Testing Asynchronous Code in Node.js with Jest
In the world of Node.js development, writing tests for asynchronous code is a crucial aspect of ensuring the reliability and stability of your applications. Asynchronous operations are common in Node.js applications, such as making HTTP requests, querying databases, or reading files. Testing these operations effectively requires the right tools and techniques. In this guide, we’ll explore how to test asynchronous code in Node.js using Jest, a popular JavaScript testing framework.
Understanding Asynchronous Code Testing
Before diving into testing asynchronous code, let’s understand why it’s essential. Asynchronous code executes non-blocking operations, meaning it doesn’t wait for one operation to complete before starting another. This can lead to unpredictable behavior if not tested thoroughly. Jest provides a robust testing framework that simplifies testing asynchronous code by offering built-in support for promises, async/await, and callbacks.
Getting Started with Jest
If you haven’t already, install Jest in your Node.js project using npm or yarn:
npm install --save-dev jest
Or
yarn add --dev jest
Once Jest is installed, create a test file with a .test.js extension, for example, example.test.js. Jest will automatically find and run files with this naming convention.
Testing Asynchronous Code with Jest
1. Using Async/Await
Async/await is a modern JavaScript feature that allows writing asynchronous code in a synchronous manner, making it easier to reason about. Jest seamlessly supports testing functions that use async/await. Here’s an example:
// asyncFunction.js async function fetchData() { const response = await fetch('https://api.example.com/data'); return response.json(); } module.exports = fetchData;
——————
// asyncFunction.test.js const fetchData = require('./asyncFunction'); test('fetchData returns the correct data', async () => { const data = await fetchData(); expect(data).toEqual({ message: 'Hello, World!' }); });
2. Using Promises
Jest also supports testing functions that return promises. You can use the resolves matcher to test that a promise resolves with the expected value. Here’s an example:
// promiseFunction.js function fetchData() { return new Promise((resolve) => { setTimeout(() => { resolve({ message: 'Hello, World!' }); }, 1000); }); } module.exports = fetchData;
—————————–
// promiseFunction.test.js const fetchData = require('./promiseFunction'); test('fetchData returns the correct data', () => { return expect(fetchData()).resolves.toEqual({ message: 'Hello, World!' }); });
3. Mocking Asynchronous Functions
Sometimes, you may need to mock asynchronous functions, such as API calls or database queries, to isolate the code under test. Jest provides powerful mocking capabilities to achieve this. Here’s an example of mocking an API call:
// apiFunction.js const axios = require('axios'); async function fetchData() { const response = await axios.get('https://api.example.com/data'); return response.data; } module.exports = fetchData;
————————–
// apiFunction.test.js const axios = require('axios'); const fetchData = require('./apiFunction'); jest.mock('axios'); test('fetchData returns the correct data', async () => { axios.get.mockResolvedValue({ data: { message: 'Hello, World!' } }); const data = await fetchData(); expect(data).toEqual({ message: 'Hello, World!' }); });
Conclusion
Testing asynchronous code in Node.js with Jest is essential for building reliable and maintainable applications. By leveraging Jest’s built-in support for async/await, promises, and mocking, you can write comprehensive tests that ensure the correctness of your asynchronous code.
Start incorporating these testing techniques into your Node.js projects today to improve code quality and developer confidence.
External Links for Further Reading:
Table of Contents
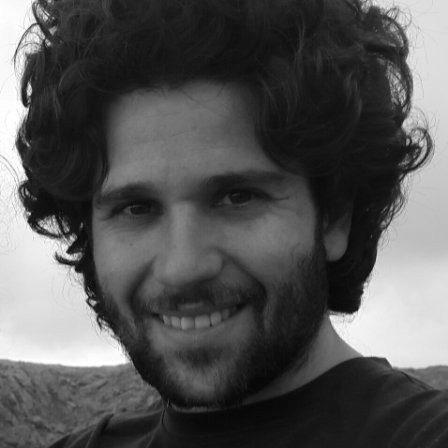
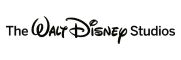