Building Web APIs with Node.js and Fastify
Node.js has long been a popular choice for building web APIs due to its non-blocking architecture and vast ecosystem. Fastify, a modern framework for Node.js, further enhances API development by providing an ultra-fast, low-overhead solution. This blog explores how to use Node.js with Fastify to build efficient and scalable web APIs, including practical examples and best practices.
Understanding Fastify
Fastify is a web framework designed for building high-performance applications. It provides a straightforward and extensible API, optimized for speed and low overhead. With built-in support for JSON schema validation and async/await, Fastify simplifies the process of creating robust and scalable APIs.
Getting Started with Fastify
To start building APIs with Fastify, you’ll first need to set up your project. Here’s a step-by-step guide to get you going.
1. Setting Up the Project
Begin by initializing a new Node.js project and installing Fastify.
```bash mkdir fastify-api cd fastify-api npm init -y npm install fastify ```
2. Creating a Basic Fastify Server
Here’s how to create a simple Fastify server that responds with a “Hello World” message.
```javascript // index.js const Fastify = require('fastify'); const fastify = Fastify({ logger: true }); fastify.get('/', async (request, reply) => { return { message: 'Hello World' }; }); fastify.listen(3000, err => { if (err) { fastify.log.error(err); process.exit(1); } fastify.log.info('Server listening on http://localhost:3000'); }); ```
Run the server with `node index.js` and navigate to `http://localhost:3000` to see the response.
3. Defining Routes
Fastify allows you to define routes with ease. Below is an example of defining multiple routes, including a parameterized route.
```javascript // routes.js const routes = (fastify, options, done) => { fastify.get('/users', async (request, reply) => { return [{ id: 1, name: 'John Doe' }]; }); fastify.get('/users/:id', async (request, reply) => { const userId = request.params.id; return { id: userId, name: 'John Doe' }; }); done(); }; module.exports = routes; ```
Integrate this route file with your server.
```javascript // index.js const Fastify = require('fastify'); const fastify = Fastify({ logger: true }); const routes = require('./routes'); fastify.register(routes); fastify.listen(3000, err => { if (err) { fastify.log.error(err); process.exit(1); } fastify.log.info('Server listening on http://localhost:3000'); }); ```
4. Handling Requests and Validations
Fastify uses JSON schema for request validation, making it easy to ensure data integrity. Here’s how to add validation to your routes.
```javascript // routes.js const routes = (fastify, options, done) => { fastify.post('/users', { schema: { body: { type: 'object', required: ['name'], properties: { name: { type: 'string' } } } } }, async (request, reply) => { const { name } = request.body; return { id: 2, name }; }); done(); }; module.exports = routes; ```
5. Error Handling
Fastify provides mechanisms for handling errors gracefully. Here’s an example of custom error handling.
```javascript // index.js const Fastify = require('fastify'); const fastify = Fastify({ logger: true }); fastify.setErrorHandler((error, request, reply) => { request.log.error(error); reply.status(500).send({ error: 'Internal Server Error' }); }); fastify.get('/', async (request, reply) => { throw new Error('Something went wrong'); }); fastify.listen(3000, err => { if (err) { fastify.log.error(err); process.exit(1); } fastify.log.info('Server listening on http://localhost:3000'); }); ```
6. Testing Fastify Applications
Fastify supports testing with a variety of tools. Here’s a basic example using `tap`.
```bash npm install tap fastify-tap ``` ```javascript // test.js const Fastify = require('fastify'); const test = require('tap').test; test('GET `/` route', async t => { const fastify = Fastify(); fastify.get('/', async (request, reply) => { return { message: 'Hello World' }; }); await fastify.ready(); const response = await fastify.inject({ method: 'GET', url: '/' }); t.equal(response.statusCode, 200); t.same(JSON.parse(response.payload), { message: 'Hello World' }); }); ```
Conclusion
Fastify, combined with Node.js, offers a powerful and efficient framework for building scalable web APIs. Its ease of use, performance benefits, and built-in features make it a great choice for developers looking to create high-performance applications. By leveraging Fastify’s capabilities, you can build robust and reliable APIs that meet modern performance and scalability requirements.
Further Reading
Table of Contents
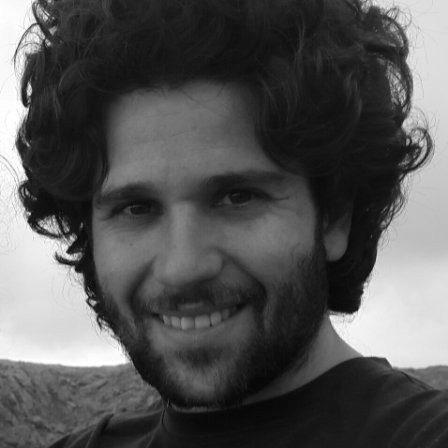
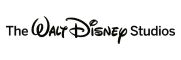