Web Scraping with Node.js: Techniques and Tools
In today’s data-driven world, information is a valuable asset. Whether you’re a developer, researcher, or business professional, accessing and extracting data from the web can provide you with insights, trends, and competitive advantages. Web scraping, the process of automating the extraction of data from websites, has become an indispensable skill in various fields. In this blog post, we’ll delve into the world of web scraping using Node.js, a powerful JavaScript runtime. We’ll explore various techniques, tools, and provide you with practical code samples to get you started on your web scraping journey.
Table of Contents
1. Introduction to Web Scraping
1.1. What is Web Scraping?
Web scraping, also known as web harvesting or web data extraction, involves the automated extraction of data from websites. This process involves sending HTTP requests to websites, retrieving HTML content, and parsing it to extract the desired information. Web scraping allows you to gather data for analysis, research, competitive intelligence, and more.
1.2. Legality and Ethics
While web scraping offers numerous benefits, it’s crucial to be aware of the legal and ethical considerations. Some websites prohibit scraping in their terms of service, and scraping too aggressively can potentially overload servers and impact the website’s performance. Always review a website’s terms of use, respect the robots.txt file that specifies which parts of a website can be scraped, and avoid overloading servers with excessive requests.
2. Getting Started with Node.js
2.1. Installing Node.js
Before we dive into web scraping, ensure you have Node.js installed on your system. You can download it from the official Node.js website (https://nodejs.org/) and follow the installation instructions for your operating system.
2.2. Basic Node.js Concepts
Node.js is a JavaScript runtime that allows you to execute JavaScript code on the server-side. It uses an event-driven, non-blocking I/O model, making it suitable for asynchronous operations like web scraping. Familiarize yourself with basic Node.js concepts, such as modules, npm (Node Package Manager), and asynchronous programming, to make the most of your scraping projects.
3. Essential Tools for Web Scraping with Node.js
3.1. Axios
Axios is a popular HTTP client for Node.js that simplifies making HTTP requests. It supports promises and async/await, making it ideal for web scraping tasks. Here’s a simple example of using Axios to fetch a web page’s HTML content:
javascript const axios = require('axios'); async function fetchWebPage(url) { try { const response = await axios.get(url); const html = response.data; return html; } catch (error) { console.error('Error fetching web page:', error); } } const webpageUrl = 'https://example.com'; fetchWebPage(webpageUrl) .then(html => { console.log(html); });
3.2. Cheerio
Cheerio is a fast and flexible library that enables you to parse and manipulate HTML content using a jQuery-like syntax. It’s particularly useful for scraping static websites. Here’s a basic example of using Cheerio to extract information from an HTML page:
javascript const cheerio = require('cheerio'); const html = '<div><h1>Hello, World!</h1></div>'; const $ = cheerio.load(html); const text = $('h1').text(); console.log(text); // Output: Hello, World!
3.3. Puppeteer
Puppeteer is a headless browser automation library that provides a full browser environment for web scraping. It’s excellent for scraping websites with dynamic content rendered using JavaScript. Puppeteer allows you to interact with pages, fill out forms, take screenshots, and more. Here’s a simple example of using Puppeteer to take a screenshot of a webpage:
javascript const puppeteer = require('puppeteer'); (async () => { const browser = await puppeteer.launch(); const page = await browser.newPage(); await page.goto('https://example.com'); await page.screenshot({ path: 'screenshot.png' }); await browser.close(); })();
4. Web Scraping Techniques
4.1. Scraping Static Websites
Static websites have HTML content that doesn’t change frequently. To scrape data from static sites, use libraries like Axios and Cheerio. Identify the HTML structure and use selectors to extract the desired information.
javascript // Example: Scraping quotes from a static website const axios = require('axios'); const cheerio = require('cheerio'); async function scrapeQuotes() { const url = 'https://example-quotes.com'; const response = await axios.get(url); const html = response.data; const $ = cheerio.load(html); const quotes = []; $('blockquote.quote').each((index, element) => { const text = $(element).find('p').text(); quotes.push(text); }); return quotes; } scrapeQuotes().then(quotes => { console.log(quotes); });
4.2. Handling Dynamic Content
Dynamic websites generate content using JavaScript, which requires a headless browser like Puppeteer to render and scrape the data. Use Puppeteer to interact with dynamic elements, wait for AJAX requests to complete, and access the fully rendered content.
javascript // Example: Scraping product details from a dynamic website const puppeteer = require('puppeteer'); async function scrapeProducts() { const browser = await puppeteer.launch(); const page = await browser.newPage(); await page.goto('https://example-products.com'); await page.waitForSelector('.product-list'); const products = await page.evaluate(() => { const productElements = document.querySelectorAll('.product'); const productData = []; productElements.forEach(element => { const name = element.querySelector('.name').textContent; const price = element.querySelector('.price').textContent; productData.push({ name, price }); }); return productData; }); await browser.close(); return products; } scrapeProducts().then(products => { console.log(products); });
4.3. Pagination and Iteration
When scraping multiple pages, handle pagination by iterating through pages using a loop or recursion. Adjust the URL parameters to navigate through different pages and collect the required data.
javascript // Example: Scraping news articles from paginated website const axios = require('axios'); const cheerio = require('cheerio'); async function scrapeNewsArticles() { const baseUrl = 'https://example-news.com'; const articles = []; for (let page = 1; page <= 5; page++) { const url = `${baseUrl}/page/${page}`; const response = await axios.get(url); const html = response.data; const $ = cheerio.load(html); $('.article').each((index, element) => { const title = $(element).find('.title').text(); articles.push(title); }); } return articles; } scrapeNewsArticles().then(articles => { console.log(articles); });
4.4. Dealing with Asynchronous Requests
Web scraping often involves asynchronous tasks like making multiple requests concurrently. Use asynchronous programming techniques like Promises, async/await, or libraries like Promise.all() to handle parallel requests efficiently.
javascript // Example: Scraping data from multiple URLs concurrently const axios = require('axios'); async function scrapeMultipleUrls(urls) { const promises = urls.map(url => axios.get(url)); const responses = await Promise.all(promises); const data = responses.map(response => response.data); return data; } const websiteUrls = ['https://example1.com', 'https://example2.com', 'https://example3.com']; scrapeMultipleUrls(websiteUrls).then(data => { console.log(data); });
5. Best Practices
5.1. Respect Robots.txt
The robots.txt file is a standard used by websites to communicate with web crawlers and scrapers. Always check the robots.txt file of a website to understand which parts are open for scraping and which are off-limits.
5.2. Use Request Delays
To avoid overloading websites and potentially getting blocked, incorporate delays between your requests. Respect the website’s bandwidth and response times to ensure a smoother scraping experience.
5.3. Error Handling and Retry Strategies
Websites may encounter errors or timeouts during scraping due to various reasons. Implement error handling and retry mechanisms to gracefully handle such situations and improve the overall reliability of your scraper.
5.4. Monitoring and Maintenance
Websites frequently update their structure and content, which can break your scrapers. Regularly monitor your scrapers for errors and adapt them to changes in the target websites. Consider setting up alerts to notify you of any issues.
6. Putting It All Together
6.1. Building a Simple Web Scraper
Let’s put our knowledge into action and build a simple web scraper using Node.js and Cheerio. In this example, we’ll scrape book titles and prices from an online bookstore.
javascript const axios = require('axios'); const cheerio = require('cheerio'); async function scrapeBookstore() { const url = 'https://example-bookstore.com'; const response = await axios.get(url); const html = response.data; const $ = cheerio.load(html); const books = []; $('.book').each((index, element) => { const title = $(element).find('.title').text(); const price = $(element).find('.price').text(); books.push({ title, price }); }); return books; } scrapeBookstore().then(books => { console.log(books); });
6.2. Advanced Scraping Examples
For more complex scenarios, consider scraping data from social media platforms, job boards, real estate listings, and more. Use the techniques and tools mentioned earlier to adapt to different website structures and data sources.
Conclusion
Web scraping with Node.js opens up a world of possibilities for gathering data from the internet. Armed with the right tools and techniques, you can extract valuable insights, automate repetitive tasks, and stay ahead in various domains. Remember to always respect website terms, employ ethical practices, and be mindful of server load. As you dive into the world of web scraping, you’ll uncover new ways to leverage data for your projects, research, and decision-making processes. Happy scraping!
Table of Contents
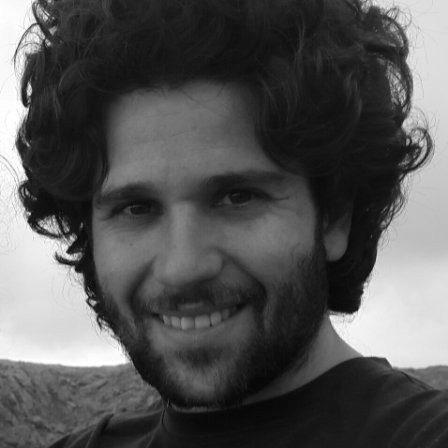
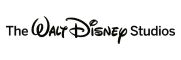