLI. Here’s a basic example of a Node.js Lambda function that handles an HTTP request:
exports.handler = async (event) => { const response = { statusCode: 200, body: JSON.stringify('Hello from Lambda!'), }; return response; };
Building APIs with AWS API Gateway
While Lambda functions provide the compute power for your serverless application, AWS API Gateway acts as the front door, allowing you to create, publish, maintain, monitor, and secure APIs at any scale. API Gateway supports various integration types, including Lambda integration, which allows you to seamlessly connect your Lambda functions to HTTP endpoints.
To create a new API with API Gateway, simply navigate to the API Gateway console, click “Create API,” and choose the “HTTP API” option. From there, you can define your API’s routes, methods, and integrations with Lambda functions. API Gateway also offers features such as request validation, authorization, and throttling to help you secure and control access to your APIs.
Best Practices for Serverless API Development
When building serverless APIs with Node.js and AWS API Gateway, it’s essential to follow best practices to ensure optimal performance, scalability, and maintainability. Here are a few tips to keep in mind:
- Keep Functions Stateless: Since Lambda functions are stateless, it’s crucial to design your functions to be idempotent and handle each request independently.
- Use Environment Variables: Store configuration values such as database credentials or API keys as environment variables to keep sensitive information secure and separate from your code.
- Implement Error Handling: Handle errors gracefully within your Lambda functions and return appropriate HTTP status codes and error messages to the client.
- Monitor and Debug: Utilize AWS CloudWatch to monitor the performance and health of your Lambda functions and API Gateway endpoints. Implement logging and tracing to facilitate debugging and troubleshooting.
- Automate Deployment: Streamline your deployment process using AWS CodePipeline or another CI/CD tool to automate the deployment of your serverless APIs.
Conclusion
Serverless architecture offers a compelling solution for building scalable and cost-effective APIs, and Node.js and AWS API Gateway provide a powerful combination for realizing the benefits of serverless computing. By following best practices and leveraging the capabilities of these technologies, you can build robust and reliable APIs that can scale to meet the demands of your application.
External Links:
Start building your serverless APIs today and unlock the full potential of cloud-native development. Happy coding!
Table of Contents
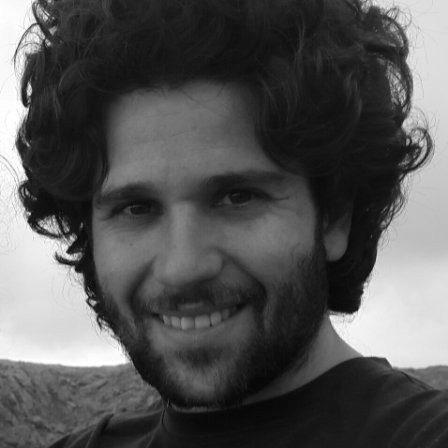
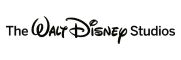