What is the purpose of the URL module in Node.js?
The URL module in Node.js provides utilities for parsing, formatting, and resolving URL strings, allowing developers to work with URLs more effectively in Node.js applications. It simplifies tasks such as URL parsing, manipulation, and validation, enabling developers to extract relevant information from URLs and construct URLs dynamically.
Key functionalities of the URL module include:
- URL Parsing:
-
- Use the URL class to parse URL strings into URL objects, representing different components of the URL such as protocol, hostname, port, path, query parameters, and fragment identifier.
- Example:
- javascript
- Copy code
const { URL } = require('url'); const urlString = 'https://www.example.com/path?query=param#fragment'; const parsedUrl = new URL(urlString); console.log(parsedUrl.protocol); // Output: 'https:' console.log(parsedUrl.hostname); // Output: 'www.example.com' console.log(parsedUrl.pathname); // Output: '/path' console.log(parsedUrl.searchParams.get('query')); // Output: 'param' console.log(parsedUrl.hash); // Output: '#fragment'
- URL Formatting:
-
- Use URL objects to format URL strings from URL components or modify existing URLs by setting individual components such as protocol, hostname, port, path, query parameters, and fragment identifier.
- Example:
- javascript
- Copy code
const { URL } = require('url'); const urlObject = new URL('https://www.example.com'); urlObject.pathname = '/new-path'; urlObject.searchParams.set('param', 'value'); console.log(urlObject.toString()); // Output: 'https://www.example.com/new-path?param=value'
- URL Resolving:
-
- Use the url.resolve() function to resolve relative URLs against a base URL, generating an absolute URL. This is useful for constructing absolute URLs from relative paths or resolving URLs dynamically based on context.
- Example:
- javascript
- Copy code
const { resolve } = require('url'); const baseUrl = 'https://www.example.com'; const relativeUrl = '/path'; const absoluteUrl = resolve(baseUrl, relativeUrl); console.log(absoluteUrl); // Output: 'https://www.example.com/path'
- URL Validation:
-
-
- Validate URL strings or URL components using built-in validation functions provided by the URL module. Validate URL syntax, protocol, hostname, and other components to ensure URL integrity and security.
-
- URL Serialization:
-
-
- Serialize URL objects into URL strings using the url.format() function. This allows developers to convert URL objects back into URL strings after manipulation or processing.
- Example:
- javascript
-
- Copy code
const { format } = require('url'); const urlObject = { protocol: 'https:', hostname: 'www.example.com', pathname: '/path', search: '?query=param', hash: '#fragment' }; console.log(format(urlObject)); // Output: 'https://www.example.com/path?query=param#fragment'
The URL module simplifies URL handling and manipulation in Node.js applications by providing a standardized interface for working with URLs. It ensures consistency, accuracy, and security when dealing with URL-related operations, facilitating URL parsing, formatting, and resolution tasks efficiently.
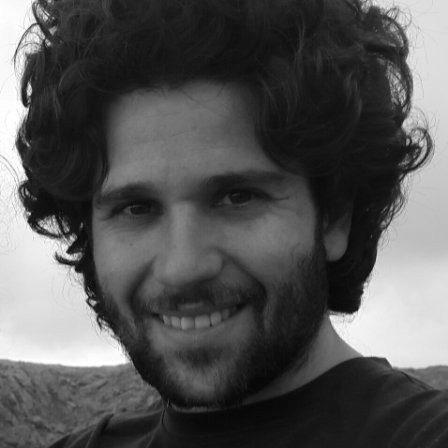
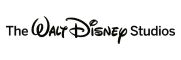