Node.js Q & A
What is the purpose of the timers module in Node.js?
The timers module in Node.js provides utilities for scheduling and executing asynchronous operations after a specified delay or at regular intervals. It allows developers to create timers, schedule callbacks, and perform time-based operations in Node.js applications.
Key functionalities of the timers module include:
- setTimeout():
-
-
- The setTimeout() function schedules a callback function to be executed after a specified delay (in milliseconds). It returns a timer object that can be used to cancel the scheduled operation using clearTimeout().
- Example:
- javascript
-
- Copy code
setTimeout(() => { console.log('Delayed operation'); }, 1000); // Execute after 1 second
- setInterval():
-
- The setInterval() function schedules a callback function to be executed repeatedly at fixed intervals (in milliseconds). It returns a timer object that can be used to cancel the interval-based operation using clearInterval().
- Example:
- javascript
- Copy code
setInterval(() => { console.log('Repeated operation'); }, 1000); // Execute every 1 secon
- Clearing Timers:
-
-
- Use the clearTimeout() and clearInterval() functions to cancel scheduled timeouts and intervals, respectively. Pass the timer object returned by setTimeout() or setInterval() as an argument to clear the corresponding timer.
- Example:
- javascript
-
- Copy code
const timer = setTimeout(() => { console.log('Delayed operation'); }, 1000); clearTimeout(timer); // Cancel the scheduled timeout
- Immediate Execution:
-
-
- The setImmediate() function schedules a callback function to be executed immediately after the current event loop iteration completes. It ensures that the callback is executed asynchronously without blocking the event loop.
- Example:
- javascript
-
- Copy code
setImmediate(() => { console.log('Immediate operation'); });
- Process Next Tick:
-
-
- The process.nextTick() function schedules a callback function to be executed in the next iteration of the event loop, immediately after the current operation completes. It allows developers to defer execution of callback functions to avoid blocking I/O operations.
- Example:
- javascript
-
- Copy code
process.nextTick(() => { console.log('Next tick operation'); });
- Performance Optimization:
-
-
- Use timers and asynchronous operations judiciously to optimize performance and resource utilization in Node.js applications. Avoid blocking the event loop with long-running synchronous operations and leverage timers for non-blocking asynchronous tasks.
-
- Error Handling:
-
-
- Implement error handling mechanisms to catch and handle errors in timer callbacks. Use try-catch blocks or error callback functions to handle exceptions and prevent uncaught exceptions from crashing the application.
-
- Testing and Debugging:
-
- Test timer-based functionality thoroughly using unit tests, integration tests, and end-to-end tests. Debug timer-related issues using debugging tools, logging, and error tracking to identify and fix issues efficiently.
By leveraging the timers module in Node.js, developers can implement time-based operations, schedule asynchronous tasks, and optimize performance effectively, enhancing the responsiveness and reliability of Node.js applications.
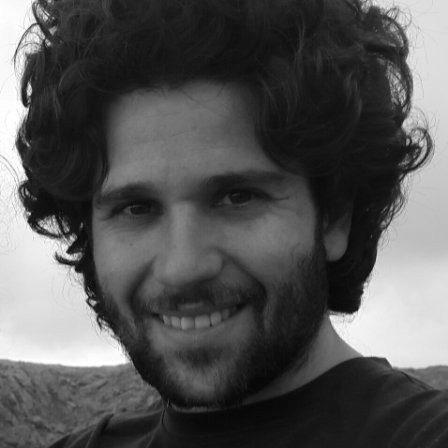
Previously at
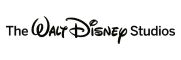
Experienced Principal Engineer and Fullstack Developer with a strong focus on Node.js. Over 5 years of Node.js development experience.