Node.js Q & A
How do you handle SSL/TLS in Node.js?
SSL/TLS (Secure Sockets Layer/Transport Layer Security) is essential for securing network communication and encrypting data exchanged between clients and servers in Node.js applications. Here’s how you can handle SSL/TLS in Node.js:
- Using HTTPS Module:
- Node.js provides the https module, which allows developers to create HTTPS servers that support SSL/TLS encryption. To create an HTTPS server, simply replace http with https when creating a server instance.
- Example:
- javascript
- Copy code
const https = require('https'); const fs = require('fs'); const options = { key: fs.readFileSync('server-key.pem'), cert: fs.readFileSync('server-cert.pem') }; const server = https.createServer(options, (req, res) => { res.writeHead(200); res.end('Hello, HTTPS!'); }); server.listen(443);
- Generating SSL/TLS Certificates:
-
-
- Generate SSL/TLS certificates (private key and public certificate) using tools like OpenSSL or Let’s Encrypt. Make sure to secure these certificates and store them securely on the server.
-
- Configuring Server Options:
-
-
- When creating an HTTPS server, provide the private key and public certificate as options. Optionally, you can also provide additional options such as CA certificates, passphrase, or SSL/TLS protocol versions.
-
- Enforcing HTTPS Redirects:
-
-
- Use middleware or server configuration to enforce HTTPS redirects for HTTP requests. Redirect HTTP traffic to HTTPS to ensure all communication is encrypted and secure.
-
- Certificate Management:
-
-
- Regularly renew SSL/TLS certificates before they expire and follow best practices for certificate management, including key rotation, certificate revocation, and certificate pinning.
-
- Hardening SSL/TLS Configuration:
-
-
- Configure SSL/TLS settings securely by disabling weak ciphers, enabling Perfect Forward Secrecy (PFS), enabling HTTP Strict Transport Security (HSTS), and configuring security headers to enhance SSL/TLS security.
-
- Client-Side Configuration:
-
- Configure SSL/TLS settings on the client-side to ensure secure communication with the server. Verify server certificates, enable certificate pinning, and handle SSL/TLS errors gracefully in client applications.
By following these best practices, developers can effectively handle SSL/TLS in Node.js applications, encrypt sensitive data, and secure communication channels against eavesdropping, tampering, and man-in-the-middle attacks.
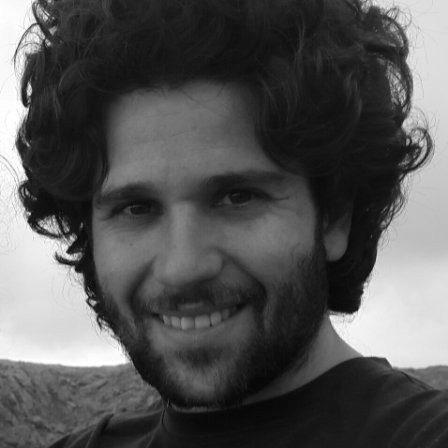
Previously at
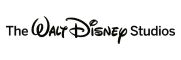
Experienced Principal Engineer and Fullstack Developer with a strong focus on Node.js. Over 5 years of Node.js development experience.