Building Serverless Functions with Node.js and Azure Functions
In the ever-evolving landscape of cloud computing, serverless architecture has emerged as a game-changer, allowing developers to focus solely on writing code without worrying about infrastructure management. Among the plethora of options available, Azure Functions stands out as a robust and scalable platform for building serverless applications. Coupled with the versatility of Node.js, developers can harness the full potential of serverless computing to create efficient and dynamic solutions. Let’s delve into the world of building serverless functions with Node.js and Azure Functions.
Why Node.js and Azure Functions?
Node.js has garnered immense popularity among developers due to its lightweight, event-driven architecture and vast ecosystem of libraries and frameworks. Its non-blocking I/O model makes it ideal for handling asynchronous operations, making it a natural fit for serverless computing. Azure Functions, on the other hand, offers seamless integration with other Azure services, automatic scaling, and pay-per-execution pricing model, making it a compelling choice for building serverless applications.
Getting Started
Before diving into building serverless functions, ensure you have an Azure account and the Azure Functions Core Tools installed. Once set up, creating a new Azure Functions project is as simple as running a few commands in your terminal:
func init MyFunctionApp cd MyFunctionApp func new
Select the Node.js runtime when prompted, and you’re ready to start writing serverless functions.
Writing Serverless Functions
Let’s illustrate the power of serverless functions with a practical example. Consider a scenario where you want to build an API for processing image uploads. With Azure Functions and Node.js, you can achieve this effortlessly.
module.exports = async function (context, req) { context.log('Processing image upload...'); // Code for processing image upload goes here return { status: 200, body: "Image uploaded successfully" }; };
This simple function listens for HTTP requests, processes the image upload, and returns a success response. Azure Functions take care of scaling and managing the execution environment, allowing you to focus solely on the business logic.
Integrating with External Services
One of the key advantages of serverless architecture is its ability to seamlessly integrate with external services. Let’s enhance our image upload function to store uploaded images in Azure Blob Storage:
const { BlobServiceClient } = require("@azure/storage-blob"); module.exports = async function (context, req) { // Initialize Azure Blob Storage client const blobServiceClient = BlobServiceClient.fromConnectionString(process.env.AzureWebJobsStorage); const containerClient = blobServiceClient.getContainerClient("images"); // Code for processing and storing image goes here return { status: 200, body: "Image uploaded successfully" }; };
By leveraging the Azure Storage SDK for Node.js, we can seamlessly integrate with Azure Blob Storage to store our uploaded images securely.
Monitoring and Debugging
Azure Functions provides robust monitoring and debugging capabilities to help you track the performance of your serverless functions. You can monitor function execution, view logs, and diagnose issues using Azure Monitor and Application Insights.
Conclusion
In conclusion, building serverless functions with Node.js and Azure Functions offers unparalleled flexibility, scalability, and efficiency. Whether you’re developing APIs, processing data, or building event-driven applications, serverless architecture empowers you to focus on innovation without being bogged down by infrastructure management. With Azure Functions and Node.js, the possibilities are endless.
External Resources:
Embark on your serverless journey today and unlock the true potential of cloud computing with Node.js and Azure Functions!
Table of Contents
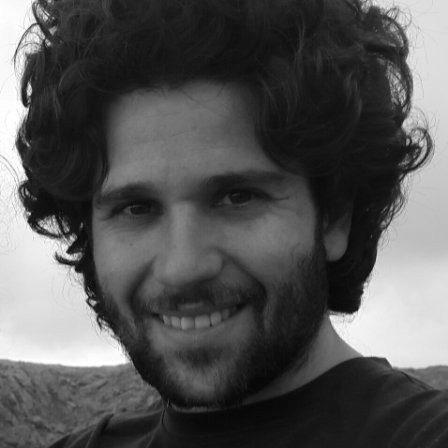
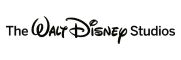