Understanding Streams in Node.js: A Deep Dive
In the world of Node.js, efficient data handling and manipulation are paramount, especially when dealing with large datasets. This is where streams come into play, providing a powerful mechanism for working with data in a way that is memory-efficient and responsive. In this comprehensive guide, we will delve deep into the concept of streams in Node.js, exploring their types, use cases, and practical implementation through code samples and examples.
Table of Contents
1. Introduction to Streams
1.1. What Are Streams?
In Node.js, streams are a fundamental concept for handling and manipulating data efficiently. A stream is essentially a flow of data that can be read from or written to in a continuous fashion, piece by piece, without loading the entire dataset into memory. This approach is especially advantageous when working with large files or network data, as it minimizes memory consumption and improves overall performance.
1.2. Why Use Streams?
The primary benefit of using streams is their memory efficiency. Instead of loading an entire dataset into memory, streams allow you to process data incrementally, significantly reducing the risk of memory exhaustion. Streams are also well-suited for scenarios where data arrives over time, such as reading data from a network socket or processing log files.
Moreover, streams enhance responsiveness. By breaking data into smaller chunks, streams enable faster data processing, making your applications more responsive to user interactions. This is crucial for real-time applications where immediate feedback is essential.
2. Types of Streams
Streams in Node.js come in four main types:
2.1. Readable Streams
Readable streams are used for reading data from a source, such as a file, HTTP response, or even user input. Examples of readable streams include fs.createReadStream for reading files and http.IncomingMessage for handling HTTP responses.
Code Sample: Reading from a Readable Stream
javascript const fs = require('fs'); const readableStream = fs.createReadStream('large-file.txt'); readableStream.on('data', (chunk) => { console.log(`Received ${chunk.length} bytes of data.`); }); readableStream.on('end', () => { console.log('Finished reading the file.'); });
2.2. Writable Streams
Writable streams, on the other hand, are used for writing data to a destination, such as a file or an HTTP request. Examples of writable streams include fs.createWriteStream for writing to files and http.ClientRequest for sending HTTP requests.
Code Sample: Writing to a Writable Stream
javascript const fs = require('fs'); const writableStream = fs.createWriteStream('output.txt'); writableStream.write('Hello, '); writableStream.write('world!'); writableStream.end();
2.3. Duplex Streams
Duplex streams represent streams that can both be read from and written to. They combine the functionality of both readable and writable streams. An example of a duplex stream is a TCP socket.
2.4. Transform Streams
Transform streams are a special type of duplex stream that allow for data modification as it passes through the stream. They are commonly used for tasks like data compression, encryption, or parsing. The zlib module’s compression streams are a prime example of transform streams.
Code Sample: Using a Transform Stream
javascript const fs = require('fs'); const zlib = require('zlib'); const readableStream = fs.createReadStream('input.txt'); const writableStream = fs.createWriteStream('output.txt.gz'); const gzipStream = zlib.createGzip(); readableStream.pipe(gzipStream).pipe(writableStream);
3. How Streams Work
3.1. The Stream Lifecycle
Streams in Node.js follow a lifecycle that consists of three main states: Readable, Writable, and Finished. A readable stream starts in the “Readable” state, where it emits data events as chunks of data are read. As the data is processed and written to a destination, a writable stream enters the “Writable” state. Finally, the stream transitions to the “Finished” state when all data has been read or written, and relevant events are emitted.
3.2. Flowing and Non-Flowing Streams
Streams can operate in two different modes: flowing and non-flowing. In flowing mode, data is continuously pushed from the source to the destination, and you need to actively listen for the data events to process it. In non-flowing mode, you manually request chunks of data to be read or written using the read() or write() methods.
4. Using Streams in Node.js
4.1. Reading from Readable Streams
Reading from a readable stream involves attaching listeners to the stream’s data and end events. The data event is emitted whenever a new chunk of data is available for consumption, while the end event indicates that there’s no more data to be read.
Code Sample: Reading from a Readable Stream
javascript const fs = require('fs'); const readableStream = fs.createReadStream('large-file.txt'); readableStream.on('data', (chunk) => { console.log(`Received ${chunk.length} bytes of data.`); }); readableStream.on('end', () => { console.log('Finished reading the file.'); });
4.2. Writing to Writable Streams
Writing to a writable stream involves using the write() method to send data to the stream and the end() method to signal the end of the writing process.
Code Sample: Writing to a Writable Stream
javascript const fs = require('fs'); const writableStream = fs.createWriteStream('output.txt'); writableStream.write('Hello, '); writableStream.write('world!'); writableStream.end();
4.3. Piping Streams
Piping is a powerful mechanism in Node.js streams that allows you to connect a readable stream to a writable stream. This enables automatic data transfer from the source stream to the destination stream.
Code Sample: Piping Streams
javascript const fs = require('fs'); const readableStream = fs.createReadStream('input.txt'); const writableStream = fs.createWriteStream('output.txt'); readableStream.pipe(writableStream);
4.4. Chaining Transform Streams
Chaining multiple transform streams together can be incredibly useful for performing complex data manipulations. This is achieved by piping the output of one transform stream into another.
Code Sample: Chaining Transform Streams
javascript const fs = require('fs'); const zlib = require('zlib'); const readableStream = fs.createReadStream('input.txt'); const writableStream = fs.createWriteStream('output.txt.gz'); const gzipStream = zlib.createGzip(); readableStream.pipe(gzipStream).pipe(writableStream);
5. Practical Use Cases
5.1. File I/O Operations
Streams are particularly useful for reading from and writing to files. By using streams, you can handle large files without overloading memory.
5.2. HTTP Requests and Responses
When working with HTTP requests and responses, streams enable you to handle data in chunks, making your applications more efficient and responsive.
5.3. Data Transformation and Manipulation
Transform streams allow you to process data on the fly, which is especially valuable when you need to modify data as it’s being read or written. This is commonly seen in compression, encryption, and data parsing tasks.
6. Error Handling and Stream Events
6.1. Listening for Events
Streams emit various events that you can listen for, such as ‘data’, ‘end’, ‘error’, and more. These events help you monitor the stream’s progress and handle different scenarios appropriately.
6.2. Handling Errors in Streams
When working with streams, it’s crucial to handle errors to prevent your application from crashing. Use the ‘error’ event to catch and handle errors gracefully.
7. Implementing a Custom Transform Stream
7.1. Extending the Transform Class
You can create custom transform streams by extending the Transform class from the stream module. This allows you to define your data transformation logic.
7.2. Overriding the Transform Method
When implementing a custom transform stream, you need to override the transform method. This method is called for each chunk of data passing through the stream, allowing you to modify the data as needed.
8. Tips for Efficient Stream Usage
8.1. Setting HighWaterMark
The highWaterMark option specifies the maximum amount of data that can be buffered by a stream at once. Adjusting this value can optimize memory usage and improve performance.
8.2. Utilizing the Stream Module
Node.js provides the stream module with various classes and functions for working with streams. Familiarize yourself with this module to harness the full power of streams in your applications.
Conclusion
In conclusion, understanding streams in Node.js is essential for developing efficient and responsive applications that handle data gracefully. By grasping the concepts of readable, writable, duplex, and transform streams, you unlock the potential for efficient data processing, real-time applications, and seamless file operations. With the knowledge shared in this guide and the examples provided, you’re well-equipped to embark on your journey of mastering streams in Node.js. Happy streaming!
Table of Contents
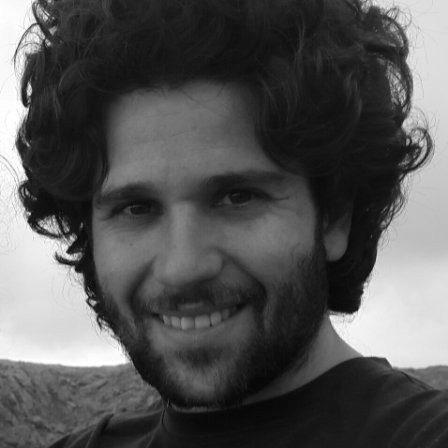
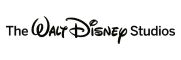